Python 中的 __future__
-
在 Python 中將
__future__
用於print_function
-
在 Python 中將
__future__
用於unicode_laterals
-
在 Python 中使用
__future__
進行除法 -
在 Python 中將
__future__
用於絕對匯入 -
在 Python 中使用
__future__
作為annotations
-
將
__future__
用於巢狀作用域 -
對 Python 生成器使用
__future__
-
使用
__future__
作為with
語句
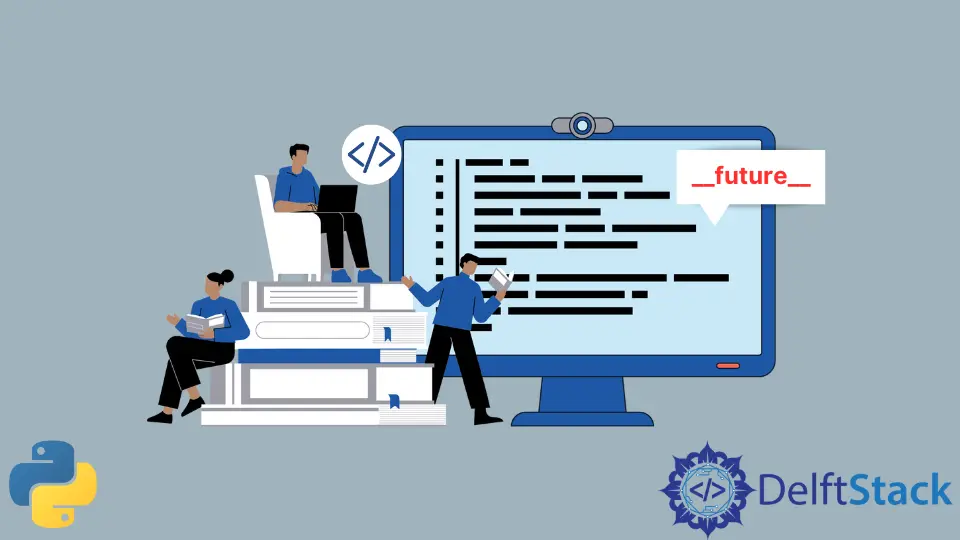
__future__
是在 Python 2.1 中引入的,它的語句改變了 Python 解釋程式碼的方式。它告訴直譯器將一些語句編譯為將在未來 Python 版本中可用的那些語句,即 Python 使用 from __future__ import feature
將功能從更高的 Python 版本反向移植到當前直譯器。
每當你看到 from __future__ import
時,就意味著最新或即將釋出的 Python 版本中的功能已向後移植到早期版本中。
本教程將使用 __future__
討論在早期版本的 Python 中已經在 Python 3 中啟用的功能。
在 Python 中將 __future__
用於 print_function
使用 print 關鍵字作為函式而不是語句為其帶來了很大的靈活性,這有助於擴充套件 print
關鍵字的能力。from __future__ import print_function
的主要功能是將 print
函式從 Python 3 引入 Python 2。
例如,
from __future__ import print_function
for x in range(0, 10):
print(x, sep=" ", end="")
輸出:
0123456789
請注意,此處的 print
用作函式,該函式早先在 Python 2.x 中用作語句
在 Python 中將 __future__
用於 unicode_laterals
這允許你更改字串文字的型別。
Python 2 中的字串字面量預設為 ‘str’,但如果我們使用 from __future__ import unicode_literals
,字串字面量的型別將更改為 Unicode
。
例如,
type("h")
輸出:
<type 'str'>
但是使用 from __future__ import unicode_literals
我們得到以下輸出。
from __future__ import unicode_literals
type("h")
輸出:
<type 'unicode'>
請注意,使用 from __future__ import
,我們不必在每個字串前加上 u
來將其視為 Unicode
。
在 Python 中使用 __future__
進行除法
在 Python 2.x 版本中,使用經典除法。
print(8 / 7)
輸出:
0
在 Python 2.x 中,8 除以 7 的簡單除法返回 0。
from __future__ import division
的使用允許 Python 2 程式使用 __truediv__()
。
例如,
from __future__ import division
print(8 / 7)
輸出:
1.1428571428571428
在 Python 中將 __future__
用於絕對匯入
在 Python 2 中,你只能有隱式相對匯入,而在 Python 3 中,你可以有顯式匯入或絕對匯入。__future__ import absolute_import
允許括號內包含多個匯入語句。
例如,
from Tkinter import Tk, Frame, Button, Entry, Canvas, Text, LEFT, DISABLED, NORMAL, END
請注意,如果沒有 __future__ import absolute_import
功能,你將無法在一行程式碼中包含多個 import
語句。
在 Python 中使用 __future__
作為 annotations
註釋是與函式的不同部分相關聯的 Python 表示式。
在這裡,使用 from __future__ import annotations
改變了如何在 Python 模組中評估型別註釋。它推遲對註釋的評估,並神奇地將所有註釋視為不同的註釋。
例如,
from __future__ import annotations
class C:
@classmethod
def make(cls) -> C:
return cls
請注意,上述程式碼僅在 __future__ import
寫在程式碼頂部時才有效,因為它改變了 Python 解釋程式碼的方式,即將註釋視為單個字串。
將 __future__
用於巢狀作用域
隨著 __future__ import nested_scopes
的加入,Python 中引入了靜態巢狀作用域。它允許以下型別的程式碼執行而不返回錯誤。
def h():
...
def m(value):
...
return m(value - 1) + 1
...
請注意,上面的程式碼會在 Python 2.1 之前引發 NameError。
對 Python 生成器使用 __future__
生成器是 Python 中定義為常規函式的函式。儘管如此,它還是使用 yield
關鍵字而不是在需要生成特定值時返回。
在這裡,通過使用 from __future__ import generators
,引入了生成器函式來儲存連續函式呼叫之間的狀態。
例如,
def gen():
c, d = 0, 1
while 1:
yield d
c, d = d, c + d
使用 __future__
作為 with
語句
這通過在 Python 中新增語句 with
作為關鍵字,消除了 try...except
語句的使用。它主要用於檔案 I/O,如下例所示。
with open("workfile", "h") as a:
read_data = a.read()