如何在 Pandas 中更改列的数据类型
Asad Riaz
2023年1月30日
Pandas
Pandas Data Type
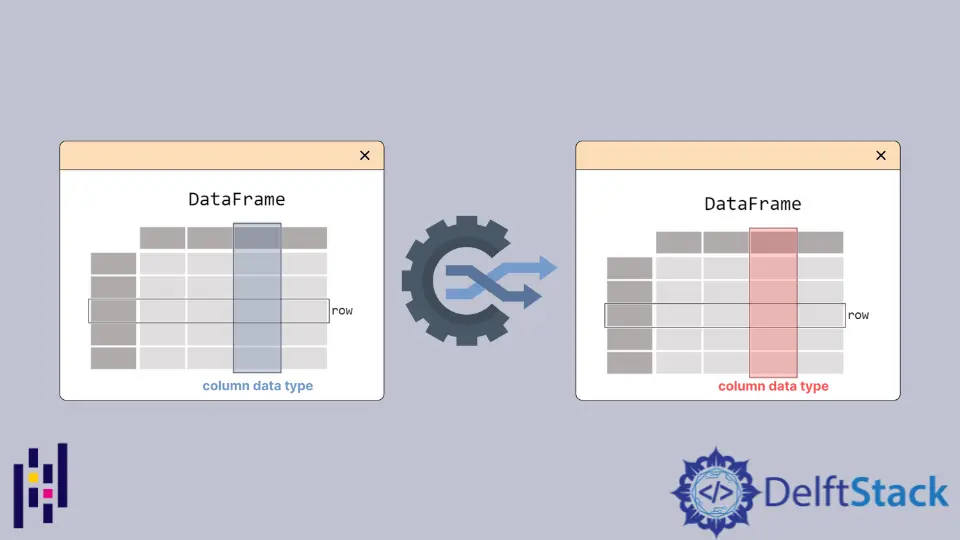
我们将介绍更改 Pandas Dataframe 中列数据类型的方法,以及 to_numaric
、as_type
和 infer_objects
等选项。我们还将讨论如何在 to_numaric
中使用 downcasting
选项。
to_numeric
方法将列转换为 Pandas 中的数值
to_numeric()
是将 dataFrame 的一列或多列转换为数值的最佳方法。它还会尝试将非数字对象(例如字符串)适当地更改为整数或浮点数。to_numeric()
输入可以是 Series
或 DataFrame
的列。如果某些值不能转换为数字类型,则 to_numeric()
允许我们将非数字值强制为 NaN。
代码举例:
# python 3.x
import pandas as pd
s = pd.Series(["12", "12", "4.7", "asad", "3.0"])
print(s)
print("------------------------------")
print(pd.to_numeric(s, errors="coerce"))
输出:
0 12
1 12
2 4.7
3 asad
4 3.0
dtype: object0 12.0
1 12.0
2 4.7
3 NaN
4 3.0
dtype: float64
默认情况下,to_numeric()
将为我们提供 int64
或 float64
dtype。我们可以使用一个选项来转换为 integer
,signed
,unsigned
或者 float
:
# python 3.x
import pandas as pd
s = pd.Series([-3, 1, -5])
print(s)
print(pd.to_numeric(s, downcast="integer"))
输出:
0 -3
1 1
2 -5
dtype: int64
0 -3
1 1
2 -5
dtype: int8
astype()
方法将一种类型转换为任何其他数据类型
astype()
方法使我们能够明确了解要转换的 dtype。通过在 astype()
方法内传递参数,我们可以从一种数据类型转到另一种数据类型。
考虑以下代码:
# python 3.x
import pandas as pd
c = [["x", "1.23", "14.2"], ["y", "20", "0.11"], ["z", "3", "10"]]
df = pd.DataFrame(c, columns=["first", "second", "third"])
print(df)
df[["second", "third"]] = df[["second", "third"]].astype(float)
print("Converting..................")
print("............................")
print(df)
输出:
first second third
0 x 1.23 14.2
1 y 20 0.11
2 z 3 10
Converting..................
............................
first second third
0 x 1.23 14.20
1 y 20.00 0.11
2 z 3.00 10.00
infer_objects()
方法将列数据类型转换为更特定的类型
从 Pandas 的 0.21.0 版本开始引入的 infer_objects()
方法,用于将 dataFrame
的列转换为更特定的数据类型(软转换)。
考虑以下代码:
# python 3.x
import pandas as pd
df = pd.DataFrame({"a": [3, 12, 5], "b": [3.0, 2.6, 1.1]}, dtype="object")
print(df.dtypes)
df = df.infer_objects()
print("Infering..................")
print("............................")
print(df.dtypes)
输出:
a object
b object
dtype: object
Infering..................
............................
a int64
b float64
dtype: object
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe