如何在 C++ 中把字符串转换为小写
Jinku Hu
2023年12月11日
C++
C++ String
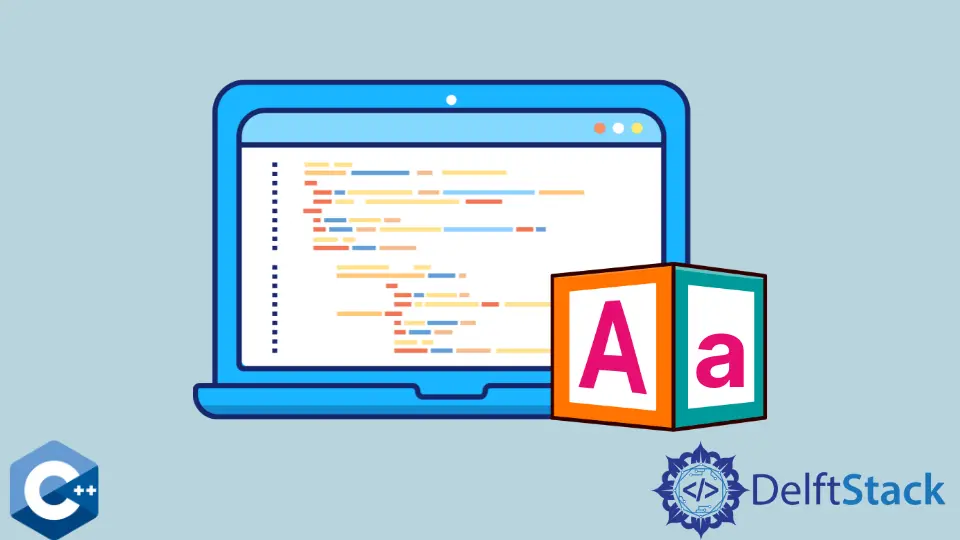
在本文中,我们将来介绍在 C++ 中如何将字符串进行小写转换。
当我们在考虑 C++ 中的字符串转换方法时,首先要问自己的是我的输入字符串有什么样的编码?因为如果你将使用 std::lower
与多字节编码字符,那么你一定会得到错误的代码。
即使下面的函数看起来是对 std::string
小写转换的整洁实现,当你期望输入的是 UTF-8
编码字符时,它不会将所有的字符转换为小写。
#include <algorithm>
#include <iostream>
std::string toLower(std::string s) {
std::transform(s.begin(), s.end(), s.begin(),
[](unsigned char c) { return std::tolower(c); });
return s;
}
int main() {
std::string string1 = u8"ÅSH to LoWer WÅN";
std::cout << "input string: " << string1 << std::endl
<< "output string: " << toLower(string1) << std::endl;
return 0;
}
上面的代码对于 ASCII 字符串和其他一些非 ASCII 字符串也能很好的工作,但是一旦你给它一个有点不寻常的输入,比如说里面有一些拉丁符号,输出就会不尽人意。
输出:
input string: ÅSH to LoWer WÅN
output string: Åsh to lower wÅn
它是不正确的,因为它应该把 Å
改为小写的 å
。那么,我们怎样才能解决这个问题,得到正确的输出呢?
最好的可移植方式是使用 ICU
(International Components for Unicode,国际统一码组件)库,它足够成熟,提供了稳定性,广泛的可访问性,并将保持你的代码跨平台。
我们只需要在源文件中包含以下头文件。这些库很有可能已经包含在你的平台上,并且在你的平台上可用,所以代码样本应该可以正常工作。但如果你在 IDE/编译时出现错误,请参考 ICU 文档网站中的库下载说明。
#include <unicode/locid.h>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
现在我们已经包含了头文件,所以我们可以写 std::string
到小写的转换代码如下。
#include <unicode/locid.h>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
#include <iostream>
int main() {
std::string string1 = u8"ÅSH to LoWer WÅN";
icu::UnicodeString unicodeString(string1.c_str());
std::cout << "input string: " << string1 << std::endl
<< "output string: " << unicodeString.toLower() << std::endl;
return 0;
}
注意,我们在编译这段代码时,应该使用以下编译器标志,以包含 ICU 库的依赖性。
g++ sample_code.cpp -licuio -licuuc -o sample_code
运行代码,我们将按预期获得正确的输出:
input string: ÅSH to LoWer WÅN
output string: åsh to lower wån
完全相同的函数可以处理一些我们通常不希望作为用户输入的语言,并且我们还可以显式地将语言环境指定为 toLower
函数的参数:
#include <iostream>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
#include <unicode/locid.h>
int main() {
std::string string2 = "Κάδμῳ ἀπιϰόμενοι.. Ελληνας ϰαὶ δὴ ϰαὶ γράμματα, οὐϰ ἐόντα πρὶν Ελλησι";
icu::UnicodeString unicodeString2(string2.c_str());
std::cout << unicodeString2.toLower("el_GR") << std::endl;
return 0;
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu