如何在 C++ 中把字串轉換為小寫
Jinku Hu
2023年12月11日
C++
C++ String
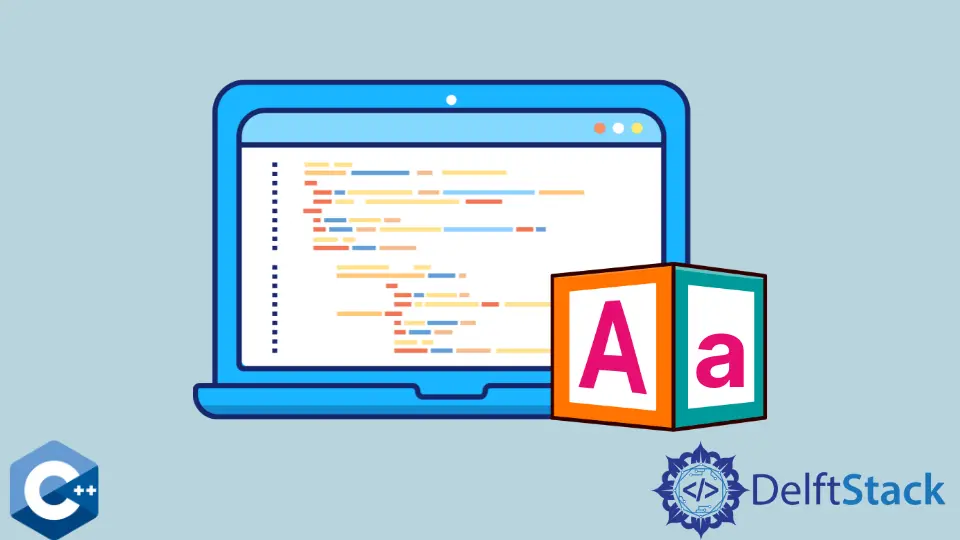
在本文中,我們將來介紹在 C++ 中如何將字串進行小寫轉換。
當我們在考慮 C++ 中的字串轉換方法時,首先要問自己的是我的輸入字串有什麼樣的編碼?因為如果你將使用 std::lower
與多位元組編碼字元,那麼你一定會得到錯誤的程式碼。
即使下面的函式看起來是對 std::string
小寫轉換的整潔實現,當你期望輸入的是 UTF-8
編碼字元時,它不會將所有的字元轉換為小寫。
#include <algorithm>
#include <iostream>
std::string toLower(std::string s) {
std::transform(s.begin(), s.end(), s.begin(),
[](unsigned char c) { return std::tolower(c); });
return s;
}
int main() {
std::string string1 = u8"ÅSH to LoWer WÅN";
std::cout << "input string: " << string1 << std::endl
<< "output string: " << toLower(string1) << std::endl;
return 0;
}
上面的程式碼對於 ASCII 字串和其他一些非 ASCII 字串也能很好的工作,但是一旦你給它一個有點不尋常的輸入,比如說裡面有一些拉丁符號,輸出就會不盡人意。
輸出:
input string: ÅSH to LoWer WÅN
output string: Åsh to lower wÅn
它是不正確的,因為它應該把 Å
改為小寫的 å
。那麼,我們怎樣才能解決這個問題,得到正確的輸出呢?
最好的可移植方式是使用 ICU
(International Components for Unicode,國際統一碼元件)庫,它足夠成熟,提供了穩定性,廣泛的可訪問性,並將保持你的程式碼跨平臺。
我們只需要在原始檔中包含以下標頭檔案。這些庫很有可能已經包含在你的平臺上,並且在你的平臺上可用,所以程式碼樣本應該可以正常工作。但如果你在 IDE/編譯時出現錯誤,請參考 ICU 文件網站中的庫下載說明。
#include <unicode/locid.h>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
現在我們已經包含了標頭檔案,所以我們可以寫 std::string
到小寫的轉換程式碼如下。
#include <unicode/locid.h>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
#include <iostream>
int main() {
std::string string1 = u8"ÅSH to LoWer WÅN";
icu::UnicodeString unicodeString(string1.c_str());
std::cout << "input string: " << string1 << std::endl
<< "output string: " << unicodeString.toLower() << std::endl;
return 0;
}
注意,我們在編譯這段程式碼時,應該使用以下編譯器標誌,以包含 ICU 庫的依賴性。
g++ sample_code.cpp -licuio -licuuc -o sample_code
執行程式碼,我們將按預期獲得正確的輸出:
input string: ÅSH to LoWer WÅN
output string: åsh to lower wån
完全相同的函式可以處理一些我們通常不希望作為使用者輸入的語言,並且我們還可以顯式地將語言環境指定為 toLower
函式的引數:
#include <iostream>
#include <unicode/unistr.h>
#include <unicode/ustream.h>
#include <unicode/locid.h>
int main() {
std::string string2 = "Κάδμῳ ἀπιϰόμενοι.. Ελληνας ϰαὶ δὴ ϰαὶ γράμματα, οὐϰ ἐόντα πρὶν Ελλησι";
icu::UnicodeString unicodeString2(string2.c_str());
std::cout << unicodeString2.toLower("el_GR") << std::endl;
return 0;
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu