Multiplicación de matrices en Java
Shubham Vora
12 octubre 2023
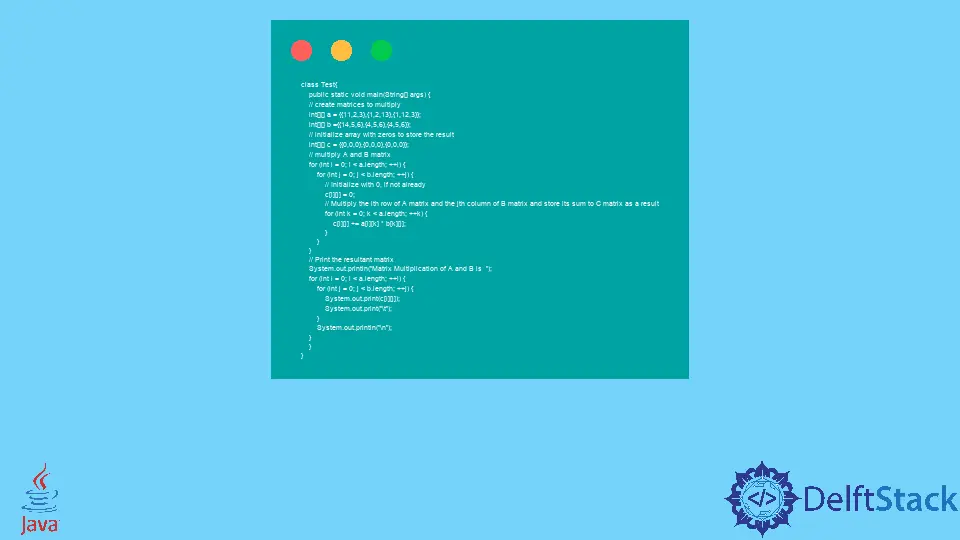
En este artículo, aprenderemos a multiplicar las dos matrices en Java.
Multiplicar dos matrices en Java
Estamos usando los operadores de multiplicación y suma para multiplicar dos matrices. Los usuarios deben seguir los pasos a continuación para multiplicar dos matrices.
-
Cree dos matrices e inicialícelas con valores.
-
Cree una matriz para almacenar la salida e inicialícela con ceros.
-
Use el bucle anidado para multiplicar cada fila de la primera matriz con cada columna de la segunda matriz. Almacene la suma de la misma en la matriz de salida.
Código de ejemplo:
class Test {
public static void main(String[] args) {
// create matrices to multiply
int[][] a = {{11, 2, 3}, {1, 2, 13}, {1, 12, 3}};
int[][] b = {{14, 5, 6}, {4, 5, 6}, {4, 5, 6}};
// initialize array with zeros to store the result
int[][] c = {{0, 0, 0}, {0, 0, 0}, {0, 0, 0}};
// multiply A and B matrix
for (int i = 0; i < a.length; ++i) {
for (int j = 0; j < b.length; ++j) {
// Initialize with 0, if not already
c[i][j] = 0;
// Multiply the ith row of A matrix and the jth column of B matrix and store its sum to C
// matrix as a result
for (int k = 0; k < a.length; ++k) {
c[i][j] += a[i][k] * b[k][j];
}
}
}
// Print the resultant matrix
System.out.println("Matrix Multiplication of A and B is ");
for (int i = 0; i < a.length; ++i) {
for (int j = 0; j < b.length; ++j) {
System.out.print(c[i][j]);
System.out.print("\t");
}
System.out.println("\n");
}
}
}
Producción :
Matrix Multiplication of A and B is
174 80 96
74 80 96
74 80 96
Complejidad temporal de la multiplicación de matrices
La complejidad de tiempo para la multiplicación de matrices es O(M^3)
, ya que estamos usando 3 bucles anidados.
Complejidad espacial de la multiplicación de matrices
La complejidad del espacio para el algoritmo de multiplicación de matrices es O(M^2)
.
Autor: Shubham Vora