How to Convert String to Float or Int in Python
-
float()
to Convert String to Float in Python -
int()
to Convert String to Int in Python -
ast.literal_eval
to Convert String to Float or Int in Python - Localization and Commas in Converting String to Float in Python
- Comparison of Performances of Different Methods to Convert String to Float or Int in Python
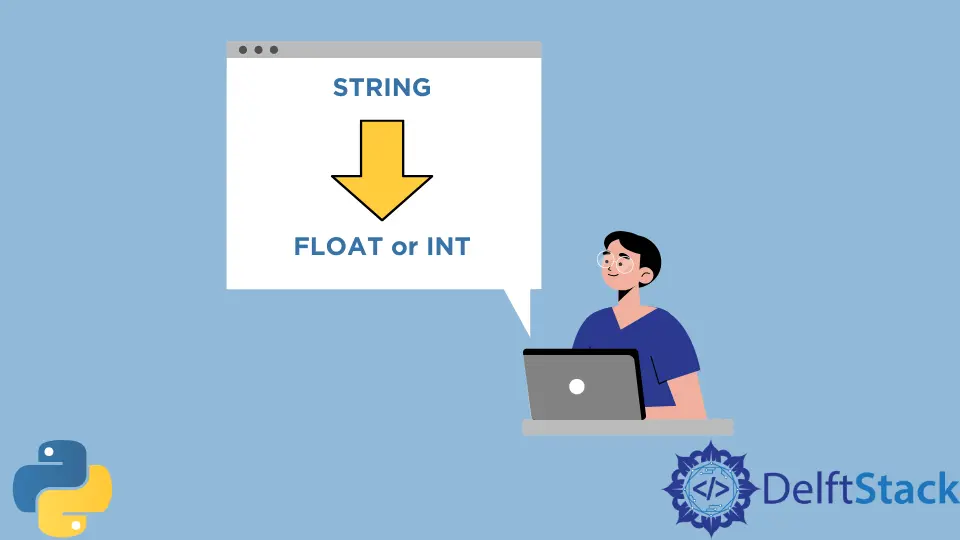
This tutorial article will introduce how to convert string to float or int in Python, with the Python built-in float()
and int()
functions.
float()
to Convert String to Float in Python
float()
converts the string to the float
pointing number, if possible.
>>> float('111.2222')
111.2222
>>> float('111.0')
111.0
>>> float('111')
111.0
int()
to Convert String to Int in Python
int()
could convert the string to an integer if the given string represents an integer. Otherwise, it will raise ValueError
.
>>> int('111')
111
>>> int('111.0')
Traceback (most recent call last):
File "<pyshell#43>", line 1, in <module>
int('111.0')
ValueError: invalid literal for int() with base 10: '111.0'
>>> int('111.22222')
Traceback (most recent call last):
File "<pyshell#45>", line 1, in <module>
int('111.22222')
ValueError: invalid literal for int() with base 10: '111.22222'
You could convert the string to float
first and then cast it to int
if the given string represents a floating pointing number.
>>> int(float('111.0'))
111
ast.literal_eval
to Convert String to Float or Int in Python
ast.literal_eval(string)
safely evaluate the given string containing a Python expression. It could convert the string to either float
or int
automatically.
>>> ast.literal_eval('111.2222')
111.2222
>>> ast.literal_eval('111.0')
111.0
>>> ast.literal_eval('111')
111
Localization and Commas in Converting String to Float in Python
If the comma ,
exists in the string representation, then the float
conversion will throw a ValueError
exception. But commas are regularly used as either a thousand separator in countries like the US or UK, for example, 111,111.22
, or decimal mark in most European countries, for example, 111,222
.
>>> float('111,111.22')
Traceback (most recent call last):
File "<pyshell#54>", line 1, in <module>
float('111,111.22')
ValueError: could not convert string to float: '111,111.22'
>>> float('111,111')
Traceback (most recent call last):
File "<pyshell#55>", line 1, in <module>
float('111,111')
ValueError: could not convert string to float: '111,111'
The locale
module should be used to assign the locale information, and then the locale.atof()
function could parse the given string as a float according to the locale settings.
Commas as Thousand Seperator in US or UK
>>> import locale
>>> A = '111,111.222'
>>> locale.setlocale(locale.LC_ALL, 'en_US.UTF-8')
'en_US.UTF-8'
>>> locale.atof(A)
111111.222
>>> locale.atof('111,111')
111111.0
The comma ,
could be interpreted correctly if the right locale setting is given.
Be aware that the conversion result is always a float
type, even if the given string representation is an integer.
Commas as Demical Mark in European Countries
>>> import locale
>>> locale.setlocale(locale.LC_ALL, 'nl_NL')
'nl_NL'
>>> locale.atof('111,222')
111.222
>>> locale.atof('111.111,222')
111111.222
111,222
is converted to 111.222
properly, and 111.111,222
could also be appropriately converted to 111111.222
as the point .
is used as the thousand separator in European countries like the Netherlands.
Comparison of Performances of Different Methods to Convert String to Float or Int in Python
We use the timeit
to check the efficiency performances between different methods.
>>> import timeint
>>> timeit.timeit('float(111.2222)', number=1000000)
0.14707240000007005
>>> timeit.timeit('ast.literal_eval("111.2222")', setup='import ast', number=1000000)
4.779956100000163
>>> timeit.timeit('locale.atof("111.2222")', setup='import locale; locale.setlocale(locale.LC_ALL, "en_US.UTF-8")', number=1000000)
6.092166299999917
ast.literal_eval()
method is tremendously slower than float
method as shown above. It should not be the first choice if you simply need to convert string to float or int in Python. ast.literal_eval()
is over-engineered in this application as its main target should be evaluating the string containing Python expression.
locale.atof()
is also extremely slow; therefore, it should only be used when commas exist in the string for the convention in US or UK, or point .
means the thousand separator but comma ,
means the decimal mark in European countries. In other words, you should use locale.atof()
only if it is really necessary.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook