Pandas は文字列を数値型に変換する
Suraj Joshi
2023年1月30日
-
pandas.to_numeric()
メソッド -
pandas.to_numeric()
メソッドを用いて Pandas DataFrame の文字列値を数値型に変換する - Pandas DataFrame の文字列の値を他の文字を含む数値型に変換する
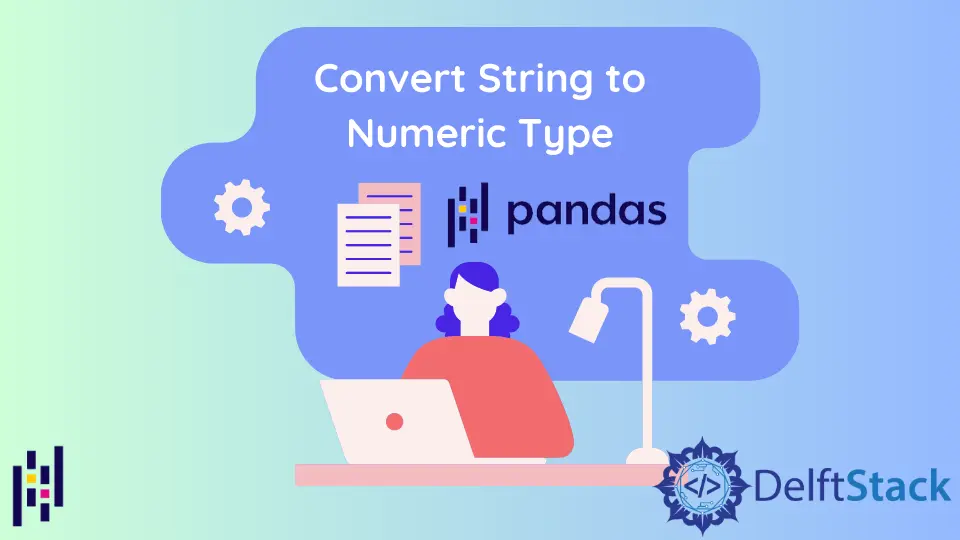
このチュートリアルでは、pandas.to_numeric()
メソッドを使って Pandas DataFrame の文字列値を数値型に変換する方法を説明します。
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": ["300", "400", "350", "100", "1000", "400"],
}
)
print(items_df)
出力:
Id Name Cost
0 302 Watch 300
1 504 Camera 400
2 708 Phone 350
3 103 Shoes 100
4 343 Laptop 1000
5 565 Bed 400
上記の例を使って、DataFrame の値を数値型に変換する方法を説明します。
pandas.to_numeric()
メソッド
構文
pandas.to_numeric(arg, errors="raise", downcast=None)
引数 arg
に渡された引数を数値型に変換します。デフォルトでは、arg
は int64
または float64
に変換されています。downcast
パラメータに値を設定することで、arg
を他のデータ型に変換することができます。
pandas.to_numeric()
メソッドを用いて Pandas DataFrame の文字列値を数値型に変換する
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": ["300", "400", "350", "100", "1000", "400"],
}
)
print("The items DataFrame is:")
print(items_df, "\n")
print("Datatype of Cost column before type conversion:")
print(items_df["Cost"].dtypes, "\n")
items_df["Cost"] = pd.to_numeric(items_df["Cost"])
print("Datatype of Cost column after type conversion:")
print(items_df["Cost"].dtypes)
出力:
The items DataFrame is:
Id Name Cost
0 302 Watch 300
1 504 Camera 400
2 708 Phone 350
3 103 Shoes 100
4 343 Laptop 1000
5 565 Bed 400
Datatype of Cost column before type conversion:
object
Datatype of Cost column after type conversion:
int64
これは items_df
の Cost
カラムのデータ型を object
から int64
に変換します。
Pandas DataFrame の文字列の値を他の文字を含む数値型に変換する
ある文字を含む値を持つ数値型のカラムを変換したい場合、ValueError: Unable to parse string
というエラーが発生します。このような場合は、数値以外の文字をすべて削除してから、型変換を実行できます。
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": ["$300", "$400", "$350", "$100", "$1000", "$400"],
}
)
print("The items DataFrame is:")
print(items_df, "\n")
print("Datatype of Cost column before type conversion:")
print(items_df["Cost"].dtypes, "\n")
items_df["Cost"] = pd.to_numeric(items_df["Cost"].str.replace("$", ""))
print("Datatype of Cost column after type conversion:")
print(items_df["Cost"].dtypes, "\n")
print("DataFrame after Type Conversion:")
print(items_df)
出力:
The items DataFrame is:
Id Name Cost
0 302 Watch $300
1 504 Camera $400
2 708 Phone $350
3 103 Shoes $100
4 343 Laptop $1000
5 565 Bed $400
Datatype of Cost column before type conversion:
object
Datatype of Cost column after type conversion:
int64
DataFrame after Type Conversion:
Id Name Cost
0 302 Watch 300
1 504 Camera 400
2 708 Phone 350
3 103 Shoes 100
4 343 Laptop 1000
5 565 Bed 400
Cost
カラムの値に添付されている $
文字を削除し、pandas.to_numeric()
メソッドを用いて数値型に変換します。
著者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn