How to Add Dictionary to Dictionary in Python
-
Use the
update()
Method to Add a Dictionary to Another Dictionary in Python -
Use Dictionary Unpacking Operator
**
to Add a Dictionary to Another Dictionary in Python -
Use the
|
Operator to Add a Dictionary to Another Dictionary in Python -
Use the
collections.ChainMap
Container to Add a Dictionary to Another Dictionary in Python - Conclusion
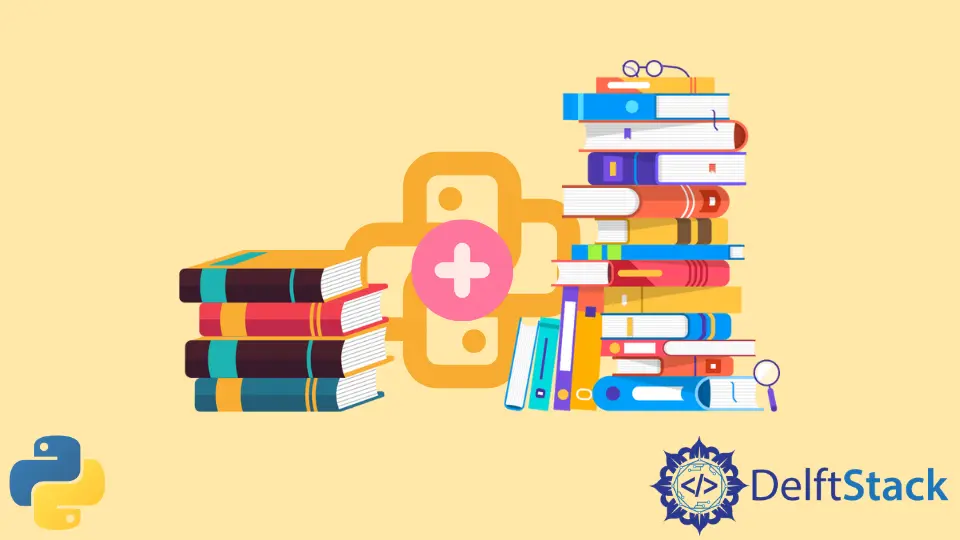
Dictionaries in Python are versatile data structures that store key-value pairs. They are unordered, mutable, and can hold any data type.
With dictionaries, you can efficiently retrieve values by referencing their corresponding keys, making them ideal for organizing and accessing data in a flexible manner. Keys are unique within a dictionary, allowing for quick searching and retrieval of values.
Dictionaries are widely used in Python for tasks like data storage, mapping, and building complex data structures. Their simplicity and power make them a fundamental tool for handling and manipulating data in Python programs.
Adding one dictionary to another in Python, also known as merging or combining dictionaries, is a valuable operation that serves various purposes. In scenarios where you have multiple dictionaries with related data, merging them becomes essential for creating a unified and comprehensive dataset.
For instance, imagine a scenario where you have one dictionary containing customer information like names and addresses and another dictionary storing their purchase history. Adding the purchase history dictionary to the customer information dictionary lets you consolidate all relevant data in one place.
This facilitates efficient data analysis, personalized recommendations, and streamlined data management. Python’s ability to add dictionaries together provides a convenient solution for integrating disparate data sources and enhancing data-driven processes.
We will discuss different methods to append a dictionary to another dictionary in Python in this tutorial.
Use the update()
Method to Add a Dictionary to Another Dictionary in Python
The update()
method in Python is a powerful tool for adding one dictionary to another, allowing you to merge their key-value pairs seamlessly. By invoking update()
on the target dictionary and passing the source dictionary as an argument, the method incorporates the key-value pairs from the source into the target dictionary.
If there are common keys between the dictionaries, the values in the source dictionary overwrite the existing values in the target dictionary. Using this method, we can add key-value pairs of one dictionary to the other dictionary.
For example,
D1 = {"loginID": "xyz", "country": "USA"}
D2 = {"firstname": "justin", "lastname": "lambert"}
D1.update(D2)
print(D1)
Output:
This code segment showcases how to add one dictionary to another in Python, effectively merging their key-value pairs. The dictionaries D1
and D2
are defined, with D1
containing information such as a login ID and country, while D2
holds details about a first name and a last name. The code utilizes the update()
method in Python to accomplish the merging.
By invoking the update()
method on D1
and passing D2
as the argument, the code adds the key-value pairs from D2
to D1
, resulting in a merged dictionary. In case of any overlapping keys between the dictionaries, the values in D2
overwrite the corresponding values in D1
.
The merged dictionary, D1
, is then printed using the print()
function. This will display the updated dictionary, including the combined key-value pairs from both D1
and D2
.
Note: The first dictionary gets updated with the values of the second dictionary. In our example,
D1
got updated.
Use Dictionary Unpacking Operator **
to Add a Dictionary to Another Dictionary in Python
In addition to the update()
method, Python offers another approach to add a dictionary to another dictionary using the dictionary unpacking operator **
. This operator allows for a concise and elegant way of merging dictionaries.
By unpacking a dictionary with **
and combining it with another dictionary, their key-value pairs are merged effortlessly. Compared to the update()
method, the dictionary unpacking operator offers a more direct and visually appealing syntax for dictionary merging.
This method does not change the key-value pairs of the original dictionary. This code segment effectively demonstrates how to add one dictionary to another in Python using the dictionary unpacking operator.
For example,
def merge(D1, D2):
py = {**D1, **D2}
return py
D1 = {"loginID": "xyz", "country": "USA"}
D2 = {"firstname": "justin", "lastname": "lambert"}
D3 = merge(D1, D2)
print(D3)
Output:
The above code segment demonstrates a Python function that merges two dictionaries, D1
and D2
, using the dictionary unpacking operator **
. The merge()
function takes two dictionaries as input parameters and combines them into a new dictionary, py
. Using the **
operator with D1
and D2
, their key-value pairs are merged effortlessly, creating a unified dictionary.
The resulting merged dictionary, D3
, is obtained by invoking the merge()
function with D1
and D2
as arguments. Finally, the merged dictionary D3
is printed using the print()
function.
Note: This operator doesn’t work on Python versions older than 3.5
Use the |
Operator to Add a Dictionary to Another Dictionary in Python
In Python, the |
(pipe) operator, also known as the union operator, provides a concise and intuitive way to add a dictionary to another dictionary. This operator performs a union operation on the dictionaries, merging their key-value pairs into a new dictionary.
Unlike the update()
method and dictionary unpacking operator **
, the |
operator combines dictionaries without modifying the original dictionaries, making it a non-destructive approach. Additionally, the |
operator allows for merging multiple dictionaries in a single expression, further enhancing flexibility. This method, alongside update()
and **
, provides developers with various options to efficiently add dictionaries together, enabling the seamless merging of dictionary data in Python.
We can use this operator in the latest update of Python (Python 3.9). It is an easy and convenient method to merge two dictionaries.
In the following code snippet, we use the |
operator.
# >= Python 3.9
def Merge(D1, D2):
py = D1 | D2
return py
D1 = {"RollNo": "10", "Age": "18"}
D2 = {"Marks": "90", "Grade": "A"}
D3 = Merge(D1, D2)
print(D3)
Output:
The above code segment demonstrates a Python function named Merge()
that adds two dictionaries, D1
and D2
, together using the |
(pipe) operator. The Merge()
function takes D1
and D2
as input parameters and returns a new merged dictionary, py
.
Using the |
operator between D1
and D2
, their key-value pairs are combined to form a unified dictionary. The resulting merged dictionary, D3
, is obtained by invoking the Merge()
function with D1
and D2
as arguments.
Finally, the merged dictionary D3
is printed using the print()
function. This code segment demonstrates a concise and effective method to add one or more dictionaries to another in Python using the |
operator.
Note: This operator doesn’t work on Python versions older than 3.9
Use the collections.ChainMap
Container to Add a Dictionary to Another Dictionary in Python
In Python, the collections.ChainMap
container provides a convenient way to add one dictionary to another. It offers a unified view of multiple dictionaries as a single entity, allowing for seamless dictionary merging.
Unlike the update()
method, dictionary unpacking operator **
, and |
operator, the collections.ChainMap
container preserves the individual dictionaries without creating a new merged dictionary. It acts as a dynamic view, providing access to all the dictionaries in the chain.
To use collections.ChainMap
, we can create a chain by passing dictionaries as arguments during initialization. Then, we can access and modify the dictionaries in the chain as if they were a single dictionary.
By leveraging the collections.ChainMap
container, we can efficiently handle scenarios where dictionaries need to be added or accessed together. It simplifies dictionary management by providing a unified interface while keeping the original dictionaries intact.
The ChainMap
container is imported from the collections
module. The following code segment demonstrates how to add one dictionary to another in Python using the collections.ChainMap
container and dictionary comprehension.
from collections import ChainMap
D1 = {"w": 1, "x": 2}
D2 = {"y": 3, "z": 4}
D3 = ChainMap(D1, D2)
D3 = {k: v for d in (D1, D2) for (k, v) in d.items()}
print(D3)
Output:
In this code, two dictionaries, D1
and D2
, are defined. To merge the dictionaries, a ChainMap
object, D3
, is created by passing D1
and D2
as arguments. This creates a view that treats D1
and D2
as a single dictionary.
Additionally, dictionary comprehension is used to create a new dictionary, D3
, by iterating over the items of D1
and D2
and mapping them to key-value pairs. This process effectively adds the key-value pairs from both dictionaries into D3
.
Finally, the merged dictionary D3
is printed using the print()
function, displaying the combined key-value pairs from D1
and D2
.
Conclusion
In conclusion, each of the four approaches discussed for adding dictionaries to one another in Python offers its own advantages and considerations.
The update()
function is simple and straightforward, modifying the original dictionary in place. However, it overwrites existing keys and values.
The **
method and the |
operator provide concise syntax, creating a new merged dictionary. They preserve the original dictionaries but may override common keys.
The collections.ChainMap
container offers a dynamic view of multiple dictionaries without creating a new merged dictionary. However, modifications to the chain affect subsequent operations.
The best method to use depends on our specific requirements. update()
is suitable for in-place modifications, while **
, |
, and ChainMap
are preferable when preserving the original dictionaries or utilizing dynamic views is essential.