Java での行列乗算
Shubham Vora
2023年10月12日
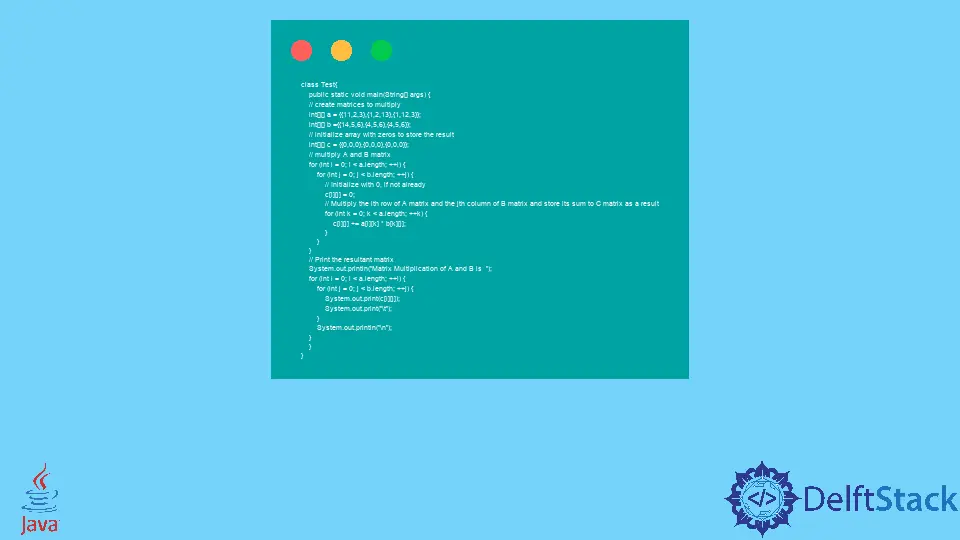
この記事では、Java で 2つの行列を乗算する方法を学習します。
Java で 2つの行列を乗算する
乗算演算子と加算演算子を使用して、2つの行列を乗算しています。 ユーザーは、次の手順に従って 2つの行列を乗算する必要があります。
-
2つの行列を作成し、値で初期化します。
-
出力を格納する行列を作成し、ゼロで初期化します。
-
ネストされたループを使用して、最初の行列の各行を 2 番目の行列の各列で乗算します。 その合計を出力行列に格納します。
コード例:
class Test {
public static void main(String[] args) {
// create matrices to multiply
int[][] a = {{11, 2, 3}, {1, 2, 13}, {1, 12, 3}};
int[][] b = {{14, 5, 6}, {4, 5, 6}, {4, 5, 6}};
// initialize array with zeros to store the result
int[][] c = {{0, 0, 0}, {0, 0, 0}, {0, 0, 0}};
// multiply A and B matrix
for (int i = 0; i < a.length; ++i) {
for (int j = 0; j < b.length; ++j) {
// Initialize with 0, if not already
c[i][j] = 0;
// Multiply the ith row of A matrix and the jth column of B matrix and store its sum to C
// matrix as a result
for (int k = 0; k < a.length; ++k) {
c[i][j] += a[i][k] * b[k][j];
}
}
}
// Print the resultant matrix
System.out.println("Matrix Multiplication of A and B is ");
for (int i = 0; i < a.length; ++i) {
for (int j = 0; j < b.length; ++j) {
System.out.print(c[i][j]);
System.out.print("\t");
}
System.out.println("\n");
}
}
}
出力:
Matrix Multiplication of A and B is
174 80 96
74 80 96
74 80 96
行列乗算の時間計算量
3つのネストされたループを使用しているため、行列乗算の時間計算量は O(M^3)
です。
行列乗算の空間複雑度
行列乗算アルゴリズムの空間計算量は O(M^2)
です。
著者: Shubham Vora