__future__ in Python
-
Use
__future__
forprint_function
in Python -
Use
__future__
forunicode_laterals
in Python -
Use
__future__
for Division in Python -
Use
__future__
for Absolute_import in Python -
Use
__future__
forannotations
in Python -
Use
__future__
for Nested Scopes -
Use
__future__
for Python Generators -
Use
__future__
for thewith
Statement
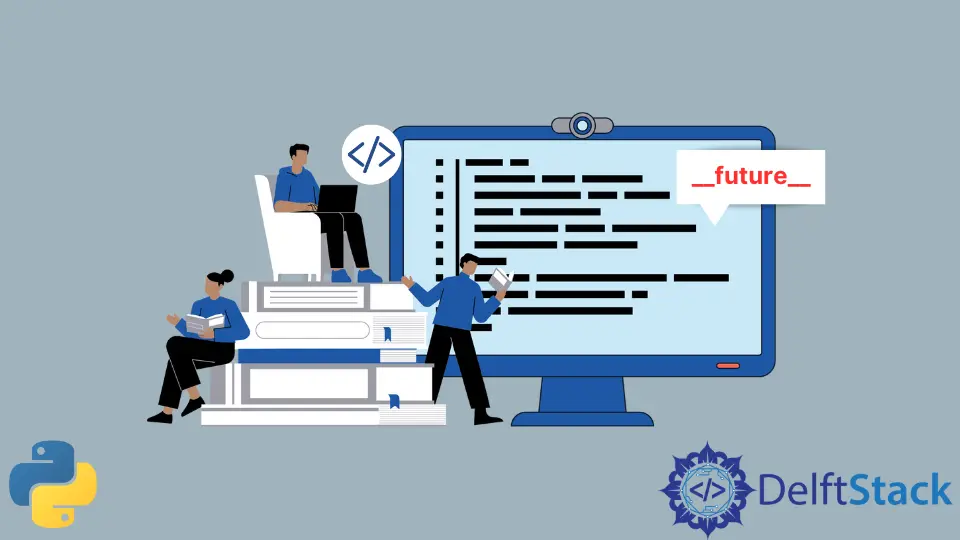
__future__
was introduced in Python 2.1, and its statements change how Python interprets the code. It tells the interpreter to compile some statements as those statements that will be available in future Python versions, i.e., Python uses from __future__ import feature
to backport the features from higher Python versions to the current interpreter.
Whenever you see from __future__ import
, it means that a feature from the latest or an upcoming release of Python has been backported into an earlier version.
This tutorial will discuss features already enabled in Python 3 in earlier versions of Python using __future__
.
Use __future__
for print_function
in Python
Using the print keyword as a function instead of a statement brings a lot of flexibility to it, which helps extend the print
keyword’s ability. The main function of from __future__ import print_function
is to bring the print
function from Python 3 into Python 2.
For example,
from __future__ import print_function
for x in range(0, 10):
print(x, sep=" ", end="")
Output:
0123456789
Note that print
here is used as a function, which was earlier used as a statement in Python 2.x
Use __future__
for unicode_laterals
in Python
This allows you to change the type of string literals.
String literals are str
by default in Python 2, but if we use from __future__ import unicode_literals
, the type of string literal changes to Unicode
.
For example,
type("h")
Output:
<type 'str'>
But with from __future__ import unicode_literals
we get the following output.
from __future__ import unicode_literals
type("h")
Output:
<type 'unicode'>
Note that using from __future__ import
, we don’t have to prefix every string with u
to treat it as a Unicode
.
Use __future__
for Division in Python
In Python 2.x versions, the classic division is used.
print(8 / 7)
Output:
0
A simple division of 8 by 7 returns 0 in Python 2.x.
The use of from __future__ import division
allows a Python 2 program to use __truediv__()
.
For example,
from __future__ import division
print(8 / 7)
Output:
1.1428571428571428
Use __future__
for Absolute_import in Python
In Python 2, you could only have implicit relative imports, whereas in Python 3, you can have explicit imports or absolute imports. __future__ import absolute_import
allows the parenthesis to have multiple import statements enclosed within the brackets.
For example,
from Tkinter import Tk, Frame, Button, Entry, Canvas, Text, LEFT, DISABLED, NORMAL, END
Note that without the __future__ import absolute_import
feature, you will not be able to enclose multiple import
statements in one line of code.
Use __future__
for annotations
in Python
Annotations are Python expressions associated with different parts of a function.
Here, using from __future__ import annotations
changes how to type annotations are evaluated in a Python module. It postpones the evaluation of annotations and magically treats all annotations as differed annotations.
For example,
from __future__ import annotations
class C:
@classmethod
def make(cls) -> C:
return cls
Note that the above code would only work if the __future__ import
is written at the top of the code, as it changes how Python interprets the code, i.e., it treats the annotations as individual strings.
Use __future__
for Nested Scopes
With the addition of __future__ import nested_scopes
, statically nested scopes have been introduced to Python. It allows the following types of code to run without returning an error.
def h():
...
def m(value):
...
return m(value - 1) + 1
...
Note that the above code would have raised a NameError before Python 2.1.
Use __future__
for Python Generators
A generator is a function in Python defined as a regular function. Still, it does that with the “yield” keyword rather than the return whenever it needs to generate a specific value.
Here, with the use of from __future__ import generators
, generator functions were introduced to save states between successive function calls.
For example,
def gen():
c, d = 0, 1
while 1:
yield d
c, d = d, c + d
Use __future__
for the with
Statement
This eliminates the use of the try...except
statements by adding the statement with
as a keyword in Python. It is mainly used while doing file I/O, as shown in the example below.
with open("workfile", "h") as a:
read_data = a.read()