C++에서 문자열을 소문자로 변환하는 방법
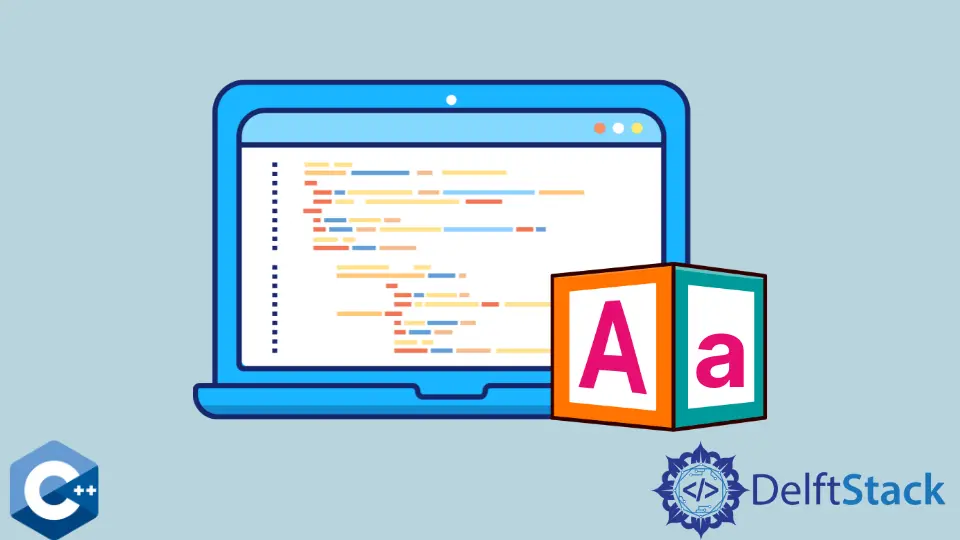
이 기사에서는 C++에서 문자열을 소문자로 변환하는 방법을 소개하겠다.
C++에서 문자열 변환을 수행하기 전에 먼저 자신에게 물어봐야 할 것은 내 입력 문자열의 인코딩 종류는 무엇인가? 왜냐하면 만약 당신이 멀티바이트 인코딩 문자에 std::lower를 사용한다면, 당신은 분명히 버기 코드를 얻게 될 것이기 때문이다.
std::string
소문자 변환을 깔끔하게 구현한 것처럼 보이지만 인코딩이 UTF-8이기 때문에 모든 문자를 소문자로 변환하지는 않는다.
#include <algorithm>
#include <iostream>
std::string toLower(std::string s) {
std::transform(s.begin(), s.end(), s.begin(),
[](unsigned char c) { return std::tolower(c); });
return s;
}
int main() {
std::string string1 = u8"ÅSH to LoWer WÅN";
std::cout << "input string: " << string1 << std::endl
<< "output string: " << toLower(string1) << std::endl;
return 0;
}
위의 코드는 ASCII 문자열과 다른 비 ASCII 문자열에도 잘 작동하지만, 일단 좀 특이한 입력을 하면, 라틴어 기호를 입력하면 출력이 만족스럽지 않을 것이다.
출력:
input string: ÅSH to LoWer WÅN
output string: Åsh to lower wÅn
Å
기호를å
로 낮춰야하므로 잘못되었습니다. 그렇다면 올바른 출력을 얻기 위해이 문제를 어떻게 해결할 수 있습니까?
이 작업을 수행하는 가장 좋은 이식 방법은 안정성을 제공하고 광범위하게 액세스 할 수있을만큼 성숙하고 코드를 교차 플랫폼에 유지하는ICU
(International Components for Unicode) 라이브러리를 사용하는 것입니다.
우리는 다음의 헤더만 우리의 소스 파일에 포함시키면 된다. 이 도서관들은 이미 당신의 플랫폼에 포함되어 있고 이용할 수 있기 때문에 코드 샘플은 잘 작동될 것이다. 그러나 IDE/컴파일 시간 오류가 발생하면 ICU 설명서 웹 사이트)에서 라이브러리를 다운로드하는 지침을 참조하십시오.
#include <unicode/locid.h>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
헤더를 포함했으므로, 다음과 같이 소문자 변환 코드에 std::string
을 쓸 수 있다.
#include <unicode/locid.h>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
#include <iostream>
int main() {
std::string string1 = u8"ÅSH to LoWer WÅN";
icu::UnicodeString unicodeString(string1.c_str());
std::cout << "input string: " << string1 << std::endl
<< "output string: " << unicodeString.toLower() << std::endl;
return 0;
}
ICU 라이브러리 종속성을 포함하도록 다음과 같은 컴파일러 플래그를 사용하여 이 코드를 컴파일해야 한다는 점에 유의하십시오.
g++ sample_code.cpp -licuio -licuuc -o sample_code
코드를 실행하면 예상대로 정확한 출력을 얻을 수 있다.
input string: ÅSH to LoWer WÅN
output string: åsh to lower wån
동일한 함수는 일반적으로 사용자 입력으로 기대하지 않는 일부 다른 언어를 처리할 수 있으며 로케일을 toLower
함수의 매개 변수로 명시적으로 지정할 수도 있다.
#include <iostream>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
#include <unicode/locid.h>
int main() {
std::string string2 = "Κάδμῳ ἀπιϰόμενοι.. Ελληνας ϰαὶ δὴ ϰαὶ γράμματα, οὐϰ ἐόντα πρὶν Ελλησι";
icu::UnicodeString unicodeString2(string2.c_str());
std::cout << unicodeString2.toLower("el_GR") << std::endl;
return 0;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook