How to Append Data to Div in JavaScript
- Using innerHTML to Append Data
- Using createElement and appendChild
- Using insertAdjacentHTML
- Conclusion
- FAQ
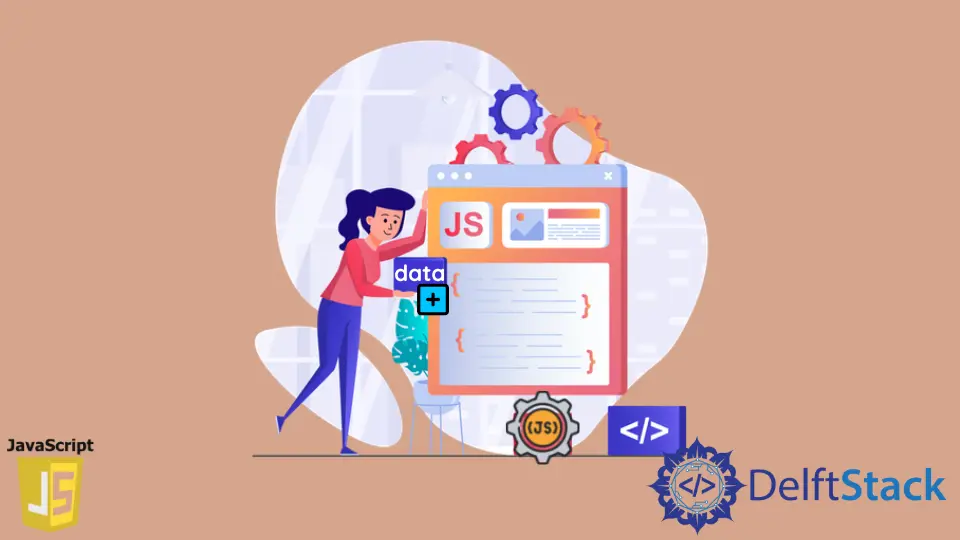
Appending data to a div in JavaScript is a fundamental skill for web developers. Whether you’re dynamically adding content to enhance user experience or simply updating the interface, knowing how to manipulate the DOM (Document Object Model) is crucial.
In this tutorial, we will explore various methods to append data to a div using JavaScript. We will cover simple techniques, such as using innerHTML, and more advanced methods like createElement. By the end of this guide, you’ll have a solid understanding of how to efficiently add content to your web pages using JavaScript.
Using innerHTML to Append Data
One of the simplest ways to append data to a div is by using the innerHTML
property. This method allows you to directly set or modify the HTML content of an element. Here’s how you can do it:
const myDiv = document.getElementById('myDiv');
myDiv.innerHTML += '<p>This is a new paragraph.</p>';
Output:
This is a new paragraph.
In this example, we first select the div using document.getElementById
. The innerHTML
property is then used to add a new paragraph. The +=
operator is crucial here as it appends the new content rather than replacing the existing content. This method is straightforward and works well for simple text and HTML structures. However, it’s important to note that using innerHTML
can lead to performance issues if used excessively or with complex structures, as it re-parses the entire HTML content of the div every time it is called.
Using createElement and appendChild
For a more structured approach, you can use the createElement
method to create new elements and then append them to the div using appendChild
. This method is more efficient and safer, especially when dealing with user-generated content.
const myDiv = document.getElementById('myDiv');
const newParagraph = document.createElement('p');
newParagraph.textContent = 'This is a new paragraph created with createElement.';
myDiv.appendChild(newParagraph);
Output:
This is a new paragraph created with createElement.
In this example, we create a new paragraph element using document.createElement
. We then set its text content with textContent
to avoid any HTML parsing issues. Finally, we append the new paragraph to the div using appendChild
. This method is particularly useful when you need to add multiple elements or more complex structures, as it allows for better control over the DOM and prevents potential security vulnerabilities related to HTML injection.
Using insertAdjacentHTML
Another effective method to append content to a div is by using insertAdjacentHTML
. This method allows you to insert HTML at specific positions relative to the target element. It’s a great option when you want to control exactly where the new content appears.
const myDiv = document.getElementById('myDiv');
myDiv.insertAdjacentHTML('beforeend', '<p>This paragraph is added with insertAdjacentHTML.</p>');
Output:
This paragraph is added with insertAdjacentHTML.
In this code snippet, insertAdjacentHTML
is called on the target div. The first argument, 'beforeend'
, specifies that the new content will be added at the end of the div. This method is advantageous because it doesn’t re-parse the entire HTML like innerHTML
, making it more efficient for performance. You can also use other positions such as 'afterbegin'
, 'beforebegin'
, and 'afterend'
, giving you flexibility in how you append your content.
Conclusion
In conclusion, appending data to a div in JavaScript can be accomplished through various methods, each with its own advantages. Whether you choose to use innerHTML
, createElement
with appendChild
, or insertAdjacentHTML
, understanding these techniques will enhance your ability to create dynamic web applications. By mastering these methods, you can ensure a better user experience and more efficient code. As you continue to develop your skills, remember to consider performance and security implications, especially when dealing with dynamic content.
FAQ
-
What is the difference between innerHTML and textContent?
innerHTML allows you to insert HTML content, while textContent only sets or retrieves the text content of an element. -
Is it safe to use innerHTML for user-generated content?
No, using innerHTML with user-generated content can expose your application to XSS attacks. It’s safer to use textContent or createElement. -
Can I use insertAdjacentHTML with plain text?
Yes, you can use insertAdjacentHTML to insert plain text, but it will treat the input as HTML, so be cautious with special characters. -
Which method is best for performance?
Using createElement and appendChild is typically the best choice for performance, especially when adding multiple elements. -
How can I remove an element after appending it?
You can remove an element by selecting it and using the remove() method or by using parentNode.removeChild().
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn