如何在 Pandas 中更改列的資料型別
Asad Riaz
2023年1月30日
Pandas
Pandas Data Type
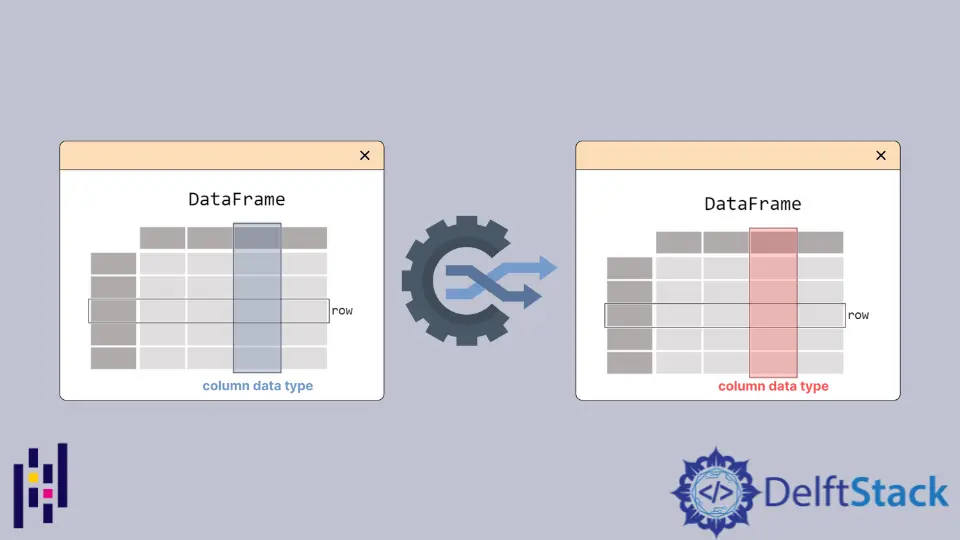
我們將介紹更改 Pandas Dataframe 中列資料型別的方法,以及 to_numaric
、as_type
和 infer_objects
等選項。我們還將討論如何在 to_numaric
中使用 downcasting
選項。
to_numeric
方法將列轉換為 Pandas 中的數值
to_numeric()
是將 dataFrame 的一列或多列轉換為數值的最佳方法。它還會嘗試將非數字物件(例如字串)適當地更改為整數或浮點數。to_numeric()
輸入可以是 Series
或 DataFrame
的列。如果某些值不能轉換為數字型別,則 to_numeric()
允許我們將非數字值強制為 NaN。
程式碼舉例:
# python 3.x
import pandas as pd
s = pd.Series(["12", "12", "4.7", "asad", "3.0"])
print(s)
print("------------------------------")
print(pd.to_numeric(s, errors="coerce"))
輸出:
0 12
1 12
2 4.7
3 asad
4 3.0
dtype: object0 12.0
1 12.0
2 4.7
3 NaN
4 3.0
dtype: float64
預設情況下,to_numeric()
將為我們提供 int64
或 float64
dtype。我們可以使用一個選項來轉換為 integer
,signed
,unsigned
或者 float
:
# python 3.x
import pandas as pd
s = pd.Series([-3, 1, -5])
print(s)
print(pd.to_numeric(s, downcast="integer"))
輸出:
0 -3
1 1
2 -5
dtype: int64
0 -3
1 1
2 -5
dtype: int8
astype()
方法將一種型別轉換為任何其他資料型別
astype()
方法使我們能夠明確瞭解要轉換的 dtype。通過在 astype()
方法內傳遞引數,我們可以從一種資料型別轉到另一種資料型別。
考慮以下程式碼:
# python 3.x
import pandas as pd
c = [["x", "1.23", "14.2"], ["y", "20", "0.11"], ["z", "3", "10"]]
df = pd.DataFrame(c, columns=["first", "second", "third"])
print(df)
df[["second", "third"]] = df[["second", "third"]].astype(float)
print("Converting..................")
print("............................")
print(df)
輸出:
first second third
0 x 1.23 14.2
1 y 20 0.11
2 z 3 10
Converting..................
............................
first second third
0 x 1.23 14.20
1 y 20.00 0.11
2 z 3.00 10.00
infer_objects()
方法將列資料型別轉換為更特定的型別
從 Pandas 的 0.21.0 版本開始引入的 infer_objects()
方法,用於將 dataFrame
的列轉換為更特定的資料型別(軟轉換)。
考慮以下程式碼:
# python 3.x
import pandas as pd
df = pd.DataFrame({"a": [3, 12, 5], "b": [3.0, 2.6, 1.1]}, dtype="object")
print(df.dtypes)
df = df.infer_objects()
print("Infering..................")
print("............................")
print(df.dtypes)
輸出:
a object
b object
dtype: object
Infering..................
............................
a int64
b float64
dtype: object
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe