How to Sort a Python Dictionary by Value
- Python Sort Dictionary by Value - Only Get the Sorted Values
-
Use
operator.itemgetter
to Sort the Python Dictionary -
Use
lambda
Function in the Key ofsorted
to Sort Python the Dictionary -
OrderedDict
to Get the Dictionary-Compatible Result in Python Dictionary Sorting
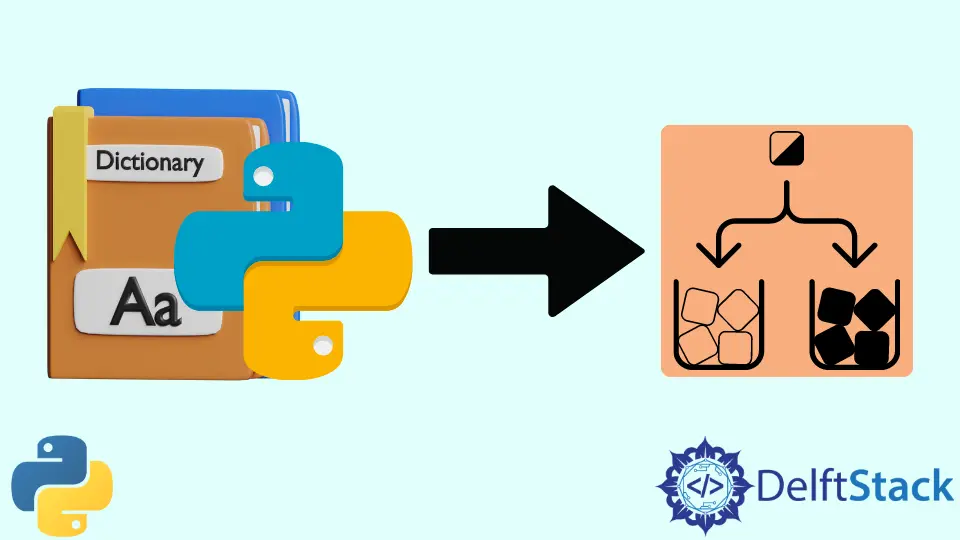
Python dictionary is an order-less data type, therefore, you could not sort the Python dictionary by its keys or values. But you could get the representation of sorted Python dictionary in other data types like list.
Assume we have a dictionary like below,
exampleDict = {"first": 3, "second": 4, "third": 2, "fourth": 1}
Python Sort Dictionary by Value - Only Get the Sorted Values
sortedDict = sorted(exampleDict.values())
# Out: [1, 2, 3, 4]
Use operator.itemgetter
to Sort the Python Dictionary
import operator
sortedDict = sorted(exampleDict.items(), key=operator.itemgetter(1))
# Out: [('fourth', 1), ('third', 2), ('first', 3), ('second', 4)]
exampleDict.items
returns the key-value pair of dictionary elements. key=operator.itemgetter(1)
specifies the comparison key is the value of the dictionary, meanwhile operator.itemgetter(0)
has the comparison key of the dictionary key.
Use lambda
Function in the Key of sorted
to Sort Python the Dictionary
You could also lambda
function to get the comparison key instead of operator.itemgetter
sortedDict = sorted(exampleDict.items(), key=lambda x: x[1])
# Out: [('fourth', 1), ('third', 2), ('first', 3), ('second', 4)]
exampleDict.items()
returns a list of key-value pairs of the dictionary and its element’s data type is tuple. x
is the element of this tuple, where x[0]
is the key and x[1]
is the value. key=lambda x:x[1]
indicates the comparison key is the value of the dictionary elements.
Optional parameter reverse
could be set to be True
if the values need to be sorted in descending order.
sortedDict = sorted(exampleDict.items(), key=lambda x: x[1], reverse=True)
# Out: [('second', 4), ('first', 3), ('third', 2), ('fourth', 1)]
OrderedDict
to Get the Dictionary-Compatible Result in Python Dictionary Sorting
The example code shown above returns the result as a list, but not a dictionary type. If you want to keep the result as dictionary-compatible type, OrderedDict
introduced from Python 2.7 is the right choice.
from collections import OrderedDict
sortedDict = OrderedDict(sorted(exampleDict.items(), key=lambda x: x[1]))
# Out: OrderedDict([('fourth', 1), ('third', 2), ('first', 3), ('second', 4)])
The OrderedDict
is a Python dict
subclass that supports the usual methods and also remembers the order that keys are first inserted.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook