How to Compare Two Dictionaries in Python
Najwa Riyaz
Feb 02, 2024
Python
Python Dictionary
-
Use the
==
Operator to Compare Two Dictionaries in Python - Write Custom Code to Compare Two Dictionaries in Python
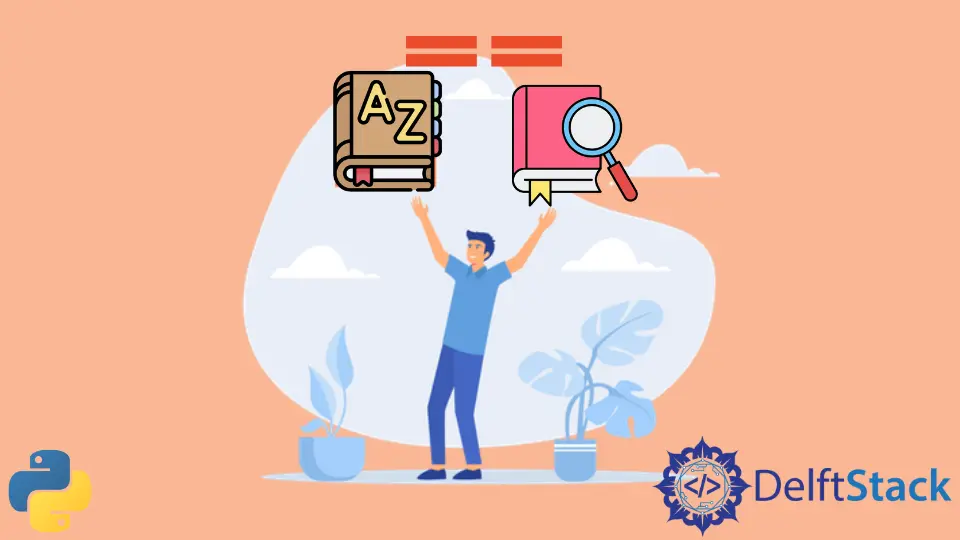
This article will introduce how to compare two dictionaries in Python.
Use the ==
Operator to Compare Two Dictionaries in Python
The ==
operator in Python can be used to determine if the dictionaries are identical or not.
Here is an example when there are identical dictionaries.
dict1 = dict(name="Tom", Vehicle="Benz Car")
dict2 = dict(name="Tom", Vehicle="Benz Car")
dict1 == dict2
Output:
True
Here is an example, when there are non-identical dictionaries -
dict1 = dict(name="John", Vehicle="Benz Car")
dict2 = dict(name="Tom", Vehicle="Benz Car")
dict1 == dict2
Output:
False
You can compare many dictionaries as mentioned in the following example,
dict1 = dict(name="John", Vehicle="Benz Car")
dict2 = dict(name="Tom", Vehicle="Benz Car")
dict3 = dict(name="Shona", Vehicle="Alto Car")
dict4 = dict(name="Ruby", Vehicle="Honda Car")
dict1 == dict2 == dict3 == dict4
Output:
False
Write Custom Code to Compare Two Dictionaries in Python
Here is how you can write code to compare the dictionaries and determine how many pairs are common between the dictionaries. Below are the steps.
-
Use a
for
loop to traverse through each item in one of the dictionaries. Compare each item of this dictionary with the other dictionary based on the shared index. -
If the items are equal, then place the
key:value
pair into the result shared dictionary. -
Once the whole dictionary is traversed, calculate the result shared dictionary’s length to determine the number of common items between the dictionaries.
Below is an example that demonstrates the method to compare two dictionaries in Python.
In this case the dictionaries are identical.
dict1 = dict(name="Tom", Vehicle="Mercedes Car")
dict2 = dict(name="Tom", Vehicle="Mercedes Car")
dict1_len = len(dict1)
dict2_len = len(dict2)
total_dict_count = dict1_len + dict2_len
shared_dict = {}
for i in dict1:
if (i in dict2) and (dict1[i] == dict2[i]):
shared_dict[i] = dict1[i]
len_shared_dict = len(shared_dict)
print("The items common between the dictionaries are -", shared_dict)
print("The number of items common between the dictionaries are -", len_shared_dict)
if len_shared_dict == total_dict_count / 2:
print("The dictionaries are identical")
else:
print("The dictionaries are non-identical")
Output:
The items common between the dictionaries are - {'name': 'Tom', 'Vehicle': 'Mercedes Car'}
The number of items common between the dictionaries are - 2
The dictionaries are identical
Next, let us try an example when the dictionaries are non-identical -
dict1 = dict(name="Tom", Vehicle="Alto Car")
dict2 = dict(name="Tom", Vehicle="Mercedes Car")
dict1_len = len(dict1)
dict2_len = len(dict2)
total_dict_count = dict1_len + dict2_len
shared_dict = {}
for i in dict1:
if (i in dict2) and (dict1[i] == dict2[i]):
shared_dict[i] = dict1[i]
len_shared_dict = len(shared_dict)
print("The items common between the dictionaries are -", shared_dict)
print("The number of items common between the dictionaries are -", len_shared_dict)
if len_shared_dict == total_dict_count / 2:
print("The dictionaries are identical")
else:
print("The dictionaries are non-identical")
Output:
The items common between the dictionaries are - {'name': 'Tom'}
The number of items common between the dictionaries are - 1
The dictionaries are non-identical
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe