How to Add Dimension to NumPy Array
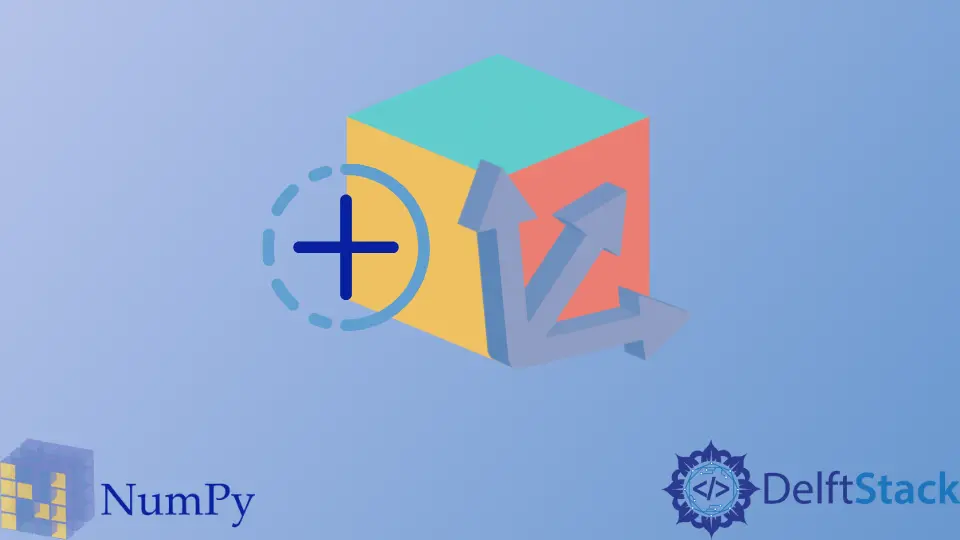
Adding dimensions to a NumPy array can be a crucial step in data manipulation, especially when preparing data for machine learning or other analytical tasks. In Python, there are two primary methods to achieve this: the numpy.expand_dims()
function and the numpy.newaxis
method. These techniques allow you to reshape your arrays, making it possible to add a new axis without altering the data itself.
In this article, we will explore both methods in detail, complete with code examples and explanations. By the end, you will have a solid understanding of how to add dimensions to your NumPy arrays effectively.
Using numpy.expand_dims()
The numpy.expand_dims()
function is a straightforward way to add a new dimension to your array. This method takes two arguments: the array you want to expand and the axis along which you want to add the new dimension. The axis can be specified as an integer, allowing you to choose the position of the new dimension in your array’s shape.
Here’s how you can use numpy.expand_dims()
:
import numpy as np
array_1d = np.array([1, 2, 3, 4])
expanded_array = np.expand_dims(array_1d, axis=0)
print(expanded_array)
Output:
[[1 2 3 4]]
In this example, we start with a one-dimensional array array_1d
containing four elements. By using np.expand_dims()
and specifying axis=0
, we add a new dimension at the front, transforming our array into a two-dimensional array. The result is a 2D array with one row and four columns. You can also add dimensions at different positions by changing the axis value. For instance, if you set axis=1
, the new dimension will be added as a column instead of a row.
This function is particularly useful when you need to prepare your data for operations that require specific dimensions, such as broadcasting or matrix multiplication. Understanding how to manipulate the shape of your arrays can significantly enhance your data processing capabilities in Python.
Using numpy.newaxis
Another powerful method for adding dimensions to a NumPy array is using numpy.newaxis
. This is a convenient way to increase the dimensions of an existing array without changing its data. By inserting np.newaxis
in the array’s indexing, you can specify where you want the new dimension to be added.
Here’s an example of how to use numpy.newaxis
:
import numpy as np
array_1d = np.array([1, 2, 3, 4])
expanded_array = array_1d[np.newaxis, :]
print(expanded_array)
Output:
[[1 2 3 4]]
In this code snippet, we again start with a one-dimensional array array_1d
. By using array_1d[np.newaxis, :]
, we insert a new axis at the front of the array. The result is the same as with np.expand_dims()
, yielding a two-dimensional array.
You can also use np.newaxis
to add dimensions at different positions. For example, if you want to add a new dimension at the end, you can do so by placing np.newaxis
after the colon:
expanded_array = array_1d[:, np.newaxis]
This will convert the original 1D array into a 2D column vector. The flexibility of numpy.newaxis
makes it an excellent tool for reshaping your data, especially when you’re dealing with multi-dimensional arrays or need to prepare your data for functions that require specific input shapes.
In summary, both numpy.expand_dims()
and numpy.newaxis
provide effective ways to add dimensions to your NumPy arrays. Depending on your needs and coding style, you can choose the method that best suits your workflow.
Conclusion
Adding dimensions to NumPy arrays is a fundamental skill for anyone working with data in Python. Whether you choose to use numpy.expand_dims()
or numpy.newaxis
, both methods offer powerful capabilities for reshaping your data. By mastering these techniques, you can ensure your arrays are in the correct format for further analysis or machine learning tasks. As you continue to explore the world of data science, understanding how to manipulate array dimensions will undoubtedly enhance your efficiency and effectiveness.
FAQ
-
what is the purpose of adding dimensions to a NumPy array?
Adding dimensions helps reshape the data for various operations, making it compatible with functions that require specific input shapes. -
can I use both methods interchangeably?
Yes, both methods achieve the same result, but the choice depends on personal preference and coding style. -
how does adding dimensions affect array operations?
Adding dimensions can change how broadcasting works, allowing for more complex mathematical operations between arrays of different shapes. -
are there any performance implications when using these methods?
Generally, both methods are efficient. However, the choice of method may depend on the context and readability of your code. -
can I remove dimensions from a NumPy array?
Yes, you can remove dimensions using thenumpy.squeeze()
function, which removes single-dimensional entries from the shape of an array.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn