Pandas 將列值轉換為字串
Suraj Joshi
2023年1月30日
-
使用
apply()
方法將 DataFrame 的列值的資料型別轉換為字串 -
使用
applymap()
方法將所有 DataFrame 列的資料型別轉換為string
-
使用
astype()
方法將 DataFrame 列值的資料型別轉換為string
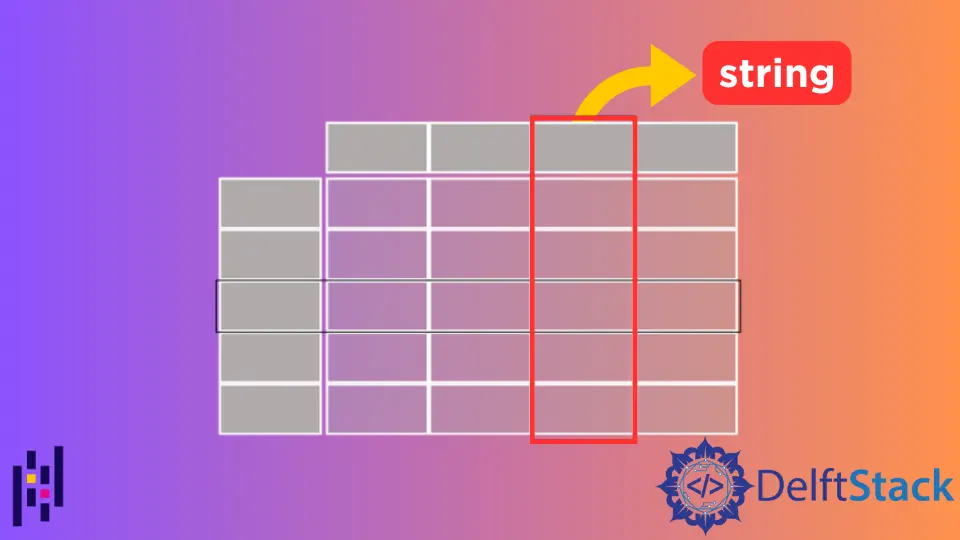
本教程介紹瞭如何將 DataFrame 的列值的資料型別轉換為字串。
import pandas as pd
employees_df = pd.DataFrame(
{
"Name": ["Ayush", "Bikram", "Ceela", "Kusal", "Shanty"],
"Score": [31, 38, 33, 39, 35],
"Age": [33, 34, 38, 45, 37],
}
)
print(employees_df)
輸出:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
我們將使用上面例子中顯示的 DataFrame 來解釋如何將 DataFrame 的列值的資料型別轉換為字串。
使用 apply()
方法將 DataFrame 的列值的資料型別轉換為字串
import pandas as pd
employees_df = pd.DataFrame(
{
"Name": ["Ayush", "Bikram", "Ceela", "Kusal", "Shanty"],
"Score": [31, 38, 33, 39, 35],
"Age": [33, 34, 38, 45, 37],
}
)
print("DataFrame before Conversion:")
print(employees_df, "\n")
print("Datatype of columns before conversion:")
print(employees_df.dtypes, "\n")
employees_df["Age"] = employees_df["Age"].apply(str)
print("DataFrame after conversion:")
print(employees_df, "\n")
print("Datatype of columns after conversion:")
print(employees_df.dtypes)
輸出:
DataFrame before Conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns before conversion:
Name object
Score int64
Age int64
dtype: object
DataFrame after conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns after conversion:
Name object
Score int64
Age object
dtype: object
它將 Age
列的資料型別從 int64
改為代表字串的 object
型別。
使用 applymap()
方法將所有 DataFrame 列的資料型別轉換為 string
如果我們想將 DataFrame 中所有列值的資料型別改為 string
型別,我們可以使用 applymap()
方法。
import pandas as pd
employees_df = pd.DataFrame(
{
"Name": ["Ayush", "Bikram", "Ceela", "Kusal", "Shanty"],
"Score": [31, 38, 33, 39, 35],
"Age": [33, 34, 38, 45, 37],
}
)
print("DataFrame before Conversion:")
print(employees_df, "\n")
print("Datatype of columns before conversion:")
print(employees_df.dtypes, "\n")
employees_df = employees_df.applymap(str)
print("DataFrame after conversion:")
print(employees_df, "\n")
print("Datatype of columns after conversion:")
print(employees_df.dtypes)
輸出:
DataFrame before Conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
zeppy@zeppy-G7-7588:~/test/Week-01/taddaa$ python3 1.py
DataFrame before Conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns before conversion:
Name object
Score int64
Age int64
dtype: object
DataFrame after conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns after conversion:
Name object
Score object
Age object
dtype: object
它將所有 DataFrame 列的資料型別轉換為 string
型別,在輸出中用 object
表示。
使用 astype()
方法將 DataFrame 列值的資料型別轉換為 string
import pandas as pd
employees_df = pd.DataFrame(
{
"Name": ["Ayush", "Bikram", "Ceela", "Kusal", "Shanty"],
"Score": [31, 38, 33, 39, 35],
"Age": [33, 34, 38, 45, 37],
}
)
print("DataFrame before Conversion:")
print(employees_df, "\n")
print("Datatype of columns before conversion:")
print(employees_df.dtypes, "\n")
employees_df["Score"] = employees_df["Score"].astype(str)
print("DataFrame after conversion:")
print(employees_df, "\n")
print("Datatype of columns after conversion:")
print(employees_df.dtypes)
輸出:
DataFrame before Conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns before conversion:
Name object
Score int64
Age int64
dtype: object
DataFrame after conversion:
Name Score Age
0 Ayush 31 33
1 Bikram 38 34
2 Ceela 33 38
3 Kusal 39 45
4 Shanty 35 37
Datatype of columns after conversion:
Name object
Score object
Age int64
dtype: object
它將 employees_df
Dataframe 中 Score
列的資料型別轉換為 string
型別。
作者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn