VBA 中函式的使用
Glen Alfaro
2023年1月30日
VBA
VBA Function
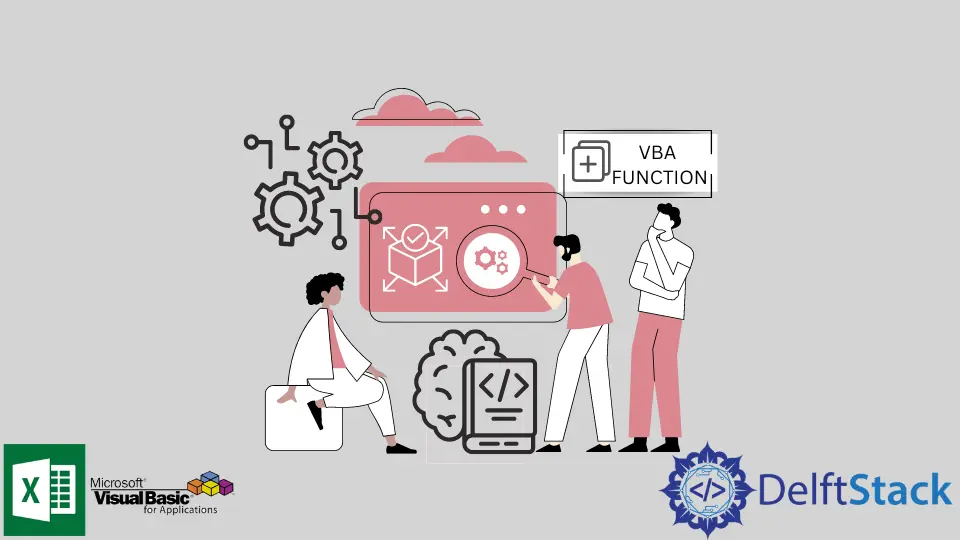
本文將演示在處理 VBA 時如何建立和使用函式。
函式用於返回子例程無法返回的結果。當程式變得更長和更復雜時,函式是一種有用的工具,可以更輕鬆地組織程式碼塊、除錯和修改指令碼。
函式可以分為兩個子集:
non-object-type |
將返回字串、整數、布林值等的函式 |
object-type |
函式將返回物件,如範圍、陣列列表等。 |
語法:
[Public | Private] [ Static ] Function name [ ( argumentlist ) ] [ As retType ]
[ statements ]
[ name = expression ]
[ Exit Function ]
End Function
定義:
Public |
可選的。表示所有模組中的其他程式都可以訪問該函式 |
Private |
可選的。表示該函式只能被當前模組中的過程訪問 |
Static |
可選的。表示程式變數在函式呼叫之間保留 |
name |
必需的。函式名稱 |
argumentlist |
可選的。呼叫函式後傳遞的變數列表。 |
retType |
可選的。函式的返回型別 |
statements |
要執行的程式碼塊 |
expression |
函式的返回值 |
在 VBA 中使用非物件
函式型別
下面的程式碼塊將使用非物件
函式型別計算三角形的面積,給定它的底和高作為其引數。
Public Function GetTriangleArea(b, h) as Double
Dim area as Double
area= (b*h)/2
GetTriangleArea= area
End Function
Sub TestFunction()
Debug.print("The area of the triangle is " & GetTriangleArea(10,8) & " square meters.")
End Sub
輸出:
The area of the triangle is 40 square meters.
在 VBA 中使用 object
函式型別
下面的程式碼塊將演示如何使用 object
函式型別來計算兩個數字之間所有數字的總和。
Public Function GetCollection(numStr, numEnd) As Object
'Declaring variable for the counter
Dim i As Integer
'Creating a new Arraylist named coll
Dim coll As Object
Set coll = CreateObject("System.Collections.ArrayList")
'Add all number from numStr to numEnd to the arraylist named coll using a for loop
For i = numStr To numEnd
coll.Add (i)
Next i
'Returning the arraylist named coll to the subroutine
Set GetCollection = coll
End Function
Sub testFunction()
'Declaring collForSum as an Object
'collForSum object will be the placeholder object where the coll arraylist will be in placed
Dim collForSum As Object
'Setting up a counter named j
Dim j As Integer
'Sum variable will hold the running sum of the elements in collForSum
Dim sum As Double
'Calling the GetCollection Function to fill collForSUm Object
Set collForSum = GetCollection(10, 20)
'For loop to iterate on all element of collForSum arraylist
For j = 0 To collForSum.Count - 1
'Add all the elements inside the collForSum arraylist.
sum = sum + collForSum(j)
Next j
Debug.Print ("The total sum is " & sum & ".")
End Sub
輸出:
The total sum is 165.
使用 Exit Function
命令強制返回子程式
將值返回給子例程或函式並不一定意味著當前函式中的程式碼執行將停止。我們需要明確宣告停止函式上的程式碼執行。要使用的命令是退出功能
。
在沒有 Exit Function
命令的情況下呼叫函式可能會返回錯誤值。
Public Function PositiveOrNegative(num) as String
If num >= 0 Then
PositiveOrNegative = "Positive"
End If
PositiveOrNegative = "Negative"
End Function
Sub TestFunction()
Debug.Print (PositiveOrNegative(5))
End Sub
TestFunction
輸出:
Negative
即使 TestFunction
輸入是 5
,輸出也是 Negative
,因為程式碼執行在到達 PositiveOrNegative = "Positive"
程式碼行時並沒有停止。
我們需要使用 Exit Function
命令來解決這個問題。
Public Function PositiveOrNegative(num) as String
If num >= 0 Then
PositiveOrNegative = "Positive"
'Exit Function will force the code execution in the current function to stop and return the current value of PositiveOrNegative
Exit Function
End If
PositiveOrNegative = "Negative"
End Function
Sub TestFunction()
Debug.Print (PositiveOrNegative(5))
End Sub
輸出:
Positive
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe