Pandas DataFrame.describe() 関数
Minahil Noor
2023年1月30日
-
pandas.DataFrame.describe()
の構文: -
コード例:データフレームの統計を検索するための
DataFrame.describe()
メソッド -
コード例:各列の統計を検索するための
DataFrame.describe()
メソッド -
コード例:数値列の統計を検索するための
DataFrame.describe()
メソッド
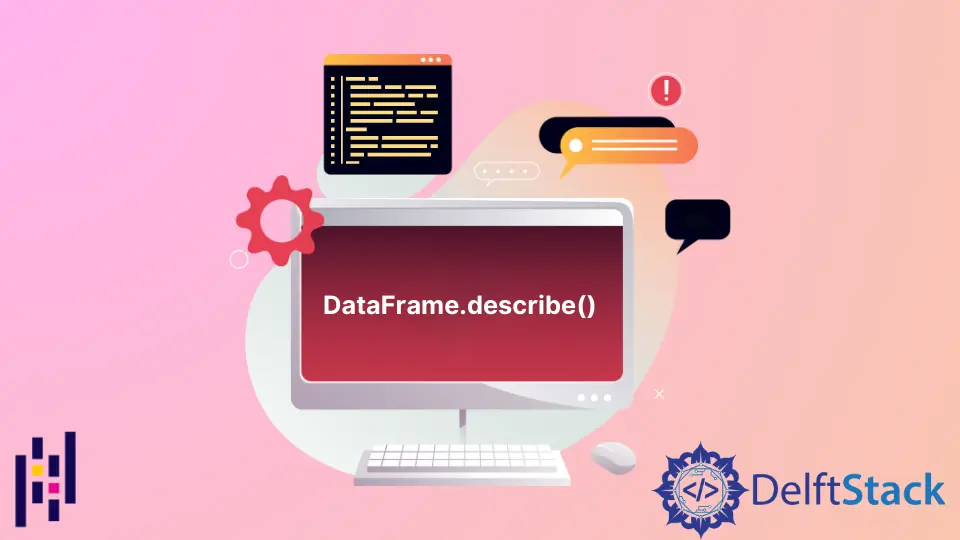
Python Pandas DataFrame.describe()
関数はデータフレームの統計データについて説明します。
pandas.DataFrame.describe()
の構文:
DataFrame.describe(
percentiles=None, include=None, exclude=None, datetime_is_numeric=False
)
パラメータ
percentiles |
このパラメータは、出力に含めるパーセンタイルを指定します。デフォルトは [.25, .5, .75] で、25, 50, 75%のパーセンタイルを返します。 |
include |
出力に含めるデータ型について説明します。3つのオプションがあります。all : 入力のすべての列が出力に含まれます。データ型のリストのようなもの: 指定されたデータ型に結果を制限します。None : 入力のすべての数値列が出力に含まれます。結果にはすべての数値列が含まれます。 |
exclude |
出力から除外するデータ型を指定します。これには 2つのオプションがあります。データ型のリストのようなもの: 指定されたデータ型を結果から除外します。None 。結果は何も除外しません。 |
datetime_is_numeric |
ブール値パラメータ。datetime データ型を数値として扱うかどうかを指定します。 |
戻り値
渡された Series
またはデータフレームの統計情報の要約を返します。
コード例:データフレームの統計を検索するための DataFrame.describe()
メソッド
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Name': {0: 'Olivia', 1: 'John', 2: 'Laura',3: 'Ben',4: 'Kevin'},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data frame is: \n")
print(dataframe)
dataframe1 = dataframe.describe()
print("Statistics are: \n")
print(dataframe1)
出力:
The Original Data frame is:
Attendance Name Obtained Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
Statistics are:
Attendance Obtained Marks
count 5.000000 5.000000
mean 82.600000 71.200000
std 15.773395 17.484279
min 60.000000 45.000000
25% 78.000000 64.000000
50% 80.000000 75.000000
75% 95.000000 82.000000
max 100.000000 90.000000
この関数は、データフレームの統計情報の要約を返しました。パラメータは何も渡されていないので、関数はすべてのデフォルト値を使用しています。
コード例:各列の統計を検索するための DataFrame.describe()
メソッド
include
パラメータを使用してすべての列の統計を検索します。
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Name': {0: 'Olivia', 1: 'John', 2: 'Laura',3: 'Ben',4: 'Kevin'},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data frame is: \n")
print(dataframe)
dataframe1 = dataframe.describe(include='all')
print("Statistics are: \n")
print(dataframe1)
出力:
The Original Data frame is:
Attendance Name Obtained Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
Statistics are:
Attendance Name Obtained Marks
count 5.000000 5 5.000000
unique NaN 5 NaN
top NaN Kevin NaN
freq NaN 1 NaN
mean 82.600000 NaN 71.200000
std 15.773395 NaN 17.484279
min 60.000000 NaN 45.000000
25% 78.000000 NaN 64.000000
50% 80.000000 NaN 75.000000
75% 95.000000 NaN 82.000000
max 100.000000 NaN 90.000000
この関数はデータフレームの全列の統計情報の要約を返しました。
コード例:数値列の統計を検索するための DataFrame.describe()
メソッド
ここで、exclude
パラメーターのみを使用して数値列の統計を検索します。
import pandas as pd
dataframe=pd.DataFrame({'Attendance': {0: 60, 1: 100, 2: 80,3: 78,4: 95},
'Name': {0: 'Olivia', 1: 'John', 2: 'Laura',3: 'Ben',4: 'Kevin'},
'Obtained Marks': {0: 90, 1: 75, 2: 82, 3: 64, 4: 45}})
print("The Original Data frame is: \n")
print(dataframe)
dataframe1 = dataframe.describe(exclude=[object])
print("Statistics are: \n")
print(dataframe1)
出力:
The Original Data frame is:
Attendance Name Obtained Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
Statistics are:
Attendance Obtained Marks
count 5.000000 5.000000
mean 82.600000 71.200000
std 15.773395 17.484279
min 60.000000 45.000000
25% 78.000000 64.000000
50% 80.000000 75.000000
75% 95.000000 82.000000
max 100.000000 90.000000
データ型 object
を除外しました。