PyQt5 Tutorial - Basic Window
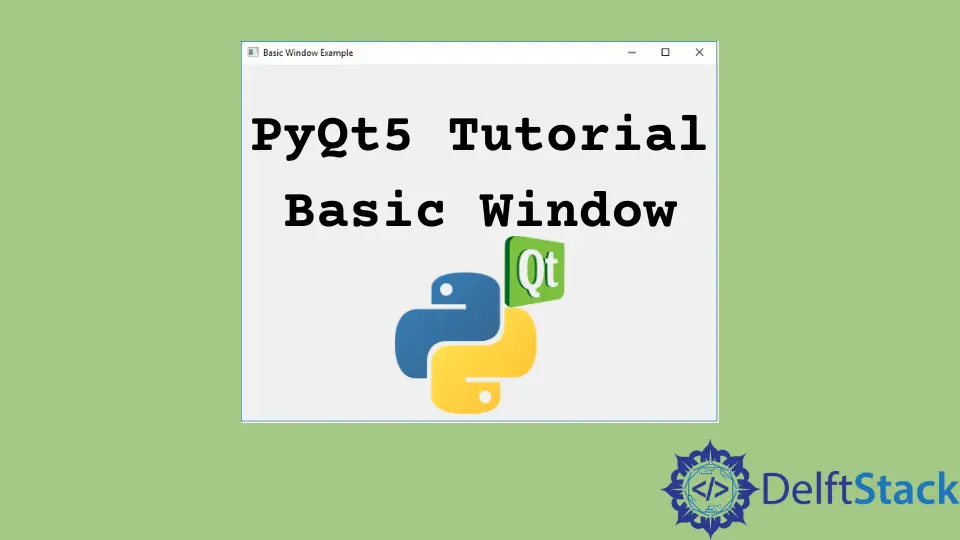
PyQt5 Basic Window
We’re going to create a basic window in PyQt5.
import sys
from PyQt5 import QtWidgets
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
windowExample.setWindowTitle("Basic Window Example")
windowExample.show()
sys.exit(app.exec_())
basicWindow()
from PyQt5 import QtWidgets
It imports the QtWidgets
module so that we have access to the graphical user interface.
app = QtWidgets.QApplication(sys.argv)
It creates an application object that has access to the event loop.
windowExample = QtWidgets.QWidget()
We then need to create a QtWidget
, because we’re going to use that as our top-level window and it has everything that we want.
windowExample.setWindowTitle("Basic Window Example")
setWindowTitle
sets the window a title and it could be called whenever you need it.
windowExample.show()
It is needed to show the window.
sys.exit(app.exec_())
We need to start that event loop by using the app.exec_()
function.
If we don’t do this, the program will run straight through because it won’t keep running back on itself and this event loop here is waiting for events from us to run there.
basicWindow()
Now we’re going to put all of that in a function which could be called to start our window running.
PyQt5 Change Window Size
If we would like to change the size of the window, we could use setGeometry()
method of window widget.
import sys
from PyQt5 import QtWidgets
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
windowExample.setGeometry(0, 0, 400, 400)
windowExample.setWindowTitle("Basic Window Example")
windowExample.show()
sys.exit(app.exec_())
basicWindow()
windowExample.setGeometry(0, 0, 400, 400)
setGeometry()
method takes 4 integers as input argument that are
- X coordinate
- Y coordinate
- Width of the frame
- Height of the frame
Therefore, the example window size is 400 x 400
pixels.
PyQt5 Add Window Icon
import sys
from PyQt5 import QtWidgets, QtGui
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
windowExample.setWindowTitle("Basic Window Example")
windowExample.setWindowIcon(QtGui.QIcon("python.jpg"))
windowExample.show()
sys.exit(app.exec_())
basicWindow()
windowExample.setWindowIcon(QtGui.QIcon("python.jpg"))
It sets the window icon to be python.jpg
. The parameter of setWindowIcon
method is QIcon
object from QtGui
module.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook