The assert Keyword in Java
-
Using
assert
as a Condition in Java -
Using
assert condition : expression
in Java - to Verify if the Unreachable Part of the Code Is Actually Unreachable
- in Case of Internal Invariants
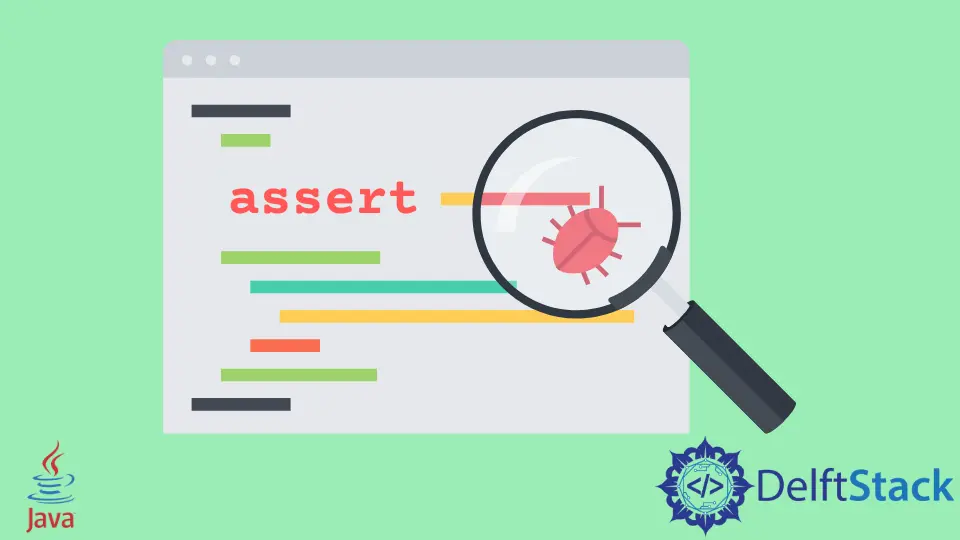
In Java, you can utilize the assert
keyword to enable assertions. This function is used to test our assumptions about the program. There are two ways in which we can use assertions in Java, and we’re going to tackle those in this article.
Using assert
as a Condition in Java
An assertion is the quickest and easiest way to detect and correct bugs in a program. An assertion is assumed to be true when executed. Otherwise, it would throw an assertion error if it’s false.
Here, the assert
condition is a boolean expression.
public class JavaAssertCheck {
public static void main(String args[]) {
String[] names = {"John", "Mary", "David"};
assert names.length == 2;
System.out.println("There are " + names.length + " names in an array");
}
}
Output:
There are 3 names in an array
The assertions are disabled; thus, this program will compile and run without showing any error. But if the assertions are enabled and the condition is false, JVM immediately throws an AssertionError
and stops the program.
Exception in thread
"main" java.lang.AssertionError at JavaAssertCheck.main(JavaAssertCheck.java : 5)
Using assert condition : expression
in Java
In this assertion format, the expression is passed into the constructor of the AssertionError
if the condition is evaluated as false. If the assertions are enabled and the assumption as shown below is false, then the AssertionError
with the passed expression as a detailed message is shown.
This message helps in correctly identifying the error and fixing the error.
public class JavaAssertCheck {
public static void main(String args[]) {
String[] names = {"John", "Mary", "David"};
assert names.length == 2 : "The assertion condition is false.";
System.out.println("There are " + names.length + " names in an array");
}
}
Output:
Exception in thread "main" java.lang.AssertionError: The assertion condition is false.
at JavaAssertCheck.main(JavaAssertCheck.java:5)
Here, it shows certain conditions when we can utilize the concept of assertion in Java.
to Verify if the Unreachable Part of the Code Is Actually Unreachable
public void unReachableCode() {
System.out.println("Unreachable Code");
return;
assert true;
}
If you place an assumption at any location you thought the flow shouldn’t be reached, this function will prompt an error on removing the unreachable statement.
in Case of Internal Invariants
Before assertions were available, many programmers used comments to document their assumptions about the program. As shown below in the multi-way if-statement, we might want to write something explaining our assumption about the else case.
public class JavaAssertCheck {
public static void main(String args[]) {
int n = 37;
if (n % 3 == 0)
System.out.println("n % 3 == 0");
else if (n % 3 == 1)
System.out.println("n % 3 == 1");
else
assert n % 3 == 2 : "Assumption is false. " + n;
}
}
Assertions should not be used in public methods to check the arguments passed because the user can give those themselves; thus, it may fail and lead to an AsssertionError
. Do NOT use assertions to manage the task that your application requires to work correctly. An example is removing null elements from a list.
As a rule, the expression contained in an assertion should be free from side effects.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn