Java의 행렬 곱셈
Shubham Vora
2023년10월12일
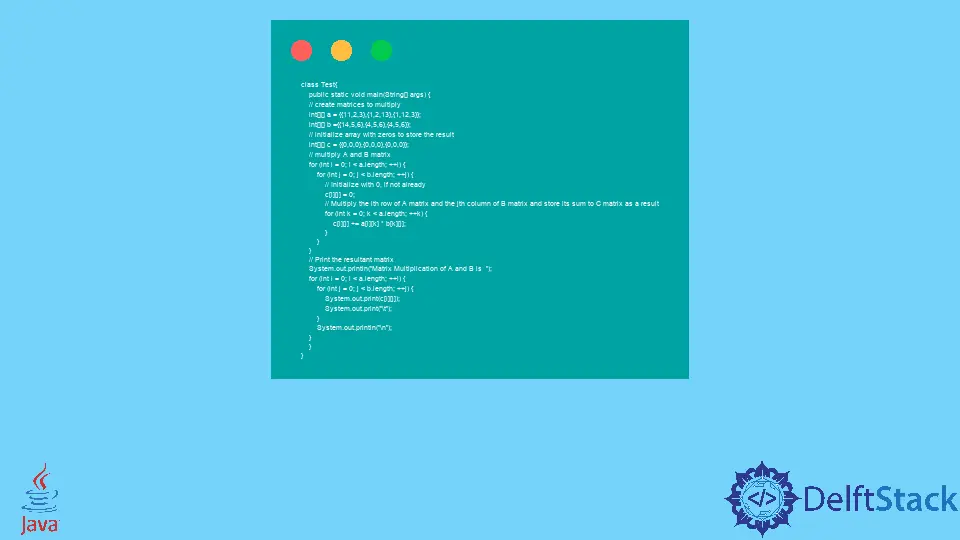
이 기사에서는 Java에서 두 행렬을 곱하는 방법을 배웁니다.
Java에서 두 개의 행렬 곱하기
곱셈 및 덧셈 연산자를 사용하여 두 행렬을 곱합니다. 사용자는 아래 단계에 따라 두 행렬을 곱해야 합니다.
-
두 개의 행렬을 만들고 값으로 초기화합니다.
-
출력을 저장할 행렬을 만들고 0으로 초기화합니다.
-
중첩 루프를 사용하여 첫 번째 행렬의 각 행을 두 번째 행렬의 각 열과 곱합니다. 그 합을 출력 행렬에 저장합니다.
예제 코드:
class Test {
public static void main(String[] args) {
// create matrices to multiply
int[][] a = {{11, 2, 3}, {1, 2, 13}, {1, 12, 3}};
int[][] b = {{14, 5, 6}, {4, 5, 6}, {4, 5, 6}};
// initialize array with zeros to store the result
int[][] c = {{0, 0, 0}, {0, 0, 0}, {0, 0, 0}};
// multiply A and B matrix
for (int i = 0; i < a.length; ++i) {
for (int j = 0; j < b.length; ++j) {
// Initialize with 0, if not already
c[i][j] = 0;
// Multiply the ith row of A matrix and the jth column of B matrix and store its sum to C
// matrix as a result
for (int k = 0; k < a.length; ++k) {
c[i][j] += a[i][k] * b[k][j];
}
}
}
// Print the resultant matrix
System.out.println("Matrix Multiplication of A and B is ");
for (int i = 0; i < a.length; ++i) {
for (int j = 0; j < b.length; ++j) {
System.out.print(c[i][j]);
System.out.print("\t");
}
System.out.println("\n");
}
}
}
출력:
Matrix Multiplication of A and B is
174 80 96
74 80 96
74 80 96
행렬 곱셈의 시간 복잡도
행렬 곱셈의 시간 복잡도는 3개의 중첩 루프를 사용하므로 O(M^3)
입니다.
행렬 곱셈의 공간 복잡도
행렬 곱셈 알고리즘의 공간 복잡도는 O(M^2)
입니다.
작가: Shubham Vora