C# でリストの長さを取得する
Fil Zjazel Romaeus Villegas
2023年10月12日
Csharp
Csharp List
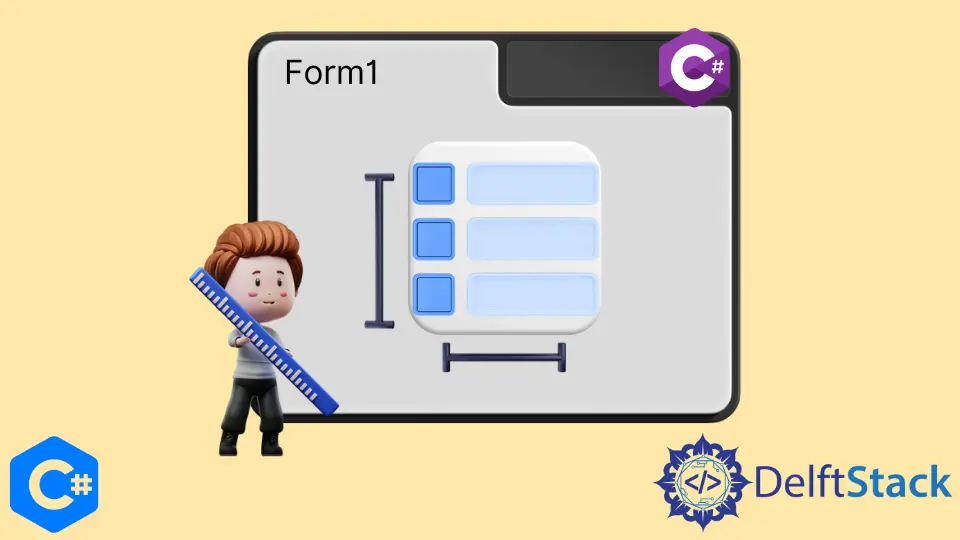
このチュートリアルでは Count
関数を使用して C# リストの長さを取得する方法を示します。
リストは、指定されたタイプのオブジェクトのコレクションのタイプであり、そのインデックスから値にアクセスできます。
List<T> myList = new List<T>();
追加する値のタイプが初期化中に定義されたものと一致する限り、リストに必要な数のアイテムを追加できます。
C# リストには、リスト内のアイテムの数を返す組み込み関数 Count
があります。
int list_count = myList.Count;
例:
using System;
using System.Collections.Generic;
namespace ListCount_Example {
class Program {
static void Main(string[] args) {
// Initializing the list of strings
List<string> student_list = new List<string>();
// Adding values to the list
student_list.Add("Anna Bower");
student_list.Add("Ian Mitchell");
student_list.Add("Dorothy Newman");
student_list.Add("Nathan Miller");
student_list.Add("Andrew Dowd");
student_list.Add("Gavin Davies");
student_list.Add("Alexandra Berry");
// Getting the count of items in the list and storing it in the student_count variable
int student_count = student_list.Count;
// Printing the result
Console.WriteLine("Total Number of students: " + student_count.ToString());
}
}
}
上記の例では、文字列のリストが初期化され、7つのレコードが追加されています。レコードを追加した後、カウント機能を使用してレコード数が取得され、整数値に格納されてから出力されます。
出力:
Total Number of students: 7
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe