How to Resize Image in PHP
- Load the Image Before Resizing
-
imagecopyresized()
in PHP -
imagecopyresampled()
in PHP -
imagescale()
in PHP
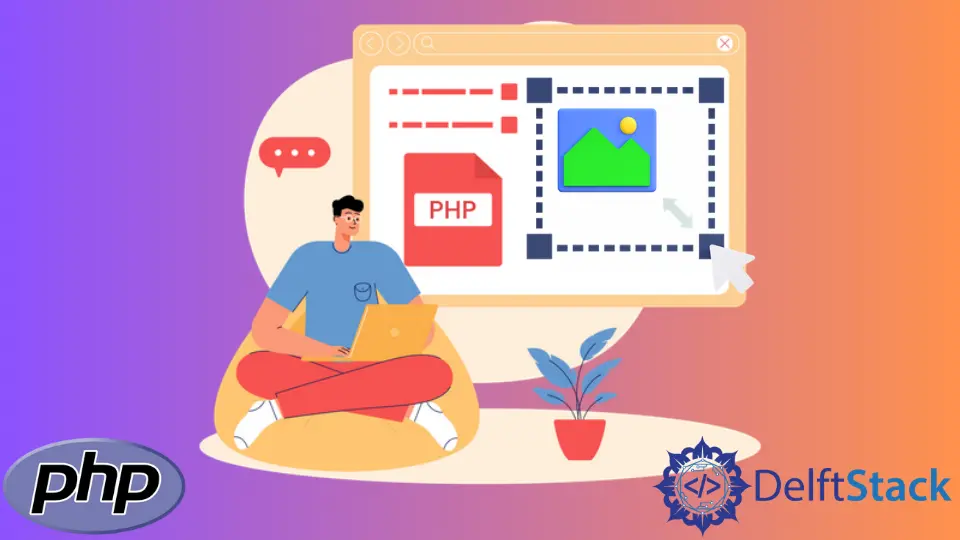
In this tutorial article, we will discuss resizing an image in PHP.
Load the Image Before Resizing
Before we resize an image, we must first load it as an image resource within the script. It is not the same as using functions like file_get_contents()
to get the image file’s content. To load the file, we need to use functions like imagecreatefromjpeg()
, imagecreatefrompng()
, and imagecreatefromgif()
, among others. Depending on the type of image we will resize, we will use a different function accordingly.
getimagesize()
in PHP
After loading the image, we use getimagesize()
to calculate the width, height and the type of the input image. This function returns a list of items, where the image’s width and height are stored at indexes 0 and 1, respectively, and the IMAGETYPE_XXX
constants are stored at index 2. We’ll use the value of this returned constant to figure out what kind of image to use and which feature to use.
<?php
function load_image($filename, $type) {
$new = 'new34.jpeg';
if( $type == IMAGETYPE_JPEG ) {
$image = imagecreatefromjpeg($filename);
echo "here is jpeg output:";
imagejpeg($image, $new);
}
elseif( $type == IMAGETYPE_PNG ) {
$image = imagecreatefrompng($filename);
echo "here is png output:";
imagepng($image,$new);
}
elseif( $type == IMAGETYPE_GIF ) {
$image = imagecreatefromgif($filename);
echo "here is gif output:";
imagejpeg($image, $new);
}
return $new;
}
$filename = "panda.jpeg";
list($width, $height,$type) = getimagesize($filename);
echo "Width:" , $width,"\n";
echo "Height:", $height,"\n";
echo "Type:", $type, "\n";
$old_image = load_image($filename, $type);
?>
Input_Image:
Output:
Width:225
Height:225
Type:2
here is jpeg output:
Output_image:
Both are the same images because we only load the image and calculate the size of the original image in this section.
imagecopyresized()
in PHP
imagecopyresized()
takes an oblong area of width src_w
and height src_h
from src_image
at position (src_x,src_y)
and places it during a rectangular area of destination image at position (dst_x,dst_y)
. It is a built-in function in PHP.
<?php
// File and new size
$filename = 'panda.jpeg';
$percent = 0.5;
// Content type
header('Content-Type: image/jpeg');
// Get new sizes
list($width, $height, $type) = getimagesize($filename);
$newwidth = $width * $percent;
$newheight = $height * $percent;
$type = $list[2];
echo $type;
// Load
$thumb = imagecreatetruecolor($newwidth, $newheight);
$source = imagecreatefromjpeg($filename);
// Resize
imagecopyresized($thumb, $source, 0, 0, 0, 0, $newwidth, $newheight, $width, $height);
// Output
imagejpeg($thumb,'new.jpeg');
?>
Input_Image:
Output_image:
As discussed above, the imagecopyresize()
function accepts ten parameters, which are listed below.
Parameters | Explanation |
---|---|
$dst_image |
The destination image resource |
$src_image |
The source image resource |
$dst_x |
The x-coordinate of destination point |
$dst_y |
The y-coordinate of destination point |
$src_x |
The x-coordinate of source point |
$src_y |
The y-coordinate of source point |
$dst_w |
The destination width |
$dst_h |
The destination height |
$src_w |
The source width |
$src_h |
The source height |
It returns the boolean value TRUE on success or FALSE on failure.
imagecopyresampled()
in PHP
imagecopyresampled()
copies an oblong portion of one image to a different, seamlessly interpolating pixel values to reduce the dimension of a picture, maintaining a high level of clarity.
It functions similarly to the imagecopyresized()
feature, with the added benefit of sampling the image in addition to resizing it.
<?php
// The file
$filename = 'panda.jpeg';
$percent = 2;
// Content type
header('Content-Type: image/jpeg');
// Get new dimensions
list($width, $height) = getimagesize($filename);
$new_width = $width * $percent;
$new_height = $height * $percent;
// Resample
$image_p = imagecreatetruecolor($new_width, $new_height);
$image = imagecreatefromjpeg($filename);
imagecopyresampled($image_p, $image, 0, 0, 0, 0, $new_width, $new_height, $width, $height);
// Output
imagejpeg($image_p, 'resampled1.jpeg', 100);
?>
Input_Image:
Output_image:
The function imagecopyresampled()
accepts ten different parameters.
Parameters | Explanation |
---|---|
$image_p |
It determine the destination image. |
$image |
It determine the source image. |
$x_dest |
It determine the x-coordinate of destination image. |
$y_dest |
It determine the y-coordinate of destination image. |
$x_src |
It determine the x-coordinate of source image. |
$y_src |
It determine the y-coordinate of source image. |
$wid_dest |
It determine the width of new image. |
$hei_dest |
It determine the height of new image. |
$wid_src |
It determine the width of old image. |
$wid_src |
It determine the height of old image. |
dest_image
instead of percent.imagescale()
in PHP
You specify the size rather than defining the width or height of the latest image. If you would like the new image size to be half the first image, you set the size to 0.8. Here is that the example code to scale a picture by a given factor while preserving the ratio.
We multiply the original width and height of the image with the specified scale within the scale image()
function.
here is its example:
<?php
function load_image($filename, $type) {
if( $type == IMAGETYPE_JPEG ) {
$image = imagecreatefromjpeg($filename);
}
elseif( $type == IMAGETYPE_PNG ) {
$image = imagecreatefrompng($filename);
}
elseif( $type == IMAGETYPE_GIF ) {
$image = imagecreatefromgif($filename);
}
return $image;
}
function scale_image($scale, $image, $width, $height) {
$new_width = $width * $scale;
$new_height = $height * $scale;
return resize_image($new_width, $new_height, $image, $width, $height);
}
function resize_image($new_width, $new_height, $image, $width, $height) {
$image12 = 'hello.jpeg';
$new_imag = imagecreatetruecolor($new_width, $new_height);
imagecopyresampled($new_imag, $image, 0, 0, 0, 0, $new_width, $new_height, $width, $height);
return imagejpeg($new_imag, $image12);
}
$filename = "panda.jpeg";
list($width, $height, $type) = getimagesize($filename);
$old_image = load_image($filename, $type);
$image_scaled = scale_image(0.8, $old_image, $width, $height);
?>
Input_Image:
Output_image:
Aside from that, PHP has a built-in feature that allows you to scale an image to the specified width and height.
See the example code.
<?php
$out_image = "sca.jpeg";
$image_name ="panda.jpeg";
// Load image file
$image = imagecreatefromjpeg($image_name);
// Use imagescale() function to scale the image
$img = imagescale( $image, 500, 400 );
header("Content-type: image/jpeg");
imagejpeg($img,$out_image);
?>
Input_Image:
Output_Image: