Pandas는 문자열을 숫자 형으로 변환
Suraj Joshi
2023년1월30일
Pandas
Pandas Data Type
-
pandas.to_numeric()
메서드 -
pandas.to_numeric()
메서드를 사용하여 Pandas DataFrame의 문자열 값을 숫자 유형으로 변환 - Pandas DataFrame의 문자열 값을 다른 문자가 포함 된 숫자 유형으로 변환
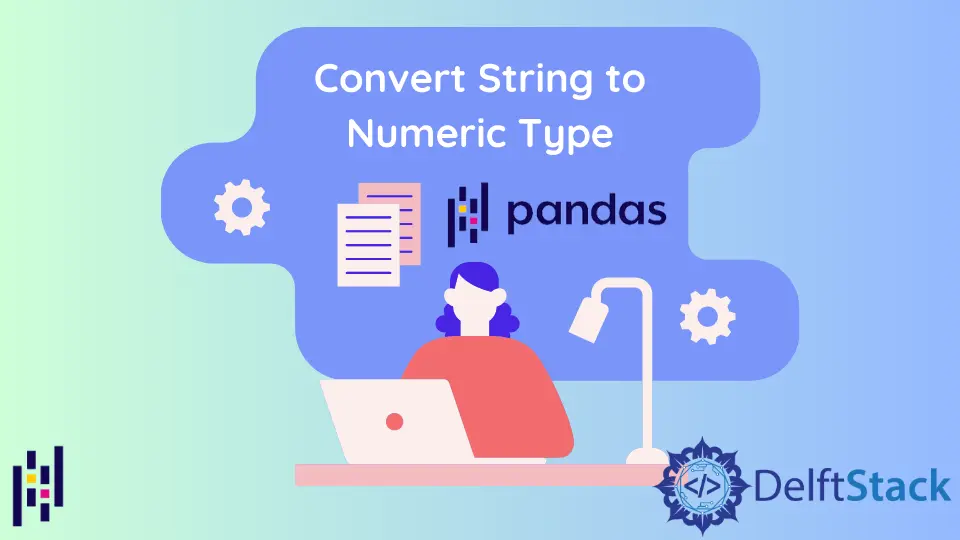
이 튜토리얼에서는pandas.to_numeric()
메소드를 사용하여 Pandas DataFrame의 문자열 값을 숫자 유형으로 변환하는 방법을 설명합니다.
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": ["300", "400", "350", "100", "1000", "400"],
}
)
print(items_df)
출력:
Id Name Cost
0 302 Watch 300
1 504 Camera 400
2 708 Phone 350
3 103 Shoes 100
4 343 Laptop 1000
5 565 Bed 400
위의 예제를 사용하여 DataFrame의 값을 숫자 유형으로 변경하는 방법을 보여줍니다.
pandas.to_numeric()
메서드
통사론
pandas.to_numeric(arg, errors="raise", downcast=None)
arg
로 전달 된 인수를 숫자 유형으로 변환합니다. 기본적으로arg
는int64
또는float64
로 변환됩니다. downcast
매개 변수의 값을 설정하여arg
를 다른 데이터 유형으로 변환 할 수 있습니다.
pandas.to_numeric()
메서드를 사용하여 Pandas DataFrame의 문자열 값을 숫자 유형으로 변환
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": ["300", "400", "350", "100", "1000", "400"],
}
)
print("The items DataFrame is:")
print(items_df, "\n")
print("Datatype of Cost column before type conversion:")
print(items_df["Cost"].dtypes, "\n")
items_df["Cost"] = pd.to_numeric(items_df["Cost"])
print("Datatype of Cost column after type conversion:")
print(items_df["Cost"].dtypes)
출력:
The items DataFrame is:
Id Name Cost
0 302 Watch 300
1 504 Camera 400
2 708 Phone 350
3 103 Shoes 100
4 343 Laptop 1000
5 565 Bed 400
Datatype of Cost column before type conversion:
object
Datatype of Cost column after type conversion:
int64
items_df
의Cost
열의 데이터 유형을object
에서int64
로 변환합니다.
Pandas DataFrame의 문자열 값을 다른 문자가 포함 된 숫자 유형으로 변환
열을 일부 문자가 포함 된 값이있는 숫자 유형으로 변환하려는 경우 ValueError: Unable to parse string
이라는 오류가 발생합니다. 이러한 경우 숫자가 아닌 모든 문자를 제거한 다음 유형 변환을 수행 할 수 있습니다.
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": ["$300", "$400", "$350", "$100", "$1000", "$400"],
}
)
print("The items DataFrame is:")
print(items_df, "\n")
print("Datatype of Cost column before type conversion:")
print(items_df["Cost"].dtypes, "\n")
items_df["Cost"] = pd.to_numeric(items_df["Cost"].str.replace("$", ""))
print("Datatype of Cost column after type conversion:")
print(items_df["Cost"].dtypes, "\n")
print("DataFrame after Type Conversion:")
print(items_df)
출력:
The items DataFrame is:
Id Name Cost
0 302 Watch $300
1 504 Camera $400
2 708 Phone $350
3 103 Shoes $100
4 343 Laptop $1000
5 565 Bed $400
Datatype of Cost column before type conversion:
object
Datatype of Cost column after type conversion:
int64
DataFrame after Type Conversion:
Id Name Cost
0 302 Watch 300
1 504 Camera 400
2 708 Phone 350
3 103 Shoes 100
4 343 Laptop 1000
5 565 Bed 400
Cost
열의 값에 첨부 된 $
문자를 제거한 다음 pandas.to_numeric()
메서드를 사용하여 이러한 값을 숫자 유형으로 변환합니다.
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다
작가: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn