How to Replace Values in NumPy
-
NumPy Replace Values With the
numpy.clip()
Function -
NumPy Replace Values With the
numpy.minimum()
andnumpy.maximum()
Functions - NumPy Replace Values With the Array Indexing Method in Python
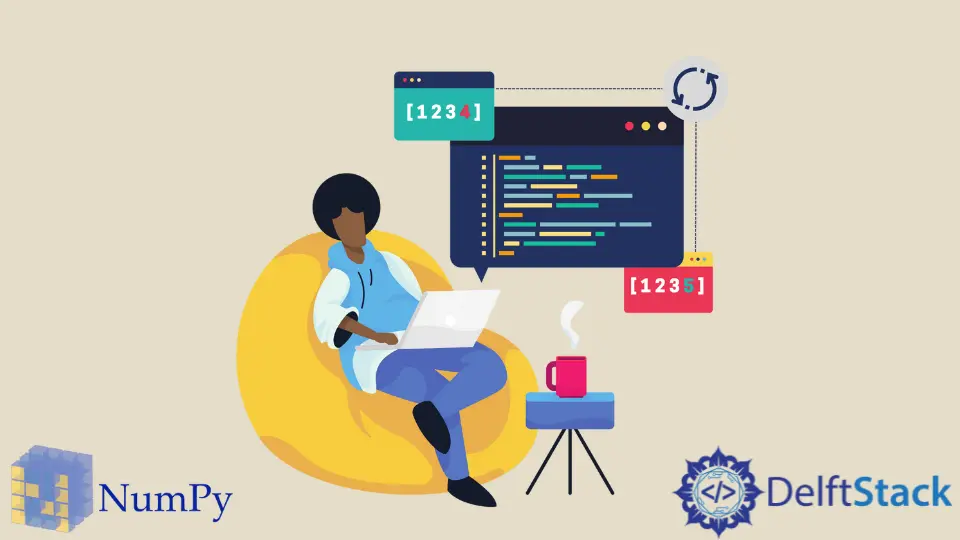
This tutorial will introduce how to replace values inside a NumPy array in Python.
NumPy Replace Values With the numpy.clip()
Function
If we need to replace all the greater values than a certain threshold in a NumPy array, we can use the numpy.clip()
function. We can specify the upper and the lower limits of an array using the numpy.clip()
function. The numpy.clip()
function returns an array where the elements less than the specified limit are replaced with the lowest limit. The elements greater than the specified limit are replaced with the greatest limit. The following code example shows us how to replace values inside a NumPy array with the numpy.clip()
function.
import numpy as np
array = np.array([1, 2, 3, 4, 5, 5, 6, 7, 8, 8, 9, 9])
result = np.clip(array, 0, 5)
print(result)
Output:
[1 2 3 4 5 5 5 5 5 5 5 5]
We replaced the values greater than 5
inside the NumPy array array
with the np.clip()
function in the above code. We first created a NumPy array with the np.array()
function. We then clipped the array
by specifying a limit from 0
to 5
inside the np.clip()
function and saved the result inside the result
array.
NumPy Replace Values With the numpy.minimum()
and numpy.maximum()
Functions
We can also use the numpy.minimum()
and the numpy.maximum()
functions to replace values in an array outside our specified limit. The numpy.maximum()
function is used to replace the values less than the lower limit with the lower limit. And the numpy.minimum()
function is used to replace values greater than the upper limit with the upper limit. The numpy.maximum()
function takes the array and the lowest possible value as input parameters. The numpy.minimum()
function takes the array and the greatest possible value as input parameters. See the following code example.
import numpy as np
array = np.array([1, 2, 3, 4, 5, 5, 6, 7, 8, 8, 9, 9])
result1 = np.minimum(array, 5)
result2 = np.maximum(result1, 0)
print(result2)
Output:
[1 2 3 4 5 5 5 5 5 5 5 5]
We replaced the values greater than 5
with 5
by using the np.minimum()
function and the values less than 0
with 0
by using the np.maximum()
function. We stored the result of these operations inside the result2
array.
NumPy Replace Values With the Array Indexing Method in Python
The simplest way of achieving the same goal as the previous two methods is to use the array indexing in Python. We can easily replace values greater than or less than a certain threshold with the array indexing method in NumPy. Rather than creating a new array like the previous two methods, this method modified the contents of our original array.
import numpy as np
array = np.array([1, 2, 3, 4, 5, 5, 6, 7, 8, 8, 9, 9])
array[array > 5] = 5
print(array)
Output:
[1 2 3 4 5 5 5 5 5 5 5 5]
We replaced all the values inside the array
greater than 5
with 5
by using array[array > 5] = 5
in Python.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn