Matrixmultiplikation in Java
Shubham Vora
12 Oktober 2023
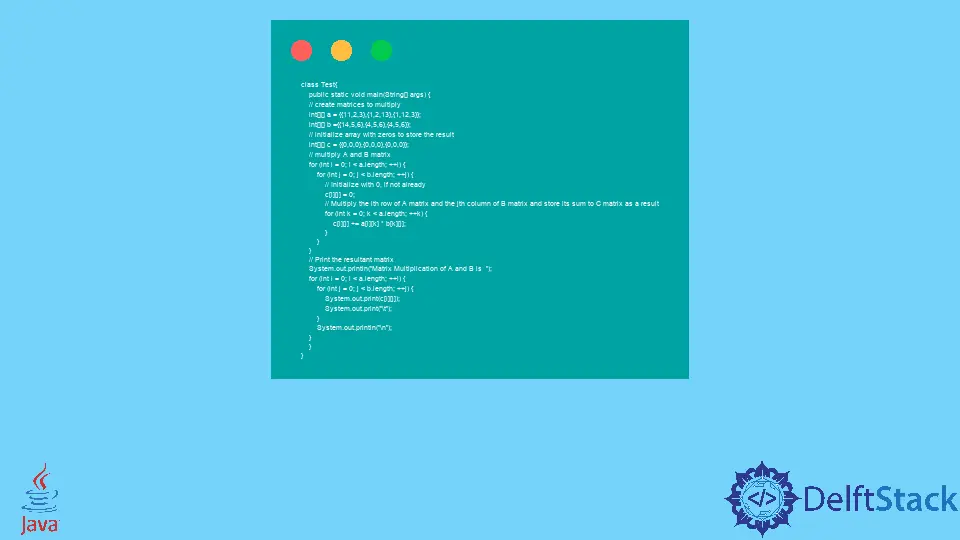
In diesem Artikel lernen wir, die beiden Matrizen in Java zu multiplizieren.
Multiplizieren Sie zwei Matrizen in Java
Wir verwenden die Multiplikations- und Additionsoperatoren, um zwei Matrizen zu multiplizieren. Benutzer sollten die folgenden Schritte ausführen, um zwei Matrizen zu multiplizieren.
-
Erstellen Sie zwei Matrizen und initialisieren Sie sie mit Werten.
-
Erstellen Sie eine Matrix zum Speichern der Ausgabe und initialisieren Sie sie mit Nullen.
-
Verwenden Sie die verschachtelte Schleife, um jede Zeile der 1. Matrix mit jeder Spalte der 2. Matrix zu multiplizieren. Speichern Sie die Summe davon in der Ausgangsmatrix.
Beispielcode:
class Test {
public static void main(String[] args) {
// create matrices to multiply
int[][] a = {{11, 2, 3}, {1, 2, 13}, {1, 12, 3}};
int[][] b = {{14, 5, 6}, {4, 5, 6}, {4, 5, 6}};
// initialize array with zeros to store the result
int[][] c = {{0, 0, 0}, {0, 0, 0}, {0, 0, 0}};
// multiply A and B matrix
for (int i = 0; i < a.length; ++i) {
for (int j = 0; j < b.length; ++j) {
// Initialize with 0, if not already
c[i][j] = 0;
// Multiply the ith row of A matrix and the jth column of B matrix and store its sum to C
// matrix as a result
for (int k = 0; k < a.length; ++k) {
c[i][j] += a[i][k] * b[k][j];
}
}
}
// Print the resultant matrix
System.out.println("Matrix Multiplication of A and B is ");
for (int i = 0; i < a.length; ++i) {
for (int j = 0; j < b.length; ++j) {
System.out.print(c[i][j]);
System.out.print("\t");
}
System.out.println("\n");
}
}
}
Ausgang:
Matrix Multiplication of A and B is
174 80 96
74 80 96
74 80 96
Zeitkomplexität der Matrixmultiplikation
Die Zeitkomplexität für die Matrixmultiplikation ist O(M^3)
, da wir 3 verschachtelte Schleifen verwenden.
Raumkomplexität der Matrixmultiplikation
Die Raumkomplexität für den Matrixmultiplikationsalgorithmus ist O(M^2)
.
Autor: Shubham Vora