How to Check the Python Version in the Scripts
-
sys.version
Method to Check Python Version -
sys.version_info
Method to Check Python Version -
platform.python_version()
Method to Check Python Version -
six
Module Method to Check Python Version
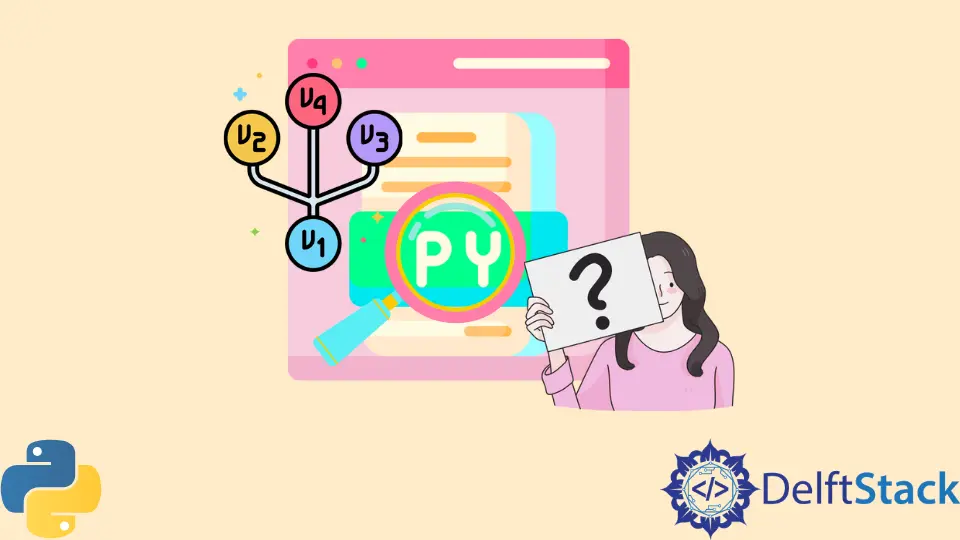
In different circumstances, we need to know the Python version, or more precisely, the Python interpreter version that is executing the Python script file.
sys.version
Method to Check Python Version
This version information could be retrieved from sys.version
in the sys
module.
In Python 2.x
>>> import sys
>>> sys.version
'2.7.10 (default, May 23 2015, 09:44:00) [MSC v.1500 64 bit (AMD64)]'
or in Python 3.x
>>> import sys
>>> sys.version
'3.7.0 (v3.7.0:1bf9cc5093, Jun 27 2018, 04:59:51) [MSC v.1914 64 bit (AMD64)]'
sys.version_info
Method to Check Python Version
sys.version
returns a string containing the human-readable version information of the current Python interpreter. But the information like major release number and micro release number needs extra processing to be derived for further usage in the codes.
sys.version_info
solves this problem easily by returning the version information as a named tuple. The version data it returns is,
Data | Description |
---|---|
major |
Major release number |
micro |
Patch release number |
minor |
Minor release number |
releaselevel |
alpha , beta , candidate or release |
serial |
Serial release number |
>>> import sys
>>> sys.version_info
sys.version_info(major=2, minor=7, micro=10, releaselevel='final', serial=0)
You could compare the current version with the reference version simply using >
, >=
, ==
, <=
or <
operators.
>>> import sys
>>> sys.version_info >= (2, 7)
True
>>> sys.version_info >= (2, 7, 11)
False
We could add an assert
in the scripts to make sure that the script runs with the requirement of minimal Python version.
import sys
assert sys.version_info >= (3, 7)
It will raise an AssertionError
if the interpreter doesn’t meet the version requirement.
Traceback (most recent call last):
File "C:\test\test.py", line 4, in <module>
assert sys.version_info >= (3, 7)
AssertionError
platform.python_version()
Method to Check Python Version
python_version()
in platform
module returns the Python version as string major.minor.patchlevel
.
>>> from platform import python_version
>>> python_version()
'3.7.0'
Or similar to sys.version_info
, platform
also has a method to return the Python version as tuple (major, minor, patchlevel)
of strings - python_version_tuple()
>>> import platform
>>> platform.python_version_tuple()
('3', '7', '0')
six
Module Method to Check Python Version
If you only need to check whether the Python version is Python 2.x or Python 3.x, you could use six
module to do the job.
from __future__ import print_function
import six
if six.PY2:
print("Python 2.x")
if six.PY3:
print ("Python 3.x")
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook