Pandas データフレームを Excel ファイルにエクスポートする
-
to_excel()
関数を使用して、Pandas の DataFrame を Excel ファイルにエクスポートする -
ExcelWriter()
メソッドを使用して Pandas の DataFrame をエクスポートする - 複数の Pandas DataFrame を複数の Excel シートにエクスポート
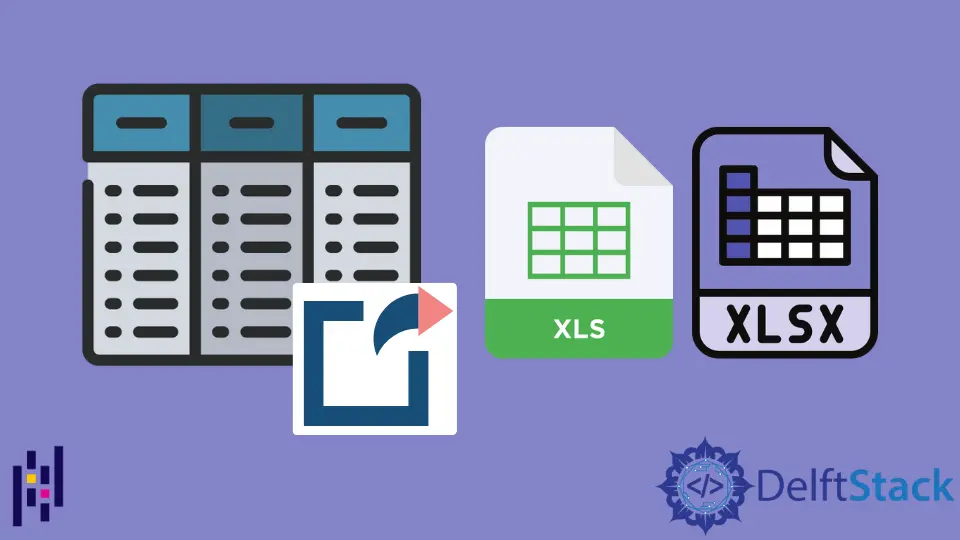
このチュートリアルでは、2つの異なる方法を使用して Pandas の DataFrame を Excel ファイルにエクスポートする方法を示します。最初の方法は、ファイル名を指定して to_excel()
関数を呼び出して、Pandas の DataFrame を Excel ファイルにエクスポートすることです。この記事で説明するもう 1つのメソッドは、ExcelWriter()
メソッドです。このメソッドは、オブジェクトを Excel シートに書き込み、to_excel
関数を使用してそれらを Excel ファイルにエクスポートします。
このガイドでは、ExcelWriter()
メソッドを使用して複数の Pandas DataFrame を複数の Excel シートに追加する方法についても説明します。さらに、各方法を詳細に説明するために、システムで複数の例を実行しました。
to_excel()
関数を使用して、Pandas の DataFrame を Excel ファイルにエクスポートする
dataframe.to_excel()
関数を使用して Pandas の DataFrame
を Excel シートにエクスポートすると、オブジェクトが Excel シートに直接書き込まれます。このメソッドを実装するには、DataFrame を作成してから、Excel ファイルの名前を指定します。ここで、dataframe.to_excel()
関数を使用して、Pandas の DataFrame
を Excel ファイルにエクスポートします。
次の例では、Products_ID
、Product_Names
、Product_Prices
、Product_Sales
列を含む sales_record
という名前の DataFrame
を作成しました。その後、Excel ファイル ProductSales_sheet.xlsx
の名前を指定しました。sales_record.to_excel()
メソッドを使用して、すべてのデータを Excel シートに保存しました。
以下のサンプルコードを参照してください。
import pandas as pd
# DataFrame Creation
sales_record = pd.DataFrame(
{
"Products_ID": {
0: 101,
1: 102,
2: 103,
3: 104,
4: 105,
5: 106,
6: 107,
7: 108,
8: 109,
},
"Product_Names": {
0: "Mosuse",
1: "Keyboard",
2: "Headphones",
3: "CPU",
4: "Flash Drives",
5: "Tablets",
6: "Android Box",
7: "LCD",
8: "OTG Cables",
},
"Product_Prices": {
0: 700,
1: 800,
2: 200,
3: 2000,
4: 100,
5: 1500,
6: 1800,
7: 1300,
8: 90,
},
"Product_Sales": {0: 5, 1: 13, 2: 50, 3: 4, 4: 100, 5: 50, 6: 6, 7: 1, 8: 50},
}
)
# Specify the name of the excel file
file_name = "ProductSales_sheet.xlsx"
# saving the excelsheet
sales_record.to_excel(file_name)
print("Sales record successfully exported into Excel File")
出力:
Sales record successfully exported into Excel File
上記のソースを実行すると、Excel ファイル ProductSales_sheet.xlsx
が現在実行中のプロジェクトのフォルダーに保存されます。
ExcelWriter()
メソッドを使用して Pandas の DataFrame をエクスポートする
Excelwrite()
メソッドは、Pandas の DataFrame を Excel ファイルにエクスポートする場合にも役立ちます。まず、Excewriter()
メソッドを使用してオブジェクトを Excel シートに書き込み、次に dataframe.to_excel()
関数を使用して DataFrame
を Excel ファイルにエクスポートできます。
以下のサンプルコードを参照してください。
import pandas as pd
students_data = pd.DataFrame(
{
"Student": ["Samreena", "Ali", "Sara", "Amna", "Eva"],
"marks": [800, 830, 740, 910, 1090],
"Grades": ["B+", "B+", "B", "A", "A+"],
}
)
# writing to Excel
student_result = pd.ExcelWriter("StudentResult.xlsx")
# write students data to excel
students_data.to_excel(student_result)
# save the students result excel
student_result.save()
print("Students data is successfully written into Excel File")
出力:
Students data is successfully written into Excel File
複数の Pandas DataFrame を複数の Excel シートにエクスポート
上記の方法では、単一の Pandas DataFrame を Excel シートにエクスポートしました。ただし、この方法を使用すると、複数の Pandas DataFrame を複数の Excel シートにエクスポートできます。
複数の DataFrame を別々に複数の Excel シートにエクスポートした次の例を参照してください。
import pandas as pd
import numpy as np
import xlsxwriter
# Creating records or dataset using dictionary
Science_subject = {
"Name": ["Ali", "Umar", "Mirha", "Asif", "Samreena"],
"Roll no": ["101", "102", "103", "104", "105"],
"science": ["88", "60", "66", "94", "40"],
}
Computer_subject = {
"Name": ["Ali", "Umar", "Mirha", "Asif", "Samreena"],
"Roll no": ["101", "102", "103", "104", "105"],
"computer_science": ["73", "63", "50", "95", "73"],
}
Art_subject = {
"Name": ["Ali", "Umar", "Mirha", "Asif", "Samreena"],
"Roll no": ["101", "102", "103", "104", "105"],
"fine_arts": ["95", "63", "50", "60", "93"],
}
# Dictionary to Dataframe conversion
dataframe1 = pd.DataFrame(Science_subject)
dataframe2 = pd.DataFrame(Computer_subject)
dataframe3 = pd.DataFrame(Art_subject)
with pd.ExcelWriter("studentsresult.xlsx", engine="xlsxwriter") as writer:
dataframe1.to_excel(writer, sheet_name="Science")
dataframe2.to_excel(writer, sheet_name="Computer")
dataframe3.to_excel(writer, sheet_name="Arts")
print("Please check out subject-wise studentsresult.xlsx file.")
出力:
Please check out subject-wise studentsresult.xlsx file.