Pandas DataFrame 열을 목록으로 변환
-
tolist()
메서드를 사용하여 데이터프레임 열을 리스트로 변환하기 -
list()
함수를 사용하여 데이터프레임 열을 리스트로 변환하기 -
.values
속성을 사용하여 데이터프레임 열을 리스트로 변환하기 - 리스트 내성을 사용하여 데이터프레임 열을 리스트로 변환하기
- 결론
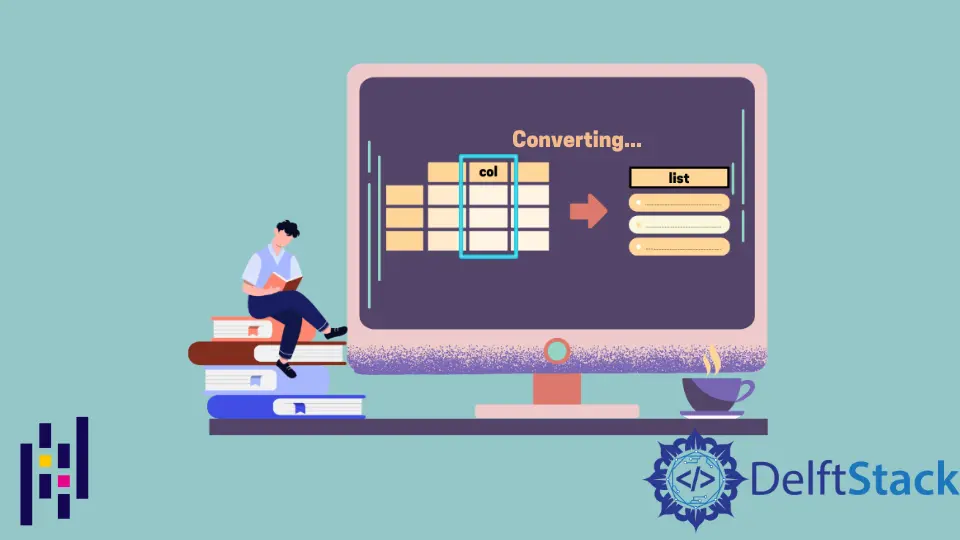
파이썬에서 Pandas 데이터프레임을 사용할 때에는 종종 데이터프레임의 열을 파이썬 리스트로 변환해야 할 때가 많습니다. 이 과정은 다양한 데이터 조작과 분석 작업에 필수적일 수 있습니다. 다행히도 Pandas는 이를 위해 여러 가지 방법을 제공하여 필요한 데이터를 리스트 형식으로 추출하기 쉽게 해줍니다.
이 글에서는 Pandas 데이터프레임 열을 파이썬 리스트로 변환하는 다양한 방법을 살펴보겠습니다. 이름, 생년월일(DOB) 및 급여에 대한 정보를 포함하는 샘플 데이터프레임을 사용하여 이러한 방법을 설명하겠습니다.
import pandas as pd
data = {
"Name": ["James", "Michelina", "Marc", "Bob", "Halena"],
"DOB": ["1/1/2014", "2/1/2014", "3/1/2014", "4/1/2014", "4/1/2014"],
"Salary": ["1000", "12000", "36000", "15000", "12000"],
}
df = pd.DataFrame(data, columns=["Name", "DOB", "Salary"])
이 글에서는 데이터프레임 열을 리스트로 변환하기 위해 tolist()
메서드, list()
함수, .values
속성 및 리스트 컴프리헨션을 사용하는 네 가지 다른 방법을 살펴보겠습니다. 각 방법은 각자의 장점을 가지고 있으므로 한 번 알아보겠습니다.
tolist()
메서드를 사용하여 데이터프레임 열을 리스트로 변환하기
Pandas 데이터프레임의 열은 Pandas Series
입니다. 따라서 열을 리스트로 변환해야 할 경우, 데이터프레임의 Series
에서 tolist()
메서드를 사용할 수 있습니다. tolist()
는 Pandas 데이터프레임의 Series
를 리스트로 변환합니다.
아래 코드에서 df['DOB']
는 DataFrame에서 DOB
라는 이름의 열 또는 Series
를 반환합니다.
tolist()
메서드는 Series
를 리스트로 변환합니다.
import pandas as pd
df = pd.DataFrame(
[
["James", "1/1/2014", "1000"],
["Michelina", "2/1/2014", "12000"],
["Marc", "3/1/2014", "36000"],
["Bob", "4/1/2014", "15000"],
["Halena", "4/1/2014", "12000"],
],
columns=["Name", "DOB", "Salary"],
)
print("Pandas DataFrame:\n\n", df, "\n")
list_of_single_column = df["DOB"].tolist()
print(
"the list of a single column from the dataframe\n",
list_of_single_column,
"\n",
type(list_of_single_column),
)
출력:
Pandas DataFrame:
Name DOB Salary
0 James 1/1/2014 1000
1 Michelina 2/1/2014 12000
2 Marc 3/1/2014 36000
3 Bob 4/1/2014 15000
4 Halena 4/1/2014 12000
the list of a single column from the dataframe
['1/1/2014', '2/1/2014', '3/1/2014', '4/1/2014', '4/1/2014']
<class 'list'>
list()
함수를 사용하여 데이터프레임 열을 리스트로 변환하기
데이터프레임의 열을 리스트로 변환하기 위해 list()
함수를 사용할 수도 있습니다. 이를 위해 데이터프레임을 list()
함수에 전달하면 됩니다.
위에서 사용한 데이터를 동일하게 사용하여 이 접근 방법을 설명하겠습니다.
import pandas as pd
df = pd.DataFrame(
[
["James", "1/1/2014", "1000"],
["Michelina", "2/1/2014", "12000"],
["Marc", "3/1/2014", "36000"],
["Bob", "4/1/2014", "15000"],
["Halena", "4/1/2014", "12000"],
],
columns=["Name", "DOB", "Salary"],
)
print("Pandas DataFrame:\n\n", df, "\n")
list_of_single_column = list(df["DOB"])
print(
"the list of a single column from the dataframe\n",
list_of_single_column,
"\n",
type(list_of_single_column),
)
출력:
Pandas DataFrame:
Name DOB Salary
0 James 1/1/2014 1000
1 Michelina 2/1/2014 12000
2 Marc 3/1/2014 36000
3 Bob 4/1/2014 15000
4 Halena 4/1/2014 12000
the list of a single column from the dataframe
['1/1/2014', '2/1/2014', '3/1/2014', '4/1/2014', '4/1/2014']
<class 'list'>
.values
속성을 사용하여 데이터프레임 열을 리스트로 변환하기
이를 위해 다른 방법은 .values
속성을 사용하는 것입니다.
.values
속성을 사용하여 이 데이터프레임의 Salary
열을 Python 리스트로 변환하는 단계를 살펴보겠습니다.
Pandas Series의 .values
속성은 데이터의 NumPy 배열 표현을 반환합니다. 이를 Python 리스트로 변환하려면 .tolist()
메서드를 사용할 수 있습니다. 다음과 같이 할 수 있습니다.
salary_list = df["Salary"].values.tolist()
전체 코드는 다음과 같습니다.
import pandas as pd
data = {
"Name": ["James", "Michelina", "Marc", "Bob", "Halena"],
"DOB": ["1/1/2014", "2/1/2014", "3/1/2014", "4/1/2014", "4/1/2014"],
"Salary": ["1000", "12000", "36000", "15000", "12000"],
}
df = pd.DataFrame(data, columns=["Name", "DOB", "Salary"])
# Convert the 'Salary' column to a list using .values
salary_list = df["Salary"].values.tolist()
print(salary_list)
출력:
['1000', '12000', '36000', '15000', '12000']
이 코드를 실행한 후에 데이터프레임의 ‘Salary’ 열은 파이썬 리스트로 변환되어 salary_list
변수에 저장됩니다.
리스트 내성을 사용하여 데이터프레임 열을 리스트로 변환하기
리스트 내성은 파이썬에서 리스트를 생성하는 간결하고 효율적인 방법입니다. 데이터프레임의 열을 리스트로 변환하기 위해 리스트 내성을 사용하는 방법은 아래 코드를 따르면 됩니다.
# Using list comprehension to convert the 'Salary' column to a list
salary_list = [salary for salary in df["Salary"]]
전체 코드는 다음과 같습니다:
import pandas as pd
data = {
"Name": ["James", "Michelina", "Marc", "Bob", "Halena"],
"DOB": ["1/1/2014", "2/1/2014", "3/1/2014", "4/1/2014", "4/1/2014"],
"Salary": [1000, 12000, 36000, 15000, 12000],
}
df = pd.DataFrame(data, columns=["Name", "DOB", "Salary"])
# Using list comprehension to convert the 'Salary' column to a list
salary_list = [salary for salary in df["Salary"]]
print(salary_list)
결과:
[1000, 12000, 36000, 15000, 12000]
결론
이 글에서는 데이터프레임의 열을 파이썬 리스트로 변환하는 네 가지 다른 방법을 알아보았습니다: tolist()
메소드를 사용하는 방법, list()
함수를 사용하는 방법, .values
속성을 사용하는 방법, 그리고 리스트 내성을 사용하는 방법입니다. 각각의 방법에는 장단점이 있으며 특정 사용 사례와 코딩 스타일에 따라 선택할 수 있습니다.
tolist()
메소드의 간결함, list()
함수의 표준 파이썬 스타일, .values
속성의 효율성 또는 리스트 내성의 가독성 중 어떤 것을 선호하든, Pandas는 데이터프레임 열을 파이썬 리스트로 손쉽게 변환할 수 있는 여러 가지 옵션을 제공합니다.
관련 문장 - Pandas DataFrame Column
- Pandas DataFrame 열 헤더를 목록으로 가져 오는 방법
- Pandas DataFrame 열을 삭제하는 방법
- Pandas 에서 DataFrame 열을 Datetime 으로 변환하는 방법
- Pandas 열의 합계를 얻는 방법
- Pandas DataFrame 열의 순서를 변경하는 방법
- Pandas에서 DataFrame 열을 문자열로 변환하는 방법