Pandas DataFrame DataFrame.interpolate() 함수
-
pandas.DataFrame.interpolate()
구문 : -
예제 코드:
DataFrame.interpolate()
메서드를 사용하여DataFrame
의 모든NaN
값 보간 -
예제 코드:
method
매개 변수가있는DataFrame.interpolate()
메서드 -
예제 코드:
row
축을 따라 보간 할axis
매개 변수가있는 PandasDataFrame.interpolate()
메서드 -
예제 코드:
limit
매개 변수가있는DataFrame.interpolate()
메서드 -
예제 코드:
limit_direction
매개 변수가있는DataFrame.interpolate()
메서드 -
DataFrame.interpolate()
메서드를 사용하여 시계열 데이터 보간
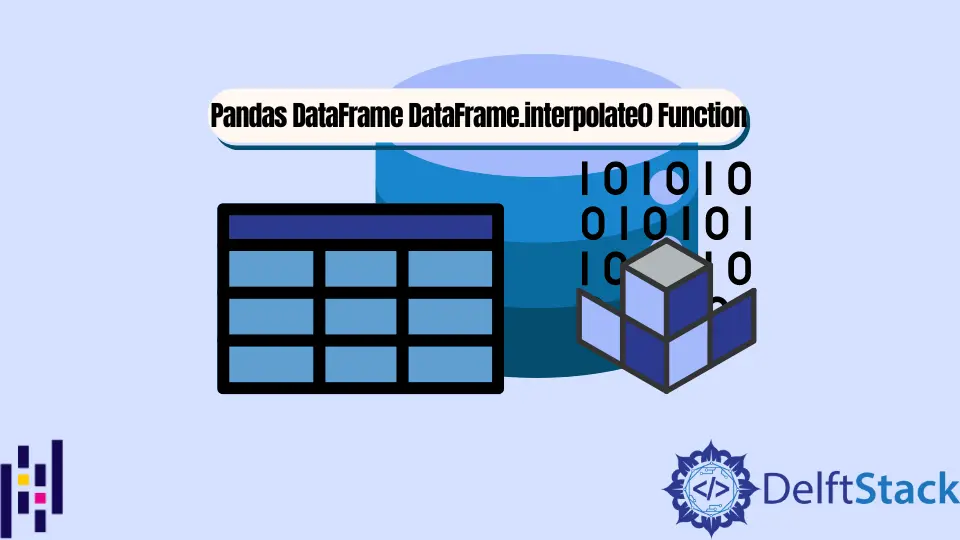
Python Pandas DataFrame.interpolate()
함수는 DataFrame의 NaN
값을 채 웁니다. 보간 기법을 사용합니다.
pandas.DataFrame.interpolate()
구문 :
DataFrame.interpolate(
method="linear",
axis=0,
limit=None,
inplace=False,
limit_direction="forward",
limit_area=None,
downcast=None,
**kwargs
)
매개 변수
method |
linear ,time ,index ,values ,nearest ,zero ,slinear ,quadratic ,cubic ,barycentric ,krogh ,polynomial ,spline ,piecewise_polynomial ,from_derivatives ,pchip ,akima 또는None . NaN 값을 보간하는 데 사용되는 방법입니다. |
axis |
행(axis=0 ) 또는 열(axis=1 )을 따라 누락 된 값 보간 |
limit |
정수. 보간 할 연속NaN 의 최대 수입니다. |
inplace |
부울. True 이면 호출자DataFrame 을 제자리에서 수정합니다. |
limit_direction |
forward , backward 또는both . 제한 이 지정되면NaN 을 따라limit 이 보간됩니다. |
limit_area |
None , inside 또는outside . limit 이 지정된 경우 보간에 대한 제한 |
downcast |
사전. 데이터 유형의 다운 캐스트를 지정합니다. |
**kwargs |
보간 함수에 대한 키워드 인수입니다. |
반환
inplace
가True
이면 주어진method
를 사용하여 모든NaN
값을 보간하는DataFrame
; 그렇지 않으면 None
.
예제 코드: DataFrame.interpolate()
메서드를 사용하여DataFrame
의 모든NaN
값 보간
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3, None, 3],
'Y': [4, None, 8, None, 3]})
print("DataFrame:")
print(df)
filled_df = df.interpolate()
print("Interploated DataFrame:")
print(filled_df)
출력:
DataFrame:
X Y
0 1.0 4.0
1 2.0 NaN
2 3.0 8.0
3 NaN NaN
4 3.0 3.0
Interploated DataFrame:
X Y
0 1.0 4.0
1 2.0 6.0
2 3.0 8.0
3 3.0 5.5
4 3.0 3.0
그것은linear
보간 방법을 사용하여DataFrame
의 모든NaN
값을 보간합니다.
이 방법은 다음을 사용하는 pandas.DataFrame.fillna()
에 비해 더 지능적입니다. DataFrame
의 모든 NaN
값을 대체하는 고정 값
예제 코드: method
매개 변수가있는DataFrame.interpolate()
메서드
DataFrame.interpolate()
함수에서method
매개 변수의 값을 설정하는 다른 보간 기법으로DataFrame
의NaN
값을 보간 할 수도 있습니다.
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3, None, 3],
'Y': [4, None, 8, None, 3]})
print("DataFrame:")
print(df)
filled_df = df.interpolate(method='polynomial', order=2)
print("Interploated DataFrame:")
print(filled_df)
출력:
DataFrame:
X Y
0 1.0 4.0
1 2.0 NaN
2 3.0 8.0
3 NaN NaN
4 3.0 3.0
Interploated DataFrame:
X Y
0 1.000000 4.000
1 2.000000 7.125
2 3.000000 8.000
3 3.368421 6.625
4 3.000000 3.000
이 방법은 2 차 NaN
보간 방법을 사용하여 DataFrame
의 모든 NaN
값을 보간합니다.
여기서 order = 2
는 NaN
함수의 키워드 인수입니다.
예제 코드: row
축을 따라 보간 할axis
매개 변수가있는 Pandas DataFrame.interpolate()
메서드
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3, None, 3],
'Y': [4, None, 8, None, 3]})
print("DataFrame:")
print(df)
filled_df = df.interpolate(axis=1)
print("Interploated DataFrame:")
print(filled_df)
출력:
DataFrame:
X Y
0 1.0 4.0
1 2.0 NaN
2 3.0 8.0
3 NaN NaN
4 3.0 3.0
Interploated DataFrame:
X Y
0 1.0 4.0
1 2.0 2.0
2 3.0 8.0
3 NaN NaN
4 3.0 3.0
여기서는axis=1
을 설정하여 행 축을 따라NaN
값을 보간합니다. 두 번째 행에서 NaN
값은 두 번째 행을 따라 선형 보간을 사용하여 대체됩니다.
단, 4 번째 행에서는 4 번째 행의 값이 모두 NaN
이므로 보간 후에도 NaN
값이 유지됩니다.
예제 코드: limit
매개 변수가있는DataFrame.interpolate()
메서드
DataFrame.interpolate()
메서드의limit
매개 변수는 메서드가 채울 연속NaN
값의 최대 개수를 제한합니다.
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3, None, 3],
'Y': [4, None, None, None, 3]})
print("DataFrame:")
print(df)
filled_df = df.interpolate( limit = 1)
print("Interploated DataFrame:")
print(filled_df)
출력:
DataFrame:
X Y
0 1.0 4.0
1 2.0 NaN
2 3.0 NaN
3 NaN NaN
4 3.0 3.0
Interploated DataFrame:
X Y
0 1.0 4.00
1 2.0 3.75
2 3.0 NaN
3 3.0 NaN
4 3.0 3.00
여기서 NaN
이 상단부터 열에 채워지면 동일한 열의 다음 연속 NaN
값은 변경되지 않습니다.
예제 코드: limit_direction
매개 변수가있는DataFrame.interpolate()
메서드
DataFrame.interpolate()
메서드의limit-direction
매개 변수는 값이 보간되는 특정 축을 따라 방향을 제어합니다.
import pandas as pd
df = pd.DataFrame({'X': [1, 2, 3, None, 3],
'Y': [4, None, None, None, 3]})
print("DataFrame:")
print(df)
filled_df = df.interpolate(limit_direction ='backward', limit = 1)
print("Interploated DataFrame:")
print(filled_df)
출력:
DataFrame:
X Y
0 1.0 4.0
1 2.0 NaN
2 3.0 NaN
3 NaN NaN
4 3.0 3.0
Interploated DataFrame:
X Y
0 1.0 4.00
1 2.0 NaN
2 3.0 NaN
3 3.0 3.25
4 3.0 3.00
여기서 NaN
이 맨 아래부터 열에 채워지면 동일한 열의 다음 연속 NaN
값은 변경되지 않습니다.
DataFrame.interpolate()
메서드를 사용하여 시계열 데이터 보간
import pandas as pd
dates=['April-10', 'April-11', 'April-12', 'April-13']
fruits=['Apple', 'Papaya', 'Banana', 'Mango']
prices=[3, None, 2, 4]
df = pd.DataFrame({'Date':dates ,
'Fruit':fruits ,
'Price': prices})
print(df)
df.interpolate(inplace=True)
print("Interploated DataFrame:")
print(df)
출력:
Date Fruit Price
0 April-10 Apple 3.0
1 April-11 Papaya NaN
2 April-12 Banana 2.0
3 April-13 Mango 4.0
Interploated DataFrame:
Date Fruit Price
0 April-10 Apple 3.0
1 April-11 Papaya 2.5
2 April-12 Banana 2.0
3 April-13 Mango 4.0
inplace = True
로 인해interpolate()
함수를 호출 한 후 원래DataFrame
이 수정됩니다.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn