Pandas 将字符串转换为数字类型
Suraj Joshi
2023年1月30日
Pandas
Pandas Data Type
-
pandas.to_numeric()
方法 -
使用
pandas.to_numeric()
方法将 Pandas DataFrame 的字符串值转换为数字类型 - 将 Pandas DataFrame 中的字符串值转换为含有其他字符的数字类型
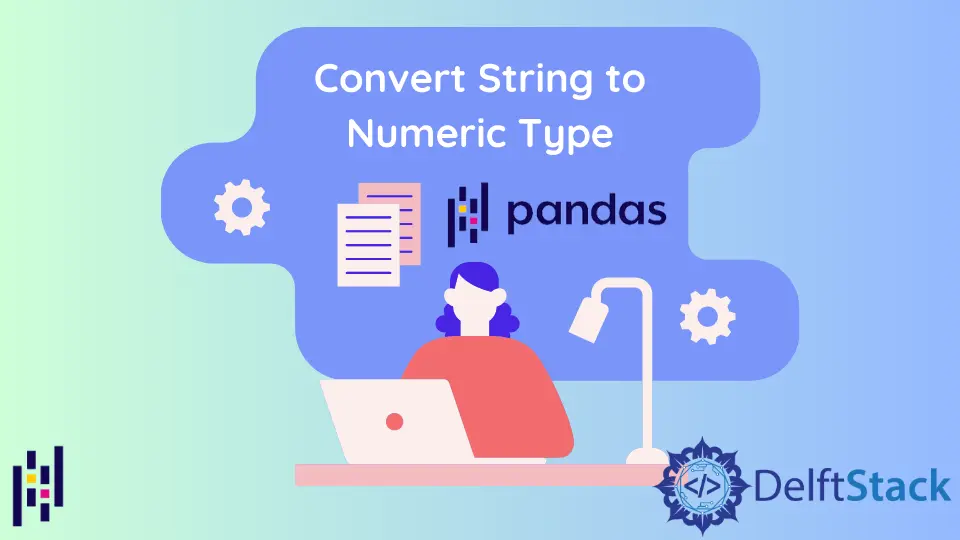
本教程解释了如何使用 pandas.to_numeric()
方法将 Pandas DataFrame 的字符串值转换为数字类型。
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": ["300", "400", "350", "100", "1000", "400"],
}
)
print(items_df)
输出:
Id Name Cost
0 302 Watch 300
1 504 Camera 400
2 708 Phone 350
3 103 Shoes 100
4 343 Laptop 1000
5 565 Bed 400
我们将用上面的例子来演示如何将 DataFrame 的值转换为数字类型。
pandas.to_numeric()
方法
语法
pandas.to_numeric(arg, errors="raise", downcast=None)
它将作为 arg
传递的参数转换为数字类型。默认情况下,arg
将被转换为 int64
或 float64
。我们可以设置 downcast
参数的值,将 arg
转换为其他数据类型。
使用 pandas.to_numeric()
方法将 Pandas DataFrame 的字符串值转换为数字类型
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": ["300", "400", "350", "100", "1000", "400"],
}
)
print("The items DataFrame is:")
print(items_df, "\n")
print("Datatype of Cost column before type conversion:")
print(items_df["Cost"].dtypes, "\n")
items_df["Cost"] = pd.to_numeric(items_df["Cost"])
print("Datatype of Cost column after type conversion:")
print(items_df["Cost"].dtypes)
输出:
The items DataFrame is:
Id Name Cost
0 302 Watch 300
1 504 Camera 400
2 708 Phone 350
3 103 Shoes 100
4 343 Laptop 1000
5 565 Bed 400
Datatype of Cost column before type conversion:
object
Datatype of Cost column after type conversion:
int64
它将 items_df
中 Cost
列的数据类型从 object
转换为 int64
。
将 Pandas DataFrame 中的字符串值转换为含有其他字符的数字类型
如果我们想将一列转换成数值类型,其中有一些字符的值,我们得到一个错误说 ValueError: Unable to parse string
。在这种情况下,我们可以删除所有非数字字符,然后进行类型转换。
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708, 103, 343, 565],
"Name": ["Watch", "Camera", "Phone", "Shoes", "Laptop", "Bed"],
"Cost": ["$300", "$400", "$350", "$100", "$1000", "$400"],
}
)
print("The items DataFrame is:")
print(items_df, "\n")
print("Datatype of Cost column before type conversion:")
print(items_df["Cost"].dtypes, "\n")
items_df["Cost"] = pd.to_numeric(items_df["Cost"].str.replace("$", ""))
print("Datatype of Cost column after type conversion:")
print(items_df["Cost"].dtypes, "\n")
print("DataFrame after Type Conversion:")
print(items_df)
输出:
The items DataFrame is:
Id Name Cost
0 302 Watch $300
1 504 Camera $400
2 708 Phone $350
3 103 Shoes $100
4 343 Laptop $1000
5 565 Bed $400
Datatype of Cost column before type conversion:
object
Datatype of Cost column after type conversion:
int64
DataFrame after Type Conversion:
Id Name Cost
0 302 Watch 300
1 504 Camera 400
2 708 Phone 350
3 103 Shoes 100
4 343 Laptop 1000
5 565 Bed 400
它删除了与 Cost
列的值相连的 $
字符,然后使用 pandas.to_numeric()
方法将这些值转换为数字类型。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn