How to Declare Global Variable in PHP
-
Use the
global
Keyword to Declare a Global Variable in a Local Scope in PHP -
Use the
$GLOBALS
Super Global Variable to Use the Global Variable in the Local Scope in PHP -
Use the
define()
Function to Define a Constant Global Variable in PHP
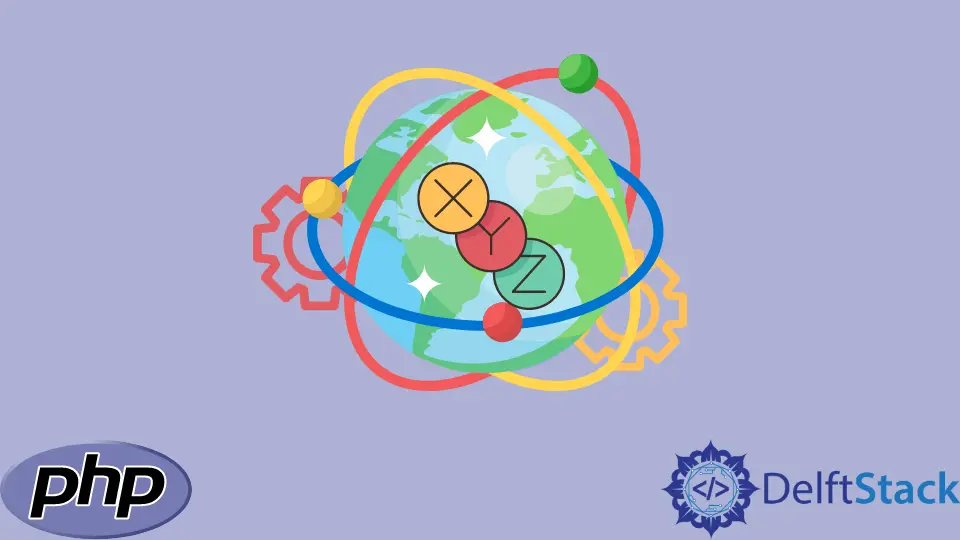
We will introduce a method to declare a global variable in PHP using the global
keyword. This method will set the global scope of the variable declared outside of a function to the local scope to use the variable inside the function.
This article will demonstrate another method to declare a global variable in PHP using the $GLOBALS
super global variable. This method will use the super global variable to access the global variable in a local scope.
We will also demonstrate another method to declare a global variable in PHP using the define()
function. This method is a way of declaring a constant global variable in PHP. We cannot change the value of the constant.
Use the global
Keyword to Declare a Global Variable in a Local Scope in PHP
We can use the global
keyword to declare a global variable in a local scope in PHP. The global
keyword sets the global scope as a variable to the local scope. We can define a variable outside a function. When we try to access the variable inside the function, the global scope of the variable won’t let us. So we can use the global
keyword inside the function with the variable. Then, we can access the variable inside the function.
For example, create a variable $crypto
and assign Bitcoin
to it. Then, create a function body()
. Inside the function, use the global
keyword before the $crypto
variable. Print the $crypto
variable using the echo
statement and concatenate the string is a top cryptocurrency.
using the .
dot operator. Outside the function body, call the body()
function.
The example below imports the global scope of the $crypto
variable to the local scope inside the body()
function. Thus, we can access the $crypto
variable inside the function. When we try to print the variable without using the global
keyword, we won’t be able to do that because the local scope of the variable does not exist. Check the PHP Manual to learn more about the scopes and the global
keyword.
Example Code:
#php 7.x
<?php
$crypto = 'Bitcoin';
function body(){
global $crypto;
echo $crypto." is a top cryptocurrency.";
}
body();
?>
Output:
Bitcoin is a top cryptocurrency.
Use the $GLOBALS
Super Global Variable to Use the Global Variable in the Local Scope in PHP
We can use the $GLOBALS
super global variable to reference the global scope variables. The $GLOBALS
variable is an associative array that contains the reference of the variables defined in the global scope. We can write the variable inside the $GLOBALS
brackets to reference the global variable as $GLOBALS["name"]
. This method attempts to print a local variable and a global variable inside a function. It uses the $GLOBALS
super global variable to reference a variable in a global scope inside the function.
For example, create a variable $var
and assign a string global scope variable
. Write a function body()
and create the same variable $var
inside the function and assign the string local scope variable
this time. Firstly, echo the $var
variable referencing it in the $GLOBALS
array as $GLOBALS["var"]
. In the next line, print the $var
variable with the echo
statement.
The example below prints the $var
variables two times. The first one uses the $GLOBALS["var"]
array, and as a result, it prints the global variable defined outside the function. In the second time, the variable inside the function displays. Check the PHP Manual to know more about the $GLOBALS
super global variable.
Code Example:
#php 7.x
<?php
$var = "global scope variable";
function body() {
$var = "local scope variable";
echo '$var in global scope: ' . $GLOBALS["var"] . "<br>";
echo '$var in current scope: ' . $var ;
}
body();
?>
Output:
$var in global scope: global scope variable
$var in current scope: local scope variable
Use the define()
Function to Define a Constant Global Variable in PHP
This method uses the define()
function to define a global variable in PHP. The function takes two parameters. The first parameter is the constant name, and the second is the value of the constant. The constant is case-insensitive by default. We can access the constant from anywhere in the script. There is no use of the $
sign while defining a constant. As the name stands, its value is immutable. The constant only can hold the string and number as the value.
For example, write a define()
function and give the name of the constant as BAND
and the value as Opeth
. Create a function bandName()
and use the echo statement to display the BAND
constant. Outside the function, call the function bandName()
. The script will output the value Opeth
. Check the PHP Manual to learn more about the define()
function.
Example Code:
# php 7.x
<?php
define('BAND', 'Opeth');
function bandName()
{
echo BAND;
}
bandName();
?>
Output:
Opeth
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn