Python 中如何將位元組 bytes 轉換為整數 int
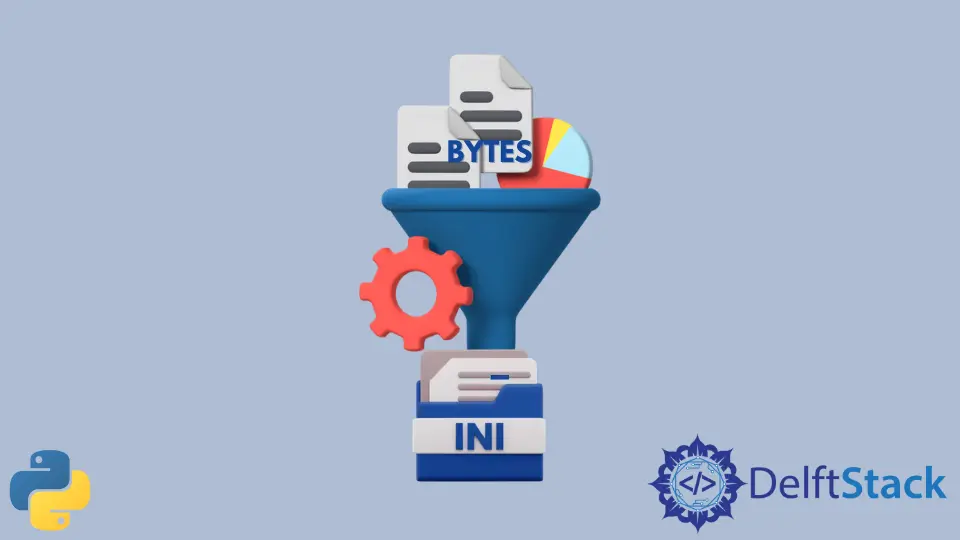
Bytes
資料型別的數值範圍為 0~255(0x00~0xFF)。一個位元組有 8 位資料,這就是為什麼它的最大值是 0xFF。在某些情況下,你需要將位元組或位元組陣列轉換為整數以進行進一步的資料處理。我們就來看如何進行位元組 Bytes
到整數 integer
的轉換。
Python 2.7 中的位元組 Bytes
Python 2.7 版本中沒有內建的 bytes
資料型別。關鍵字 byte
與 str
是等同的。我們可以通過下面的 is
判斷的高處這個結論。
>>> bytes is str
True
bytearray
用於定義一個 bytes
或一個 byte array
物件。
>>> byteExample1 = bytearray([1])
>>> byteExample1
bytearray(b'\x01')
>>> byteExample2 = bytearray([1,2,3])
>>> byteExample2
bytearray(b'\x01\x02\x03')
Python 2.7 中將位元組轉換為整數
Python 內部模組 struct
可以將二進位制資料(位元組)轉換為整數。它可以雙向轉換 Python 2.7 中的位元組(實際上是字串)和整數。下面就是它的一個基本介紹,
struct.unpack(fmt, string)
# Convert the string according to the given format `fmt` to integers. The result is a tuple even if there is only one item inside.
struct
例子
import struct
testBytes = b"\x00\x01\x00\x02"
testResult = struct.unpack(">HH", testBytes)
print testResult
(1, 2)
格式字串 >HH
包含兩部分,
>
表示二進位制資料是big-endian
,或換句話說,資料是從高階(最高有效位)排序的。例如,\x00\0x1
中,\x00
是高位元組,\x01
是低位元組。HH
表示位元組字串中有兩個H
型別的物件。H
表示它是unsigned short
整數,佔用了 2 個位元組。
如果分配的資料格式不同,你可以從相同的字串中得到不同的結果。
>>> testResult = struct.unpack('<HH', testBytes)
>>> testResult
(256, 512)
這裡,<
表示位元組順序是 little-endian
。因此\x00\x01
變成 00+1*256 = 256
不是 0*256+1 = 1
了。
>>> testResult = struct.unpack('<BBBB', testBytes)
>>> testResult
(0, 1, 0, 2)
B
表示資料型別是 unsigned char
,它佔用了 1 個位元組。因此,\x00\x01\x00\x02
將被轉換為 4 個 unsigned char
的值,而不再是 2 個 unsigned short
的值。
>>> testResult = struct.unpack('<BBB', b'\x00\x01\x00\x02')
Traceback (most recent call last):
File "<pyshell#35>", line 1, in <module>
testResult = struct.unpack('<BBB', b'\x00\x01\x00\x02')
error: unpack requires a string argument of length 3
你可以檢視 struct
模組的官方文件來獲取格式字串的更多資訊。
Python 3 中的位元組 Bytes
bytes
是 Python 3 中的內建資料型別,因此,你可以使用 bytes
關鍵字直接來定義位元組。
>>> testByte = bytes(18)
>>> type(testByte)
<class 'bytes'>
你也可以像下面這樣直接定義一個或多個位元組。
>>> testBytes = b'\x01\x21\31\41'
>>> type(testBytes)
<class 'bytes'>
Python 3 中將位元組轉換為整數
除了已經在 Python 2.7 中引入的 struct
模組之外,你還可以使用新的 Python 3 內建整數方法來執行位元組到整數的轉換,即 int.from_bytes()
方法。
int.from_bytes()
例子
>>> testBytes = b'\xF1\x10'
>>> int.from_bytes(testBytes, byteorder='big')
61712
該 byteorder
選項類似於 struct.unpack()
位元組順序格式的定義。
The byteorder
option is similar to struct.unpack()
format byte order definition.
int.from_bytes()
有第三個選項 signed
,它將整數型別賦值為 signed
或 unsigned
。
>>> testBytes = b'\xF1\x10'
>>> int.from_bytes(testBytes, byteorder='big', signed=True)
-3824
當位元組是 unsigned chart
時使用 []
如果資料格式是 unsigned char
且只包含一個位元組,則可以直接使用物件索引進行訪問來獲取該整數。
>>> testBytes = b'\xF1\x10'
>>> testBytes[0]
241
>>> testBytes[1]
16