Pandas は列の値を文字列に変換する
Suraj Joshi
2023年1月30日
-
メソッド
apply()
を用いて DataFrame のカラム値のデータ型を文字列に変換する -
すべての DataFrame カラムのデータ型を
applymap()
メソッドを用いてstring
に変換する -
astype()
メソッドを用いて DataFrame のカラム値のデータ型をstring
に変換する
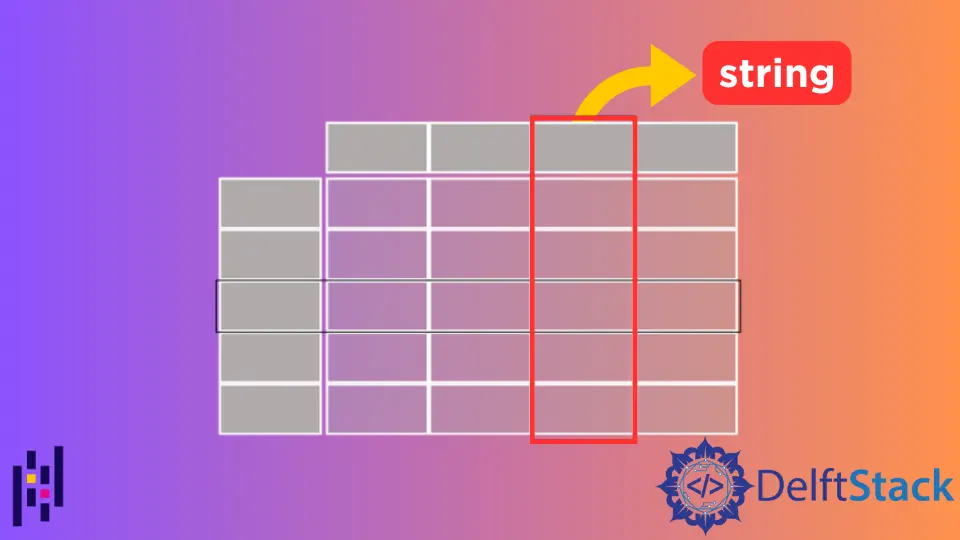
このチュートリアルでは、DataFrame のカラム値のデータ型を文字列に変換する方法について説明します。
import pandas as pd
employees_df = pd.DataFrame(
{
"Name": ["Ayush", "Bikram", "Ceela", "Kusal", "Shanty"],
"Score": [31, 38, 33, 39, 35],
"Age": [33, 34, 38, 45, 37],
}
)
print(employees_df)
出力:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
上記の例で表示された DataFrame を使用して、DataFrame の列値のデータ型を文字列に変換する方法を説明します。
メソッド apply()
を用いて DataFrame のカラム値のデータ型を文字列に変換する
import pandas as pd
employees_df = pd.DataFrame(
{
"Name": ["Ayush", "Bikram", "Ceela", "Kusal", "Shanty"],
"Score": [31, 38, 33, 39, 35],
"Age": [33, 34, 38, 45, 37],
}
)
print("DataFrame before Conversion:")
print(employees_df, "\n")
print("Datatype of columns before conversion:")
print(employees_df.dtypes, "\n")
employees_df["Age"] = employees_df["Age"].apply(str)
print("DataFrame after conversion:")
print(employees_df, "\n")
print("Datatype of columns after conversion:")
print(employees_df.dtypes)
出力:
DataFrame before Conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns before conversion:
Name object
Score int64
Age int64
dtype: object
DataFrame after conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns after conversion:
Name object
Score int64
Age object
dtype: object
この関数は、Age
カラムのデータ型を int64
から string
を表す object
型に変更します。
すべての DataFrame カラムのデータ型を applymap()
メソッドを用いて string
に変換する
DataFrame 内のすべてのカラム値のデータ型を string
型に変更したい場合は、applymap()
メソッドを用いることができます。
import pandas as pd
employees_df = pd.DataFrame(
{
"Name": ["Ayush", "Bikram", "Ceela", "Kusal", "Shanty"],
"Score": [31, 38, 33, 39, 35],
"Age": [33, 34, 38, 45, 37],
}
)
print("DataFrame before Conversion:")
print(employees_df, "\n")
print("Datatype of columns before conversion:")
print(employees_df.dtypes, "\n")
employees_df = employees_df.applymap(str)
print("DataFrame after conversion:")
print(employees_df, "\n")
print("Datatype of columns after conversion:")
print(employees_df.dtypes)
出力:
DataFrame before Conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
zeppy@zeppy-G7-7588:~/test/Week-01/taddaa$ python3 1.py
DataFrame before Conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns before conversion:
Name object
Score int64
Age int64
dtype: object
DataFrame after conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns after conversion:
Name object
Score object
Age object
dtype: object
これは DataFrame のすべてのカラムのデータ型を object
で示される string
型に変換します。
astype()
メソッドを用いて DataFrame のカラム値のデータ型を string
に変換する
import pandas as pd
employees_df = pd.DataFrame(
{
"Name": ["Ayush", "Bikram", "Ceela", "Kusal", "Shanty"],
"Score": [31, 38, 33, 39, 35],
"Age": [33, 34, 38, 45, 37],
}
)
print("DataFrame before Conversion:")
print(employees_df, "\n")
print("Datatype of columns before conversion:")
print(employees_df.dtypes, "\n")
employees_df["Score"] = employees_df["Score"].astype(str)
print("DataFrame after conversion:")
print(employees_df, "\n")
print("Datatype of columns after conversion:")
print(employees_df.dtypes)
出力:
DataFrame before Conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns before conversion:
Name object
Score int64
Age int64
dtype: object
DataFrame after conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns after conversion:
Name object
Score object
Age int64
dtype: object
これは employees_df
Dataframe の Score
カラムのデータ型を string
型に変換します。
著者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn