C#에서 목록 길이 가져오기
Fil Zjazel Romaeus Villegas
2023년10월12일
Csharp
Csharp List
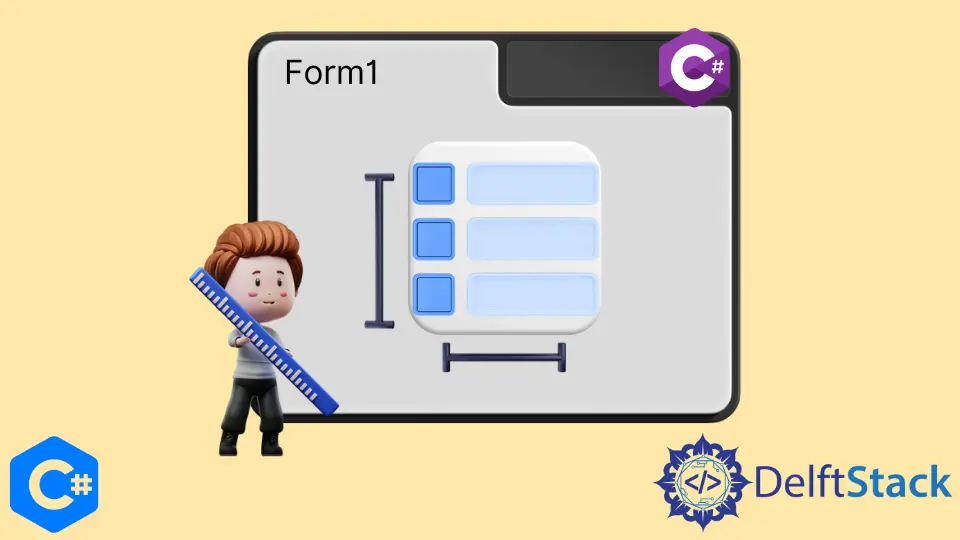
이 자습서에서는 count 함수를 사용하여 C# 목록의 길이를 가져오는 방법을 보여줍니다.
목록은 해당 인덱스에서 값에 액세스할 수 있는 지정된 유형의 개체 컬렉션 유형입니다.
List<T> myList = new List<T>();
추가하는 값의 유형이 초기화 중에 정의된 것과 일치하는 한 목록에 원하는 만큼 항목을 추가할 수 있습니다.
C# 목록에는 목록의 항목 수를 반환하는 내장 함수 Count
가 있습니다.
int list_count = myList.Count;
예시:
using System;
using System.Collections.Generic;
namespace ListCount_Example {
class Program {
static void Main(string[] args) {
// Initializing the list of strings
List<string> student_list = new List<string>();
// Adding values to the list
student_list.Add("Anna Bower");
student_list.Add("Ian Mitchell");
student_list.Add("Dorothy Newman");
student_list.Add("Nathan Miller");
student_list.Add("Andrew Dowd");
student_list.Add("Gavin Davies");
student_list.Add("Alexandra Berry");
// Getting the count of items in the list and storing it in the student_count variable
int student_count = student_list.Count;
// Printing the result
Console.WriteLine("Total Number of students: " + student_count.ToString());
}
}
}
위의 예에서 문자열 목록이 초기화되고 7개의 레코드가 추가됩니다. 레코드를 추가한 후 count 함수를 사용하여 레코드 수를 가져와 정수 값으로 저장한 다음 인쇄합니다.
출력:
Total Number of students: 7
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다