VBA での関数の使用
Glen Alfaro
2023年1月30日
VBA
VBA Function
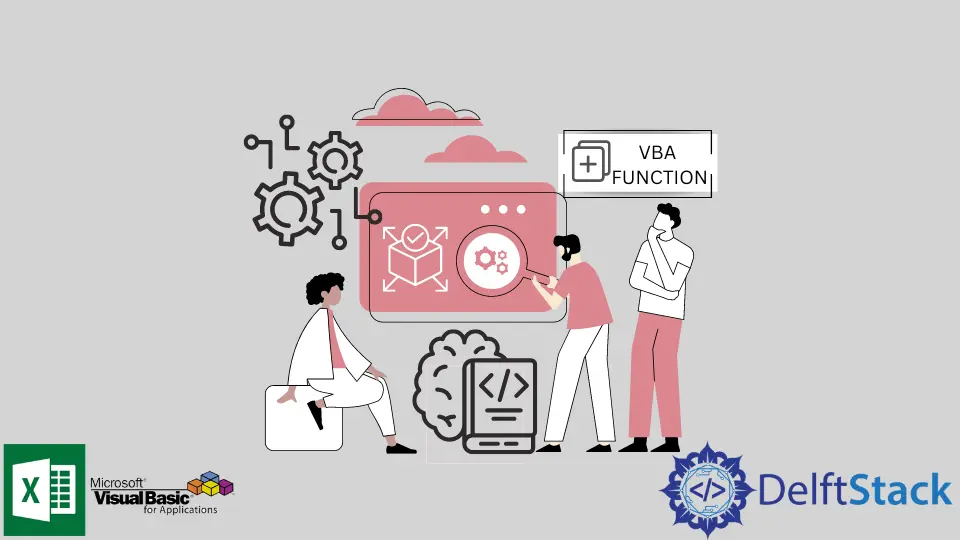
この記事では、VBA を処理するときに関数を作成して利用する方法を示します。
関数は、サブルーチンができない結果を返すために使用されます。プログラムが長く複雑になると、関数はコードブロックの編成、デバッグ、およびスクリプトの改訂を容易にするための便利なツールになります。
関数は、次の 2つのサブセットに分類できます。
non-object-type |
文字列、整数、ブール値などを返す関数 |
object-type |
範囲、配列リストなどのオブジェクトを返す関数。 |
構文:
[Public | Private] [ Static ] Function name [ ( argumentlist ) ] [ As retType ]
[ statements ]
[ name = expression ]
[ Exit Function ]
End Function
意味:
Public |
オプション。すべてのモジュールの他のプロシージャが関数にアクセスできることを示します |
Private |
オプション。現在のモジュールのプロシージャによってのみ関数にアクセスできることを示します |
Static |
オプション。プロシージャ変数が関数呼び出し間で保持されることを示します |
name |
必須。関数の名前 |
argumentlist |
オプション。関数が呼び出されたときに渡される変数のリスト。 |
retType |
オプション。関数の戻り値タイプ |
statements |
実行するコードブロック |
expression |
関数の戻り値 |
VBA で non-object
関数型を使用する
以下のコードブロックは、non-object
関数型を使用して、引数として底辺と高さを指定して三角形の面積を計算します。
Public Function GetTriangleArea(b, h) as Double
Dim area as Double
area= (b*h)/2
GetTriangleArea= area
End Function
Sub TestFunction()
Debug.print("The area of the triangle is " & GetTriangleArea(10,8) & " square meters.")
End Sub
出力:
The area of the triangle is 40 square meters.
VBA での object
関数型の使用
以下のコードブロックは、object
関数型を使用して、2つの数値の間のすべての数値の合計を計算する方法を示しています。
Public Function GetCollection(numStr, numEnd) As Object
'Declaring variable for the counter
Dim i As Integer
'Creating a new Arraylist named coll
Dim coll As Object
Set coll = CreateObject("System.Collections.ArrayList")
'Add all number from numStr to numEnd to the arraylist named coll using a for loop
For i = numStr To numEnd
coll.Add (i)
Next i
'Returning the arraylist named coll to the subroutine
Set GetCollection = coll
End Function
Sub testFunction()
'Declaring collForSum as an Object
'collForSum object will be the placeholder object where the coll arraylist will be in placed
Dim collForSum As Object
'Setting up a counter named j
Dim j As Integer
'Sum variable will hold the running sum of the elements in collForSum
Dim sum As Double
'Calling the GetCollection Function to fill collForSUm Object
Set collForSum = GetCollection(10, 20)
'For loop to iterate on all element of collForSum arraylist
For j = 0 To collForSum.Count - 1
'Add all the elements inside the collForSum arraylist.
sum = sum + collForSum(j)
Next j
Debug.Print ("The total sum is " & sum & ".")
End Sub
出力:
The total sum is 165.
サブルーチンに強制的に戻るための Exit Function
コマンドの使用
サブルーチンまたは関数に値を返すことは、必ずしも現在の関数のコード実行が停止することを意味するわけではありません。関数でのコード実行を明示的に停止することを宣言する必要があります。使用するコマンドは Exit Function
です。
Exit Function
コマンドなしで関数を呼び出すと、間違った値が返される場合があります。
Public Function PositiveOrNegative(num) as String
If num >= 0 Then
PositiveOrNegative = "Positive"
End If
PositiveOrNegative = "Negative"
End Function
Sub TestFunction()
Debug.Print (PositiveOrNegative(5))
End Sub
TestFunction
出力:
Negative
PositiveOrNegative = "Positive"
コード行に達したときにコードの実行が停止しなかったため、TestFunction
入力が 5
であっても、出力は Negative
です。
これを解決するには、Exit Function
コマンドを使用する必要があります。
Public Function PositiveOrNegative(num) as String
If num >= 0 Then
PositiveOrNegative = "Positive"
'Exit Function will force the code execution in the current function to stop and return the current value of PositiveOrNegative
Exit Function
End If
PositiveOrNegative = "Negative"
End Function
Sub TestFunction()
Debug.Print (PositiveOrNegative(5))
End Sub
出力:
Positive
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe