How to Set Text of Tkinter Entry Widget With a Button
-
Tkinter
delete
andinsert
Method to Set the Content ofEntry
-
Tkinter
StringVar
Method to Set Content of TkinterEntry
Widget
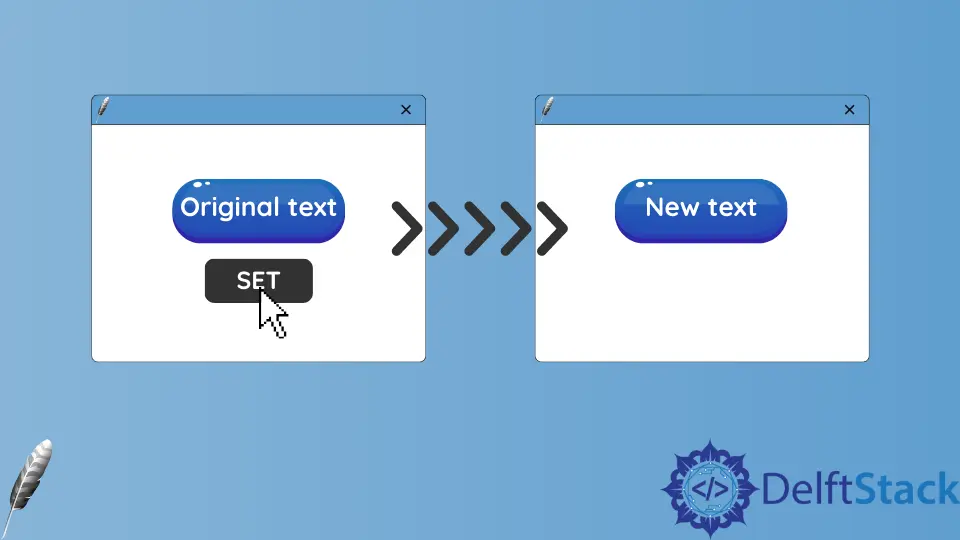
We have two methods to set or change the text of Tkinter Entry
widget by clicking a Tkinter button,
- Tkinter
delete
andinsert
Method - Tkinter
StringVar
Method
Tkinter delete
and insert
Method to Set the Content of Entry
Tkinter Entry
widget doesn’t have a dedicated set
method to set the content of the Entry
. It needs to first delete the existing content and then insert the new content if we have to change the content completely.
Complete Working Codes to Set Text in Entry
With delete
and insert
Methods
import tkinter as tk
root = tk.Tk()
root.geometry("400x50")
def setTextInput(text):
textExample.delete(0, "end")
textExample.insert(0, text)
textExample = tk.Entry(root)
textExample.pack()
btnSet = tk.Button(
root, height=1, width=10, text="Set", command=lambda: setTextInput("new content")
)
btnSet.pack()
root.mainloop()
textExample.delete(0, "end")
delete
method of Entry
deletes the specified range of characters in the Entry
.
0
is the first character and "end"
is the last character of the content in the Entry
widget. Therefore, delete(0, "end")
deletes all the content inside Entry
box.
textExample.insert(0, text)
insert
method inserts the text at the specified position. In the above code, it inserts the text
at the beginning.
Tkinter StringVar
Method to Set Content of Tkinter Entry
Widget
If the content of Tkinter Entry
widget has been associated with a StringVar
object, it could automatically change the content of Tkinter Entry
widget every time the value of StringVar
is updated.
Complete Working Codes to Set Text in Entry
With StringVar
Object
import tkinter as tk
root = tk.Tk()
root.geometry("400x50")
def setTextInput(text):
textEntry.set(text)
textEntry = tk.StringVar()
textExample = tk.Entry(root, textvariable=textEntry)
textExample.pack()
btnSet = tk.Button(
root, height=1, width=10, text="Set", command=lambda: setTextInput("new content")
)
btnSet.pack()
root.mainloop()
textEntry = tk.StringVar()
textExample = tk.Entry(root, textvariable=textEntry)
textEntry
is a StringVar
object and it is associated with the text content, or in other words textvariable
option in Entry
widget.
textEntry.set(text)
If textEntry
is updated to have a new value text
, then the widgets whose textvariable
are associated with it will be automatically updated.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook