How to Convert Bytes to Int in Python 2.7 and 3.x
- Python 2.7 Bytes Data Type
- Convert Byte to Int in Python 2.7
- Python 3 Bytes Data Type
- Convert Bytes to Int in Python 3
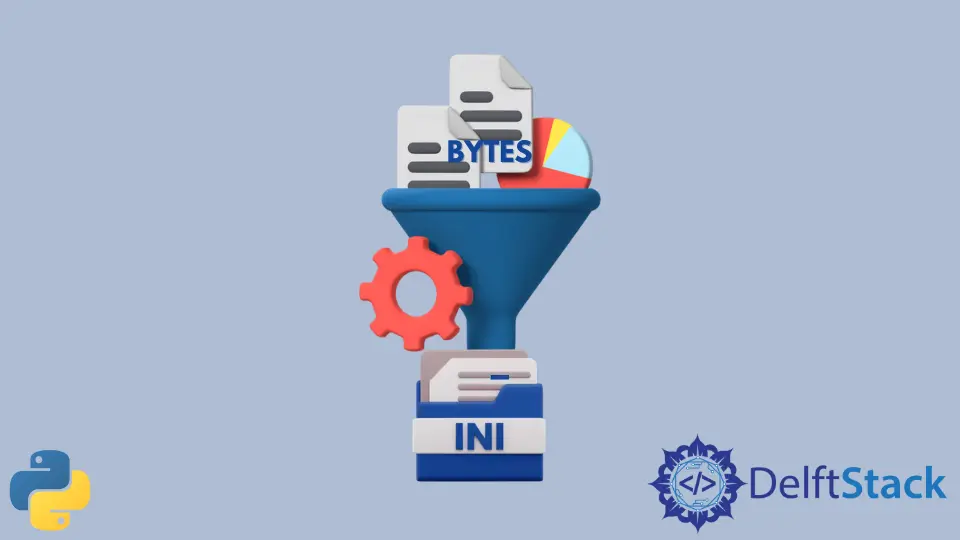
Bytes
data type has the value with a range from 0 to 255 (0x00 to 0xFF). One byte has 8 bits; that’s why its maximum value is 0xFF. In some circumstances, you need to convert bytes or bytes array to integers for further data processing. This short article introduces methods to convert byte to int in Python, like the struct.unpack
method in Python 2.7 and int.from_bytes()
in Python 3.x.
Python 2.7 Bytes Data Type
There is no built-in bytes
data type in Python 2.7 version. Keyword byte
is identical to str
.
>>> bytes is str
True
bytearray
is used to define a bytes
or byte array
object.
>>> byteExample1 = bytearray([1])
>>> byteExample1
bytearray(b'\x01')
>>> byteExample2 = bytearray([1,2,3])
>>> byteExample2
bytearray(b'\x01\x02\x03')
Convert Byte to Int in Python 2.7
Python internal module struct
could convert binary data (bytes) to integers. It could convert bytes or actually strings in Python 2.7 and integers in a bidirectional way.
struct.unpack(fmt, string)
Convert the string according to the given format `fmt` to integers. The result is a tuple even if there is only one item inside.
struct
Examples: Convert Byte to Int in Python 2.7
# python 2.x
import struct
testBytes = b"\x00\x01\x00\x02"
testResult = struct.unpack(">HH", testBytes)
print testResult
Output:
(1, 2)
The format string >HH
contains two parts.
>
indicates the binary data isbig-endian
, or in other words, the data is ordered from the big end (most significant bit). For example,\x00\0x1
means\x00
is the high byte, and\x01
is the low byte.HH
means there are two objects ofH
types in the bytes string.H
represents anunsigned short
integer that takes 2 bytes.
You could get different results from the same string if the assigned data format is different.
>>> testResult = struct.unpack('<HH', testBytes)
>>> testResult
(256, 512)
Here, <
indicates the endianess is little-endian
. Therefore \x00\x01
becomes 00+1*256 = 256
, not 0*256+1 = 1
anymore.
>>> testResult = struct.unpack('<BBBB', testBytes)
>>> testResult
(0, 1, 0, 2)
B
means the data is unsigned char
taking 1 byte. Hence, \x00\x01\x00\x02
will be converted to 4 values of unsigned char
, but not 2 values of unsigned short
anymore.
>>> testResult = struct.unpack('<BBB', b'\x00\x01\x00\x02')
Traceback (most recent call last):
File "<pyshell#35>", line 1, in <module>
testResult = struct.unpack('<BBB', b'\x00\x01\x00\x02')
error: unpack requires a string argument of length 3
You could check the struct
module’s official document to get more information on format strings.
Python 3 Bytes Data Type
bytes
is a built-in data type in Python 3; therefore, you could define bytes directly using the bytes
keyword.
>>> testByte = bytes(18)
>>> type(testByte)
<class 'bytes'>
You could also directly define a bytes or bytes array like below.
>>> testBytes = b'\x01\x21\31\41'
>>> type(testBytes)
<class 'bytes'>
Convert Bytes to Int in Python 3
Besides the struct
module as already introduced in Python 2.7, you could also use a new Python 3 built-in int
method to do the bytes-to-integers conversions, that is, the int.from_bytes()
method.
int.from_bytes()
Examples: Convert Byte to Int
>>> testBytes = b'\xF1\x10'
>>> int.from_bytes(testBytes, byteorder='big')
61712
The byteorder
option is similar to struct.unpack()
format byte order definition.
int.from_bytes()
has a third option signed
to assign the integer type to be signed
or unsigned
.
>>> testBytes = b'\xF1\x10'
>>> int.from_bytes(testBytes, byteorder='big', signed=True)
-3824
Use []
When Bytes Is unsigned char
If the format of data has the format of unsigned char
containing only one byte, you could directly use object index to access and get the integer of the data.
>>> testBytes = b'\xF1\x10'
>>> testBytes[0]
241
>>> testBytes[1]
16
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook