JavaScript Tutorial - Variables
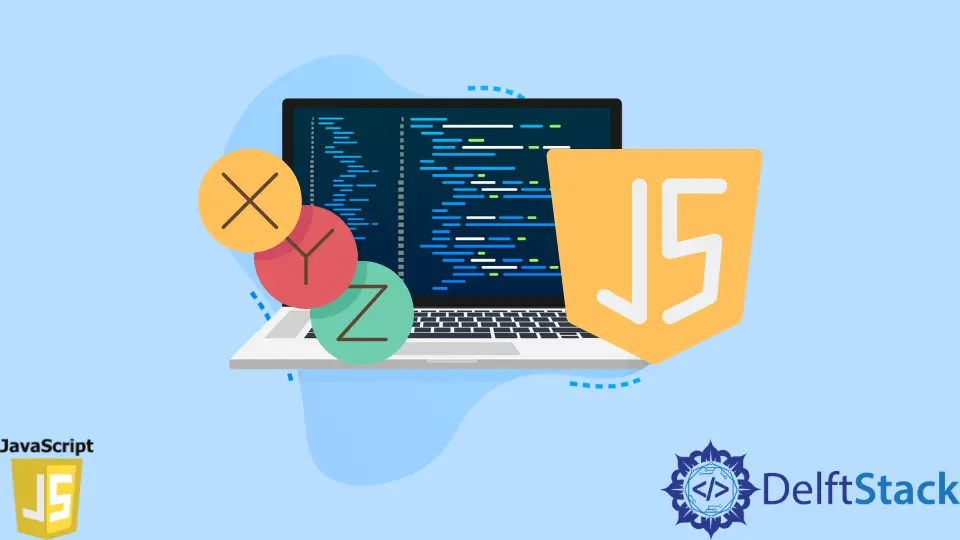
We will learn JavaScript variables in this tutorial.
Variable Definition
A variable is used in the program to store information. It could be the age of the visitor, or the name of the user. The variable stores the information and could be used again later on.
You could name your variable as almost anything you want in JavaScript, but there are a few rules:
Variable Identifier Rules
- The variable is allowed to consist of letters, numbers, and underscores, but it has to begin with either a lowercase letter or an uppercase letter or an underscore, and it can’t start with the number.
- You’re not allowed to use any JavaScript keywords.
- Variable name is case-sensitive.
- A variable name cannot contain spaces.
Declare a Variable
Syntax
var <variable - name>;
// or
var <variable - name> = <value>;
JavaScript Variable Declaration Example
var x = 1;
You need to declare a variable before you can use it. You use the keyword var
and then variable name, like x
in the above example. You could initialize the value of the variable together with the declaration or you could assign a value to it later.
JavaScript has dynamic variable data types and you could assign the variable with different data types without any problem.
It is not like other programming languages that you have to specify the type of a variable when you declare it and the type couldn’t be changed afterword.
JavaScript Multiple Variables Declaration
Multiple variables could be declared in one single line separated by the comma ,
.
var one, two, three = 1, 2, 3;
JavaScript Variable Declaration Without var
We could declare a variable in JavaScript without var
, but need to assign the value directly.
one = 1;
two = 2;
three, four = 3, 4;
let
and const
Variables in ES6
The var
keyword declares the variables in the scope of the function, but let
and const
keywords introduced in ES6 extend the variable scope to the block level {}
.
The variable declared with const
keyword couldn’t be reassigned after declaration, but the variable declared with let
could.
> let A = '1' > A = '2'
'2' > const B = 1;
> B = 2
VM944: 1 Uncaught TypeError: Assignment to constant variable.at<anonymous>: 1: 3
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook