How to Calculate the Mode of Array in NumPy
-
Calculate the Mode of a NumPy Array Using the
scipy.stats.mode
Function -
Calculate the Mode of a NumPy Array Using the
statistics
Module - Calculate the Mode of a NumPy Array Using a User-Defined Function
- Conclusion
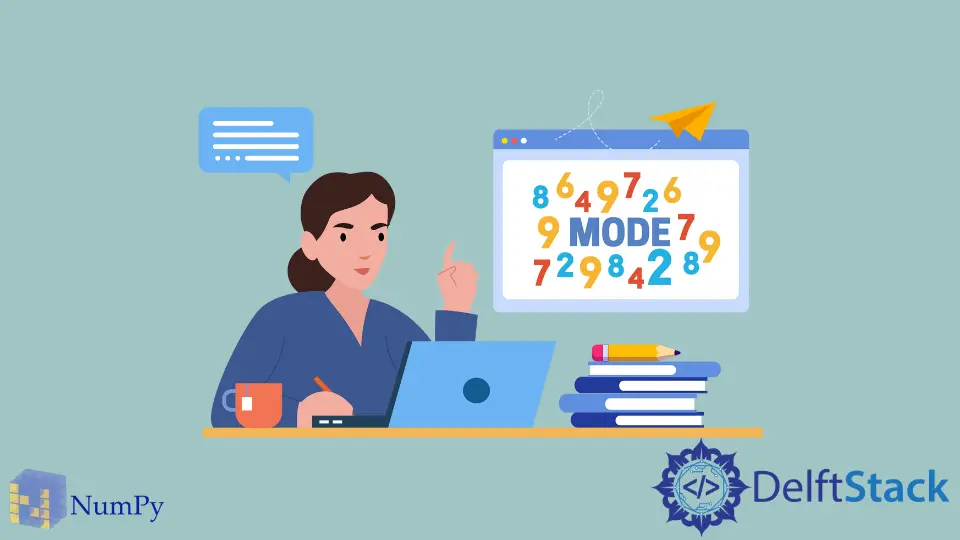
NumPy, a powerful numerical computing library in Python, provides an array object that is essential for scientific computing. While NumPy offers a plethora of functionalities, it lacks a direct method for calculating the mode of an array.
However, there are several approaches you can take to determine the mode, and in this article, we’ll explore three methods using the scipy.stats
package, the statistics
module, and a user-defined function.
Calculate the Mode of a NumPy Array Using the scipy.stats.mode
Function
The scipy.stats
module is a part of the SciPy library, which builds on NumPy to provide additional functionality for statistical analysis.
One of the functions it offers is mode
, designed to calculate the mode of a dataset. The mode represents the value(s) that appear most frequently in the dataset.
Here is the syntax for the scipy.stats.mode
function:
scipy.stats.mode(a, axis=0, nan_policy="propagate")
Parameters:
a
: This is the input array or object that can be converted to an array.axis
(Optional): The axis along which the mode is calculated. By default, it is0
.nan_policy
(Optional): This defines how to handle when input containsNaN
.
The default for nan_policy
is propagate
, which means if there are any NaN
values in the input, the result will be NaN
. Other options include raise
, which raises an error if there are any NaN
values, and omit
, which performs the calculations ignoring NaN
values.
Let’s dive into an example to demonstrate how to use scipy.stats.mode
to find the mode of a NumPy array.
from scipy.stats import mode
import numpy as np
data = np.array([1, 2, 2, 3, 4, 5, 5, 5, 6])
mode_result = mode(data)
print("Mode:", mode_result.mode)
print("Count:", mode_result.count)
Output:
Mode: 5
Count: 3
In this code snippet, the first two lines of code import the necessary modules, with mode
being specifically imported from scipy.stats
and NumPy imported with the alias np
.
The NumPy array, named data
, is then defined, containing a sequence of numerical values. The mode
function is applied to the data
array, resulting in the mode_result
object.
The mode value is accessed using mode_result.mode[0]
, and the count of occurrences is obtained with mode_result.count[0]
.
Finally, the calculated mode and its corresponding count are printed to the console using the print
statements.
Calculate the Mode of a NumPy Array Using the statistics
Module
The statistics
module is a part of the Python standard library, offering various functions for statistical calculations. Among these functions is mode
, which calculates the mode of a dataset.
While NumPy provides powerful numerical operations, the statistics
module can complement it by offering specialized statistical functions.
Let’s delve into an example to illustrate how to use the statistics
module to calculate the mode of a NumPy array:
import statistics
import numpy as np
data = np.array([1, 2, 2, 3, 4, 5, 5, 5, 6])
mode_result = statistics.mode(data)
print("Mode:", mode_result)
Output:
Mode: 5
As you can see in the first two lines, the statistics
module and numpy
are imported with the aliases statistics
and np
, respectively. The NumPy array, named data
, is then defined, containing a set of numerical values.
Subsequently, the statistics.mode()
function is applied to the data
array, calculating the mode of the dataset. The result is stored in the variable mode_result
.
Finally, the mode is printed to the console using the print
statement.
It’s important to note that while the statistics
module’s mode
function is designed for lists, it seamlessly handles NumPy arrays, showcasing the flexibility of using different Python libraries in tandem for statistical analysis.
Calculate the Mode of a NumPy Array Using a User-Defined Function
While NumPy offers powerful functions for numerical analysis, finding the mode directly is not among them. This lack of a built-in mode function opens the door for a customized solution.
By creating a user-defined function, we can tailor the mode calculation to meet specific requirements.
Let’s begin by crafting a user-defined function that utilizes NumPy for mode calculation:
import numpy as np
def calculate_mode(arr):
unique_values, counts = np.unique(arr, return_counts=True)
max_count_index = np.argmax(counts)
mode_value = unique_values[max_count_index]
mode_count = counts[max_count_index]
return mode_value, mode_count
Here, we have a user-defined function named calculate_mode
that utilizes the NumPy library to calculate the mode of a given NumPy array.
In the first line, NumPy is imported with the alias np
. The function takes a single argument, arr
, representing the input NumPy array for which the mode needs to be calculated.
Within the function, the np.unique()
function is employed to obtain two arrays: unique_values
containing the unique elements of the input array and counts
containing the corresponding counts of each unique element.
The next line identifies the index of the maximum count in the counts
array using np.argmax()
. Subsequently, the mode value is determined by retrieving the element at the computed index from the unique_values
array, and the mode count is obtained similarly from the counts
array.
Finally, the function returns a tuple consisting of the mode value and its corresponding count.
Let’s demonstrate how to use this user-defined function to calculate the mode of a NumPy array:
import numpy as np
def calculate_mode(arr):
unique_values, counts = np.unique(arr, return_counts=True)
max_count_index = np.argmax(counts)
mode_value = unique_values[max_count_index]
mode_count = counts[max_count_index]
return mode_value, mode_count
data = np.array([1, 2, 2, 3, 4, 5, 5, 5, 6])
mode_value, mode_count = calculate_mode(data)
print("Mode:", mode_value)
print("Count:", mode_count)
Output:
Mode: 5
Count: 3
This user-defined function provides a foundation that can be customized based on specific requirements. Whether you need additional functionalities, such as handling NaN
values or accommodating multidimensional arrays, you can modify the function to suit your use case.
Conclusion
Calculating the mode of a NumPy array in Python can be achieved through various methods, each catering to different preferences and library dependencies. Whether you opt for the scipy.stats
package, the statistics
module, or a custom function, understanding these approaches allows you to choose the one that best fits your specific use case.
Whichever method you choose, NumPy remains a fundamental tool for numerical computing in the Python ecosystem.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn