El uso de la función en VBA
-
Uso del tipo de función
non-object
en VBA -
Uso del tipo de función
object
en VBA -
Uso del Comando
Salir de la Función
para un Regreso Forzado a la Subrutina
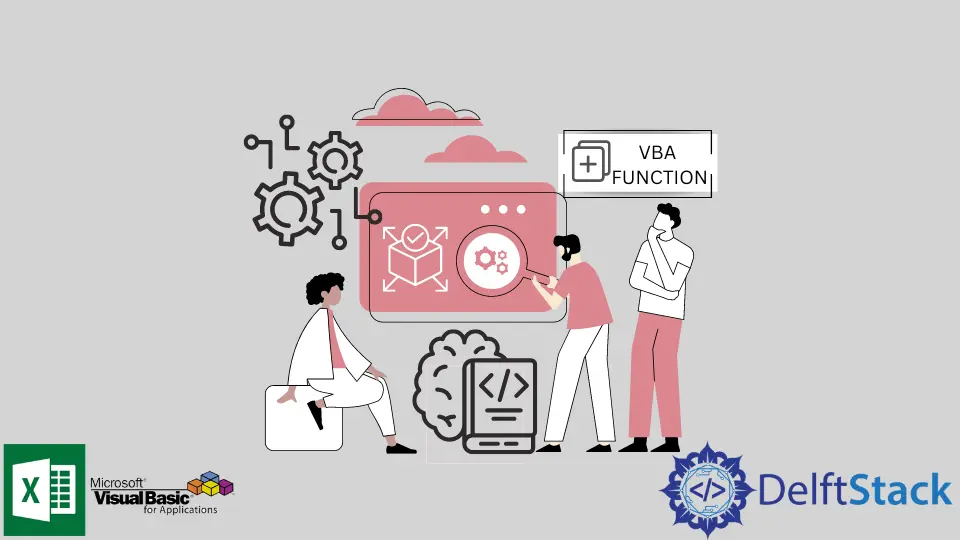
Este artículo demostrará cómo crear y utilizar una función cuando se trata de VBA.
Las funciones se utilizan para devolver un resultado donde una subrutina no puede. Cuando el programa se vuelve más largo y complejo, las funciones son una herramienta útil para facilitar la organización de bloques de código, la depuración y la revisión del script.
Las funciones se pueden clasificar en dos subconjuntos:
non-object-type |
función que devolverá cadenas, enteros, booleanos, etc. |
object-type |
función que devolverá objetos como rangos, listas de arreglos, etc. |
Sintaxis:
[Public | Private] [ Static ] Function name [ ( argumentlist ) ] [ As retType ]
[ statements ]
[ name = expression ]
[ Exit Function ]
End Function
Definición:
Public |
Opcional. indica que otros procedimientos en todos los módulos pueden acceder a la función |
Private |
Opcional. indica que solo se puede acceder a la función mediante procedimientos en el módulo actual |
Static |
Opcional. indica que la variable de procedimientos se conserva entre llamadas a funciones |
name |
Requerido. Nombre de la Función |
argumentlist |
Opcional. Lista de variables que se pasan una vez que se llama a la función. |
retType |
Opcional. Tipo de retorno de la función |
statements |
Bloque de código para ejecutar |
expression |
Valor de retorno de la función |
Uso del tipo de función non-object
en VBA
El siguiente bloque de código calculará el área de un triángulo dada su base y altura como sus argumentos utilizando un tipo de función non-object
.
Public Function GetTriangleArea(b, h) as Double
Dim area as Double
area= (b*h)/2
GetTriangleArea= area
End Function
Sub TestFunction()
Debug.print("The area of the triangle is " & GetTriangleArea(10,8) & " square meters.")
End Sub
Producción :
The area of the triangle is 40 square meters.
Uso del tipo de función object
en VBA
El siguiente bloque de código demostrará cómo usar un tipo de función object
para calcular la suma de todos los números entre dos números.
Public Function GetCollection(numStr, numEnd) As Object
'Declaring variable for the counter
Dim i As Integer
'Creating a new Arraylist named coll
Dim coll As Object
Set coll = CreateObject("System.Collections.ArrayList")
'Add all number from numStr to numEnd to the arraylist named coll using a for loop
For i = numStr To numEnd
coll.Add (i)
Next i
'Returning the arraylist named coll to the subroutine
Set GetCollection = coll
End Function
Sub testFunction()
'Declaring collForSum as an Object
'collForSum object will be the placeholder object where the coll arraylist will be in placed
Dim collForSum As Object
'Setting up a counter named j
Dim j As Integer
'Sum variable will hold the running sum of the elements in collForSum
Dim sum As Double
'Calling the GetCollection Function to fill collForSUm Object
Set collForSum = GetCollection(10, 20)
'For loop to iterate on all element of collForSum arraylist
For j = 0 To collForSum.Count - 1
'Add all the elements inside the collForSum arraylist.
sum = sum + collForSum(j)
Next j
Debug.Print ("The total sum is " & sum & ".")
End Sub
Producción :
The total sum is 165.
Uso del Comando Salir de la Función
para un Regreso Forzado a la Subrutina
Devolver un valor a una subrutina o una función no significa necesariamente que la ejecución del código en la función actual se detendrá. Necesitamos declarar para detener la ejecución del código en la función explícitamente. El comando a utilizar es Exit Function
.
Llamar a una función sin el comando Exit Function
puede devolver valores incorrectos.
Public Function PositiveOrNegative(num) as String
If num >= 0 Then
PositiveOrNegative = "Positive"
End If
PositiveOrNegative = "Negative"
End Function
Sub TestFunction()
Debug.Print (PositiveOrNegative(5))
End Sub
Salida TestFunction
:
Negative
La salida es Negativa
aunque la entrada TestFunction
sea 5
ya que la ejecución del código no se detuvo cuando llegó a la línea de código PositiveOrNegative = "Positive"
.
Necesitamos utilizar el comando Salir de la función
para resolver esto.
Public Function PositiveOrNegative(num) as String
If num >= 0 Then
PositiveOrNegative = "Positive"
'Exit Function will force the code execution in the current function to stop and return the current value of PositiveOrNegative
Exit Function
End If
PositiveOrNegative = "Negative"
End Function
Sub TestFunction()
Debug.Print (PositiveOrNegative(5))
End Sub
Producción :
Positive