React-Native でテキストを揃える
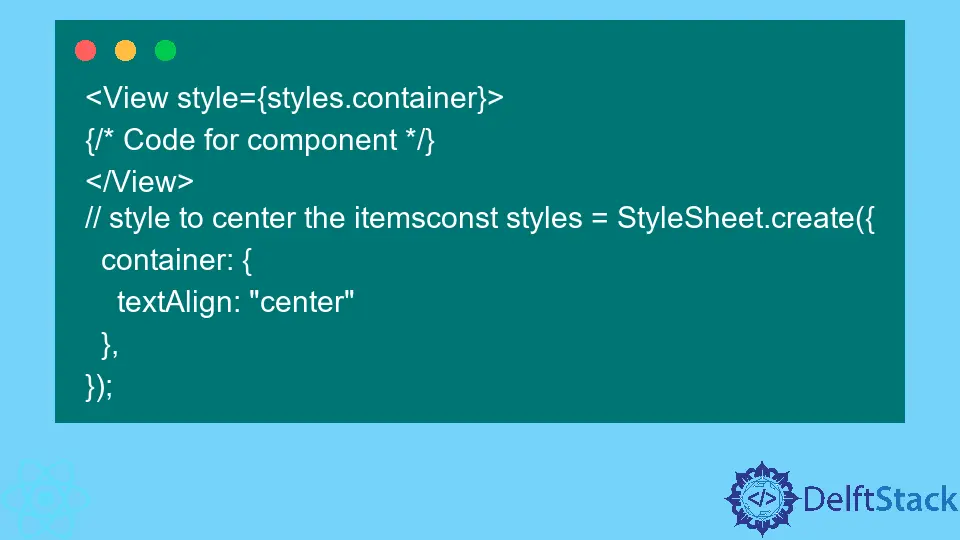
この記事では、React-native でテキストを左、右、中央などのさまざまな位置に配置する方法を学習します。 モバイル アプリケーションの開発中、魅力的な UI を作成するために、開発者が項目を揃える必要があることがよくあります。
React-Native でテキストを中央に揃える
React-native でテキストを整列するには、単純な CSS が必要です。 textAlign: center
を任意のコンポーネントに追加すると、項目が中央に配置されます。
ユーザーは、以下の構文に従って textAlign: center
を任意のコンポーネントに追加できます。
<View style={styles.container}>
{/* Code for component */}
</View>
// style to center the itemsconst styles = StyleSheet.create({
container: {
textAlign: "center"
},
});
以下の例では、いくつかの Text
コンポーネントを作成し、それらを単一の View
コンポーネント内に追加しています。 View
コンポーネントに container
スタイルを適用しました。
container
スタイルでは、textAlign: center
CSS プロパティを追加して、ユーザーが出力で見ることができる View
コンポーネントのコンテンツを中央に配置しました。
コード例:
import { StyleSheet, Text, View } from "react-native";
export default function App() {
return (
<View style={styles.container}>
<Text>Welcome to DelftStack!</Text>
<Text>Welcome to DelftStack!</Text>
<Text>Welcome to DelftStack!</Text>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
width: "100%",
backgroundColor: "#fff",
textAlign: "center"
},
});
出力:
React-Native でテキストを右揃えにする
React-native でテキストを右揃えにする最も簡単な方法は、CSS プロパティ textAlign: Right
を使用することです。 また、テキストを右揃えにする他の方法についても説明します。
以下の例では、Text
コンポーネントに textAlign: right
CSS プロパティを使用しており、ユーザーは出力でテキストが右揃えになっていることを確認できます。
コード例:
import { StyleSheet, Text, View } from "react-native";
export default function App() {
return (
<View>
<Text>Normal Postion of the text.</Text>
<Text style={styles.text}>Text is at Right</Text>
</View>
);
}
const styles = StyleSheet.create({
text: {
textAlign: "right"
},
});
出力:
また、alignSelf
CSS プロパティを使用してテキストを右揃えにすることもできます。 値 flex-end
を alignSelf
CSS プロパティの値として使用すると、テキストが右揃えになります。
alignSelf
CSS プロパティを使用するには、以下の構文を参照してください。
<View>
<Text style={{alignSelf: 'flex-end'}}>This is a Demo Text!</Text>
</View>
ここで、テキストを 1つの行の右と左に揃える方法を学習します。
以下の例では、View
コンポーネントを作成し、flexDirection: row
を CSS プロパティとして追加して、子コンポーネントを 1 行に配置しています。 子コンポーネントでは、要件に従って textAlign
CSS プロパティを追加しました。これにより、一部のテキストを右に、一部を左に 1 行に揃えることができます。
コード例:
import { StyleSheet, Text, View } from "react-native";
export default function App() {
return (
<View style={{flex: 1, flexDirection: 'row'}}>
{/* align left*/}
<View style={{flex: 1}}>
<Text>Left Text</Text>
</View>
{/* align right*/}
<View>
<Text style={{textAlign: 'right'}}>Right Text</Text>
</View>
</View>
);
}
出力:
通常、デフォルトで使用されるため、テキストを左揃えにする必要はありません。 それでも、ユーザーがテキストを左に揃えたい場合は、React Native で textAlign: left
CSS プロパティを使用できます。
この記事では、テキストを左右に揃えることを学びました。 また、1つのコンポーネントまたは 1つの行のテキストを揃えることも学びました。これは、開発者がテキストの後に画像を 1つの行に配置したい場合に非常に便利です。
関連記事 - React Native
- React Native Box Shadow
- React Native setTimeout メソッド
- React Native View onPress
- React Native でグローバル変数を使用する
- React Native でのストレージ
- React Native でのテキスト ラップ