VBA 中函数的使用
Glen Alfaro
2023年1月30日
VBA
VBA Function
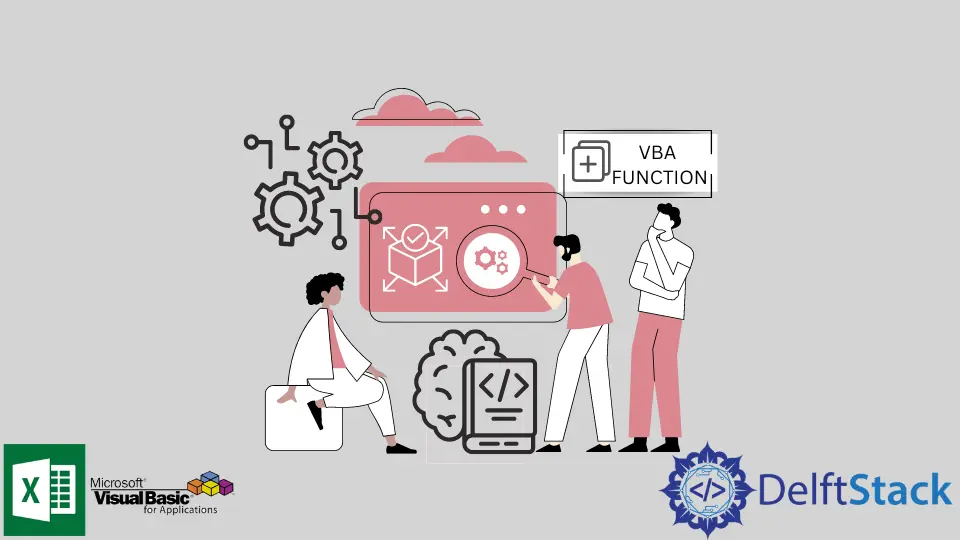
本文将演示在处理 VBA 时如何创建和使用函数。
函数用于返回子例程无法返回的结果。当程序变得更长和更复杂时,函数是一种有用的工具,可以更轻松地组织代码块、调试和修改脚本。
函数可以分为两个子集:
non-object-type |
将返回字符串、整数、布尔值等的函数 |
object-type |
函数将返回对象,如范围、数组列表等。 |
语法:
[Public | Private] [ Static ] Function name [ ( argumentlist ) ] [ As retType ]
[ statements ]
[ name = expression ]
[ Exit Function ]
End Function
定义:
Public |
可选的。表示所有模块中的其他程序都可以访问该函数 |
Private |
可选的。表示该函数只能被当前模块中的过程访问 |
Static |
可选的。表示程序变量在函数调用之间保留 |
name |
必需的。函数名称 |
argumentlist |
可选的。调用函数后传递的变量列表。 |
retType |
可选的。函数的返回类型 |
statements |
要执行的代码块 |
expression |
函数的返回值 |
在 VBA 中使用非对象
函数类型
下面的代码块将使用非对象
函数类型计算三角形的面积,给定它的底和高作为其参数。
Public Function GetTriangleArea(b, h) as Double
Dim area as Double
area= (b*h)/2
GetTriangleArea= area
End Function
Sub TestFunction()
Debug.print("The area of the triangle is " & GetTriangleArea(10,8) & " square meters.")
End Sub
输出:
The area of the triangle is 40 square meters.
在 VBA 中使用 object
函数类型
下面的代码块将演示如何使用 object
函数类型来计算两个数字之间所有数字的总和。
Public Function GetCollection(numStr, numEnd) As Object
'Declaring variable for the counter
Dim i As Integer
'Creating a new Arraylist named coll
Dim coll As Object
Set coll = CreateObject("System.Collections.ArrayList")
'Add all number from numStr to numEnd to the arraylist named coll using a for loop
For i = numStr To numEnd
coll.Add (i)
Next i
'Returning the arraylist named coll to the subroutine
Set GetCollection = coll
End Function
Sub testFunction()
'Declaring collForSum as an Object
'collForSum object will be the placeholder object where the coll arraylist will be in placed
Dim collForSum As Object
'Setting up a counter named j
Dim j As Integer
'Sum variable will hold the running sum of the elements in collForSum
Dim sum As Double
'Calling the GetCollection Function to fill collForSUm Object
Set collForSum = GetCollection(10, 20)
'For loop to iterate on all element of collForSum arraylist
For j = 0 To collForSum.Count - 1
'Add all the elements inside the collForSum arraylist.
sum = sum + collForSum(j)
Next j
Debug.Print ("The total sum is " & sum & ".")
End Sub
输出:
The total sum is 165.
使用 Exit Function
命令强制返回子程序
将值返回给子例程或函数并不一定意味着当前函数中的代码执行将停止。我们需要明确声明停止函数上的代码执行。要使用的命令是退出功能
。
在没有 Exit Function
命令的情况下调用函数可能会返回错误值。
Public Function PositiveOrNegative(num) as String
If num >= 0 Then
PositiveOrNegative = "Positive"
End If
PositiveOrNegative = "Negative"
End Function
Sub TestFunction()
Debug.Print (PositiveOrNegative(5))
End Sub
TestFunction
输出:
Negative
即使 TestFunction
输入是 5
,输出也是 Negative
,因为代码执行在到达 PositiveOrNegative = "Positive"
代码行时并没有停止。
我们需要使用 Exit Function
命令来解决这个问题。
Public Function PositiveOrNegative(num) as String
If num >= 0 Then
PositiveOrNegative = "Positive"
'Exit Function will force the code execution in the current function to stop and return the current value of PositiveOrNegative
Exit Function
End If
PositiveOrNegative = "Negative"
End Function
Sub TestFunction()
Debug.Print (PositiveOrNegative(5))
End Sub
输出:
Positive
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe