Pandas の DataFrame 列をリストに変換
-
tolist()
メソッドを使用してDataFrameの列をリストに変換する -
list()
関数を使用してDataFrameの列をリストに変換する -
.values
プロパティを使用してDataFrameの列をリストに変換する - リスト内包表記を使用してDataFrameの列をリストに変換する方法
- 結論
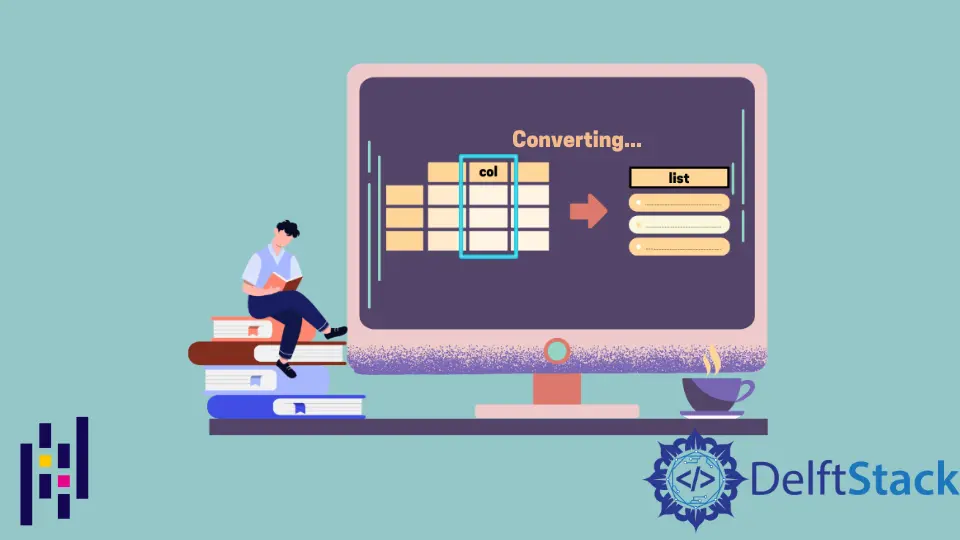
PythonでPandasのDataFrameを扱っていると、DataFrameの列をPythonのリストに変換する必要があることがよくあります。このプロセスは、さまざまなデータの操作や分析のタスクで重要となる場合があります。幸いにも、Pandasはこれを実現するためのいくつかのメソッドを提供しており、必要なデータをリスト形式で抽出するのは簡単です。
この記事では、PandasのDataFrameの列をPythonのリストに変換するためのさまざまな方法を探っていきます。そのために、名前、生年月日(DOB)、給与に関する情報を含むサンプルのDataFrameを使用してこれらの方法をデモンストレーションします。
import pandas as pd
data = {
"Name": ["James", "Michelina", "Marc", "Bob", "Halena"],
"DOB": ["1/1/2014", "2/1/2014", "3/1/2014", "4/1/2014", "4/1/2014"],
"Salary": ["1000", "12000", "36000", "15000", "12000"],
}
df = pd.DataFrame(data, columns=["Name", "DOB", "Salary"])
この変換を行うための4つの異なる方法を探っていきます: tolist()
メソッドを使用する方法、list()
関数を使用する方法、.values
プロパティを使用する方法、およびリスト内包表記を使用する方法です。それぞれの方法には利点がありますので、見ていきましょう。
tolist()
メソッドを使用してDataFrameの列をリストに変換する
PandasのDataFrameの列はPandasのSeries
です。したがって、列をリストに変換する必要がある場合は、Series
のtolist()
メソッドを使用することができます。tolist()
メソッドは、PandasデータフレームのSeries
をリストに変換します。
以下のコードでは、df['DOB']
はDataFrameからDOB
という名前の列であるSeries
、つまり列を返します。
tolist()
メソッドは、Series
をリストに変換します。
import pandas as pd
df = pd.DataFrame(
[
["James", "1/1/2014", "1000"],
["Michelina", "2/1/2014", "12000"],
["Marc", "3/1/2014", "36000"],
["Bob", "4/1/2014", "15000"],
["Halena", "4/1/2014", "12000"],
],
columns=["Name", "DOB", "Salary"],
)
print("Pandas DataFrame:\n\n", df, "\n")
list_of_single_column = df["DOB"].tolist()
print(
"the list of a single column from the dataframe\n",
list_of_single_column,
"\n",
type(list_of_single_column),
)
出力:
Pandas DataFrame:
Name DOB Salary
0 James 1/1/2014 1000
1 Michelina 2/1/2014 12000
2 Marc 3/1/2014 36000
3 Bob 4/1/2014 15000
4 Halena 4/1/2014 12000
the list of a single column from the dataframe
['1/1/2014', '2/1/2014', '3/1/2014', '4/1/2014', '4/1/2014']
<class 'list'>
list()
関数を使用してDataFrameの列をリストに変換する
DataFrameの列をリストに変換するために、list()
関数を使用することもできます。これにはDataFrameをlist()
関数に渡す方法を使用します。
上記と同じデータを使用して、このアプローチを示します。
import pandas as pd
df = pd.DataFrame(
[
["James", "1/1/2014", "1000"],
["Michelina", "2/1/2014", "12000"],
["Marc", "3/1/2014", "36000"],
["Bob", "4/1/2014", "15000"],
["Halena", "4/1/2014", "12000"],
],
columns=["Name", "DOB", "Salary"],
)
print("Pandas DataFrame:\n\n", df, "\n")
list_of_single_column = list(df["DOB"])
print(
