Python 中的 __future__
-
在 Python 中将
__future__
用于print_function
-
在 Python 中将
__future__
用于unicode_laterals
-
在 Python 中使用
__future__
进行除法 -
在 Python 中将
__future__
用于绝对导入 -
在 Python 中使用
__future__
作为annotations
-
将
__future__
用于嵌套作用域 -
对 Python 生成器使用
__future__
-
使用
__future__
作为with
语句
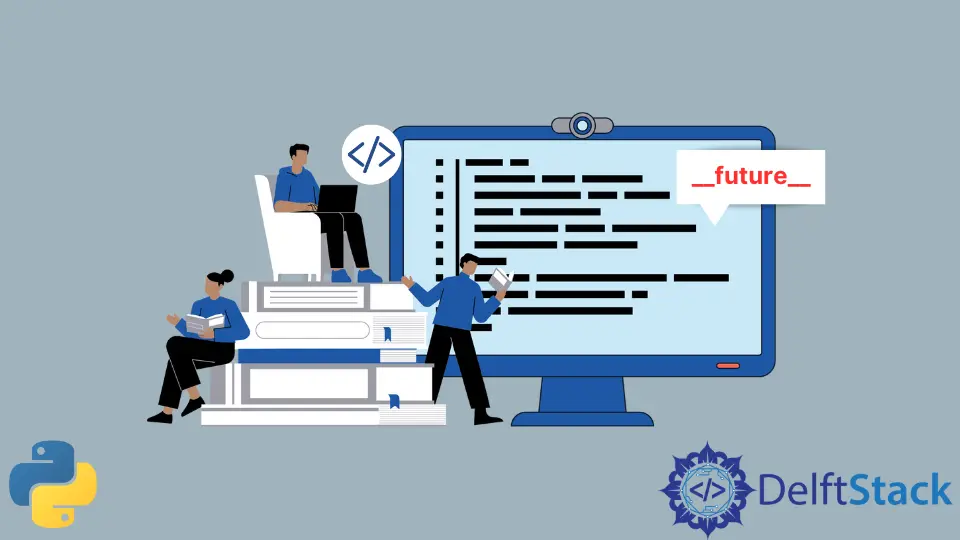
__future__
是在 Python 2.1 中引入的,它的语句改变了 Python 解释代码的方式。它告诉解释器将一些语句编译为将在未来 Python 版本中可用的那些语句,即 Python 使用 from __future__ import feature
将功能从更高的 Python 版本反向移植到当前解释器。
每当你看到 from __future__ import
时,就意味着最新或即将发布的 Python 版本中的功能已向后移植到早期版本中。
本教程将使用 __future__
讨论在早期版本的 Python 中已经在 Python 3 中启用的功能。
在 Python 中将 __future__
用于 print_function
使用 print 关键字作为函数而不是语句为其带来了很大的灵活性,这有助于扩展 print
关键字的能力。from __future__ import print_function
的主要功能是将 print
函数从 Python 3 引入 Python 2。
例如,
from __future__ import print_function
for x in range(0, 10):
print(x, sep=" ", end="")
输出:
0123456789
请注意,此处的 print
用作函数,该函数早先在 Python 2.x 中用作语句
在 Python 中将 __future__
用于 unicode_laterals
这允许你更改字符串文字的类型。
Python 2 中的字符串字面量默认为 ‘str’,但如果我们使用 from __future__ import unicode_literals
,字符串字面量的类型将更改为 Unicode
。
例如,
type("h")
输出:
<type 'str'>
但是使用 from __future__ import unicode_literals
我们得到以下输出。
from __future__ import unicode_literals
type("h")
输出:
<type 'unicode'>
请注意,使用 from __future__ import
,我们不必在每个字符串前加上 u
来将其视为 Unicode
。
在 Python 中使用 __future__
进行除法
在 Python 2.x 版本中,使用经典除法。
print(8 / 7)
输出:
0
在 Python 2.x 中,8 除以 7 的简单除法返回 0。
from __future__ import division
的使用允许 Python 2 程序使用 __truediv__()
。
例如,
from __future__ import division
print(8 / 7)
输出:
1.1428571428571428
在 Python 中将 __future__
用于绝对导入
在 Python 2 中,你只能有隐式相对导入,而在 Python 3 中,你可以有显式导入或绝对导入。__future__ import absolute_import
允许括号内包含多个导入语句。
例如,
from Tkinter import Tk, Frame, Button, Entry, Canvas, Text, LEFT, DISABLED, NORMAL, END
请注意,如果没有 __future__ import absolute_import
功能,你将无法在一行代码中包含多个 import
语句。
在 Python 中使用 __future__
作为 annotations
注释是与函数的不同部分相关联的 Python 表达式。
在这里,使用 from __future__ import annotations
改变了如何在 Python 模块中评估类型注释。它推迟对注释的评估,并神奇地将所有注释视为不同的注释。
例如,
from __future__ import annotations
class C:
@classmethod
def make(cls) -> C:
return cls
请注意,上述代码仅在 __future__ import
写在代码顶部时才有效,因为它改变了 Python 解释代码的方式,即将注释视为单个字符串。
将 __future__
用于嵌套作用域
随着 __future__ import nested_scopes
的加入,Python 中引入了静态嵌套作用域。它允许以下类型的代码运行而不返回错误。
def h():
...
def m(value):
...
return m(value - 1) + 1
...
请注意,上面的代码会在 Python 2.1 之前引发 NameError。
对 Python 生成器使用 __future__
生成器是 Python 中定义为常规函数的函数。尽管如此,它还是使用 yield
关键字而不是在需要生成特定值时返回。
在这里,通过使用 from __future__ import generators
,引入了生成器函数来保存连续函数调用之间的状态。
例如,
def gen():
c, d = 0, 1
while 1:
yield d
c, d = d, c + d
使用 __future__
作为 with
语句
这通过在 Python 中添加语句 with
作为关键字,消除了 try...except
语句的使用。它主要用于文件 I/O,如下例所示。
with open("workfile", "h") as a:
read_data = a.read()