Pandas DataFrame.describe() 함수
Minahil Noor
2023년1월30일
-
pandas.DataFrame.describe()
의 구문 : -
예제 코드: 데이터 프레임의 통계를 찾는
DataFrame.describe()
메서드 -
예제 코드 : 각 열의 통계를 찾는
DataFrame.describe()
메서드 -
예제 코드: 숫자 열의 통계를 찾는
DataFrame.describe()
메서드
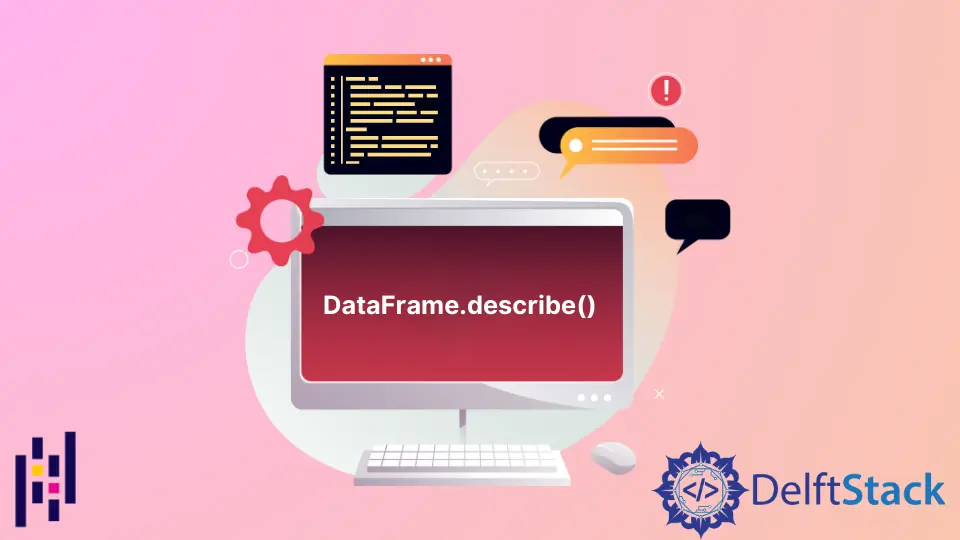
Python Pandas DataFrame.describe()
함수는 데이터 프레임의 통계 데이터에 대해 알려줍니다.
pandas.DataFrame.describe()
의 구문 :
DataFrame.describe(
percentiles=None, include=None, exclude=None, datetime_is_numeric=False
)
매개 변수
percentiles |
이 매개 변수는 출력에 포함 할 백분위 수에 대해 알려줍니다. 모든 값은 0에서 1 사이 여야합니다. 기본값은 25, 50, 75 번째 백분위 수를 반환하는[.25, .5, .75] 입니다. |
include |
출력에 포함 할 데이터 유형에 대해 알려줍니다. 세 가지 옵션이 있습니다. all : 입력의 모든 열이 출력에 포함됩니다. 목록과 유사한 데이터 유형 : 제공된 데이터 유형으로 결과를 제한합니다. None : 결과에 모든 숫자 열이 포함됩니다. |
exclude |
출력에서 제외 할 데이터 유형에 대해 알려줍니다. 두 가지 옵션이 있습니다. 목록과 유사한 데이터 유형 : 제공된 데이터 유형을 결과에서 제외합니다. None : 결과에서 아무것도 제외하지 않습니다. |
datetime_is_numeric |
부울 매개 변수. datetime 데이터 유형을 숫자로 처리할지 여부를 알려줍니다. |
반환
전달 된Series
또는 Dataframe의 통계 요약을 반환합니다.
예제 코드: 데이터 프레임의 통계를 찾는DataFrame.describe()
메서드
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Name': {0: 'Olivia', 1: 'John', 2: 'Laura',3: 'Ben',4: 'Kevin'},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data frame is: \n")
print(dataframe)
dataframe1 = dataframe.describe()
print("Statistics are: \n")
print(dataframe1)
출력:
The Original Data frame is:
Attendance Name Obtained Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
Statistics are:
Attendance Obtained Marks
count 5.000000 5.000000
mean 82.600000 71.200000
std 15.773395 17.484279
min 60.000000 45.000000
25% 78.000000 64.000000
50% 80.000000 75.000000
75% 95.000000 82.000000
max 100.000000 90.000000
이 함수는 데이터 프레임의 통계 요약을 반환했습니다. 매개 변수를 전달하지 않았으므로 함수는 모든 기본값을 사용했습니다.
예제 코드 : 각 열의 통계를 찾는DataFrame.describe()
메서드
include
매개 변수를 사용하여 모든 열의 통계를 찾습니다.
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Name': {0: 'Olivia', 1: 'John', 2: 'Laura',3: 'Ben',4: 'Kevin'},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data frame is: \n")
print(dataframe)
dataframe1 = dataframe.describe(include='all')
print("Statistics are: \n")
print(dataframe1)
출력:
The Original Data frame is:
Attendance Name Obtained Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
Statistics are:
Attendance Name Obtained Marks
count 5.000000 5 5.000000
unique NaN 5 NaN
top NaN Kevin NaN
freq NaN 1 NaN
mean 82.600000 NaN 71.200000
std 15.773395 NaN 17.484279
min 60.000000 NaN 45.000000
25% 78.000000 NaN 64.000000
50% 80.000000 NaN 75.000000
75% 95.000000 NaN 82.000000
max 100.000000 NaN 90.000000
이 함수는 데이터 프레임의 모든 열에 대한 통계 요약을 반환했습니다.
예제 코드: 숫자 열의 통계를 찾는DataFrame.describe()
메서드
이제 exclude
매개 변수 만 사용하여 숫자 열의 통계를 찾습니다.
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Name': {0: 'Olivia', 1: 'John', 2: 'Laura',3: 'Ben',4: 'Kevin'},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data frame is: \n")
print(dataframe)
dataframe1 = dataframe.describe(exclude=[object])
print("Statistics are: \n")
print(dataframe1)
출력:
The Original Data frame is:
Attendance Name Obtained Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
Statistics are:
Attendance Obtained Marks
count 5.000000 5.000000
mean 82.600000 71.200000
std 15.773395 17.484279
min 60.000000 45.000000
25% 78.000000 64.000000
50% 80.000000 75.000000
75% 95.000000 82.000000
max 100.000000 90.000000
데이터 유형 object
를 제외했습니다.