"the list of a single column from the dataframe\n",
list_of_single_column,
"\n",
type(list_of_single_column),
)
出力:
Pandas DataFrame:
Name DOB Salary
0 James 1/1/2014 1000
1 Michelina 2/1/2014 12000
2 Marc 3/1/2014 36000
3 Bob 4/1/2014 15000
4 Halena 4/1/2014 12000
the list of a single column from the dataframe
['1/1/2014', '2/1/2014', '3/1/2014', '4/1/2014', '4/1/2014']
<class 'list'>
.values
プロパティを使用してDataFrameの列をリストに変換する
これを達成する別の方法は、.values
プロパティを使用することです。
.values
プロパティを使用して、このDataFrameの ‘Salary’ 列をPythonのリストに変換する手順を見ていきましょう。
Pandas Seriesの .values
プロパティはデータのNumPy配列表現を返します。Pythonのリストに変換するには、.tolist()
メソッドを使用できます。以下にその方法を示します:
salary_list = df["Salary"].values.tolist()
以下は完全なコードです:
import pandas as pd
data = {
"Name": ["James", "Michelina", "Marc", "Bob", "Halena"],
"DOB": ["1/1/2014", "2/1/2014", "3/1/2014", "4/1/2014", "4/1/2014"],
"Salary": ["1000", "12000", "36000", "15000", "12000"],
}
df = pd.DataFrame(data, columns=["Name", "DOB", "Salary"])
# Convert the 'Salary' column to a list using .values
salary_list = df["Salary"].values.tolist()
print(salary_list)
出力:
['1000', '12000', '36000', '15000', '12000']
このコードを実行すると、DataFrameのSalary
列がPythonのリストに変換され、その結果がsalary_list
変数に格納されます。
リスト内包表記を使用してDataFrameの列をリストに変換する方法
リスト内包表記は、Pythonでリストを簡潔かつ効率的に作成する方法です。PandasのDataFrameの列をリストに変換するために、リスト内包表記を使用する方法は次のコードに従うことができます。
# Using list comprehension to convert the 'Salary' column to a list
salary_list = [salary for salary in df["Salary"]]
以下は完全なコードです:
import pandas as pd
data = {
"Name": ["James", "Michelina", "Marc", "Bob", "Halena"],
"DOB": ["1/1/2014", "2/1/2014", "3/1/2014", "4/1/2014", "4/1/2014"],
"Salary": [1000, 12000, 36000, 15000, 12000],
}
df = pd.DataFrame(data, columns=["Name", "DOB", "Salary"])
# Using list comprehension to convert the 'Salary' column to a list
salary_list = [salary for salary in df["Salary"]]
print(salary_list)
出力:
[1000, 12000, 36000, 15000, 12000]
結論
この記事では、PandasのDataFrameの列をPythonのリストに変換するための4つの異なる方法を紹介しました:tolist()
メソッド、list()
関数、.values
プロパティ、およびリスト内包表記を使用する方法です。各方法にはそれぞれ利点があり、使用する方法は特定のユースケースとコーディングスタイルに基づいて選択することができます。
tolist()
メソッドのシンプルさ、list()
関数のPythonの標準的なアプローチ、.values
プロパティの効率性、リスト内包表記の読みやすさなど、PandasはDataFrameの列をPythonのリストにシームレスに変換するための複数のオプションを提供しています。
関連記事 - Pandas DataFrame Column
- Pandas DataFrame の列ヘッダーをリストとして取得する方法
- Pandas DataFrame 列を削除する方法
- Pandas で DataFrame 列を日時に変換する方法
- Pandas 列の合計を取得する方法
- Pandas DataFrame 列の順序を変更する方法
- Pandas で DataFrame 列を文字列に変換する方法