JavaFX リージョンとペイン
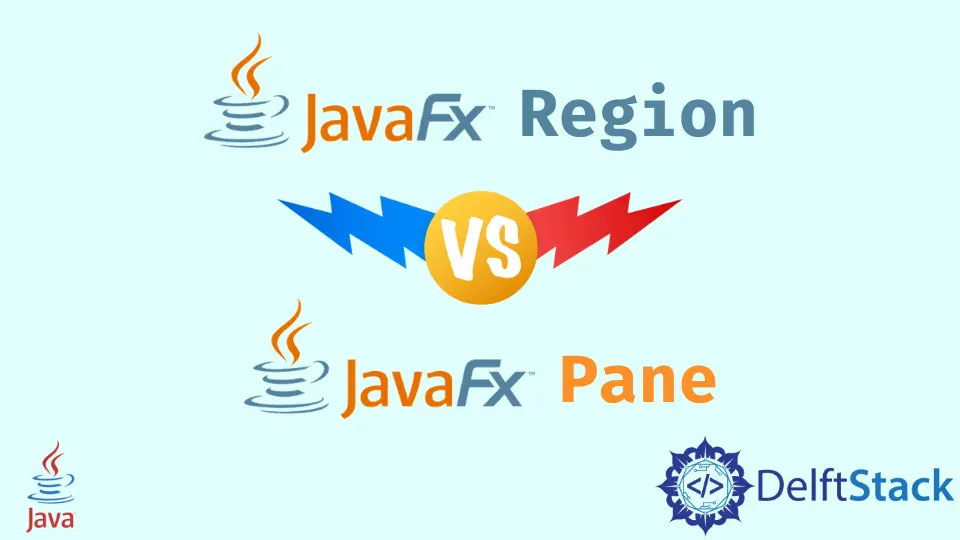
リージョンとペインは、サイズ変更可能な子ノードのサイズを適切なサイズに変更するために使用され、再配置されません。このチュートリアルでは、JavaFX の Region
と Pane
の違いを示します。
JavaFX の Region
JavaFX Region
クラスは、すべての JavaFX レイアウトペインの基本クラスとして使用できます。リージョンを拡張するために使用されるすべての JavaFX レイアウトクラスによって共有されるいくつかのプロパティを提供します。
JavaFX には Pane
, Control
, Chart
, Axis
のサブクラスである多くのクラスがあります。これらの 4つおよび他のすべてのクラスは、Region
クラスのサブクラスです。それらはすべて Region
と同じプロパティを持ちます。
リージョンのプロパティは次のとおりです。
Background
Content Area
Padding
Borders
Margin
Region Insets
JavaFX の Pane
JavaFX Pane
は Region
のサブクラスです。Pane
は、他の多くの JavaFX コンポーネントを格納してレイアウトできるレイアウトコンテナです。
レイアウトアルゴリズムは提供しませんが、その場所に含まれるコンポーネントを表示します。これは、コンポーネントに適しています。Pane
は、子コンポーネントによって指定された layoutX
と layoutY
を使用して、それらを表示する場所を決定します。
Pane
は、JavaFX Region
クラスのすべてのプロパティを継承します。これはサブクラスであるためです。そのため、背景、コンテンツエリア、パディング、境界線なども Pane
に使用できます。
JavaFX のリージョンとペインの比較
Region
と Pane
の両方を使用して、サイズ変更可能な子ノードのサイズを適切なサイズに変更します。彼らは決してそれらを再配置しません。
Region
は、子ノードを持つスーパークラスです。Pane
は、子ノードを持つRegion
クラスのサブクラスです。Region
では、パブリック API を介して子ノードを操作することはできません。一方、Pane
はパブリック API が子ノードを操作できるようにします。Region.getChildren()
は保護されていますが、Pane.getChildren
は保護されたメソッドではありません。Region
はコンポーネント開発者専用です。そのため、API ユーザーがPane
、Hbox
などの子を操作することを許可するかどうかを選択できます。一方、Pane
はそのような許可を提供しません。API ユーザーは、子ノードを直接操作できます。
Region
と Pane
の両方の例を試してみましょう。
地域
の例:
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TextArea;
import javafx.scene.layout.Region;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class JavaFX_Reigon extends Application {
public void start(Stage Label_Stage) {
String Label_Text = "DelftStack is a resource for everyone interested in programming.";
// Create a Label
Label Demo_Label = new Label(Label_Text);
// wrap the label
Demo_Label.setWrapText(true);
// Set the maximum width of the label
Demo_Label.setMaxWidth(300);
// Set the position of the label
Demo_Label.setTranslateX(30);
Demo_Label.setTranslateY(30);
// Create a Region
Region Label_Root = new Region();
// Add Children to region which will throw an error
Label_Root.getChildren().add(Demo_Label);
// Set the stage
Scene Label_Scene = new Scene(Label_Root, 595, 150, Color.BEIGE);
Label_Stage.setTitle("Region Example");
Label_Stage.setScene(Label_Scene);
Label_Stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
上記のコードは、テキストをラベルでラップするために使用されます。ご覧のとおり、Region
に子ノードを追加しましたが、これは不可能なので、このコードはエラーをスローするはずです。
出力を参照してください:
Exception in Application start method
java.lang.reflect.InvocationTargetException
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:77)
at java.base/jdk.internal.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.base/java.lang.reflect.Method.invoke(Method.java:568)
at javafx.graphics@18.0.1/com.sun.javafx.application.LauncherImpl.launchApplicationWithArgs(LauncherImpl.java:465)
at javafx.graphics@18.0.1/com.sun.javafx.application.LauncherImpl.launchApplication(LauncherImpl.java:364)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at java.base/jdk.internal.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:77)
at java.base/jdk.internal.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.base/java.lang.reflect.Method.invoke(Method.java:568)
at java.base/sun.launcher.LauncherHelper$FXHelper.main(LauncherHelper.java:1071)
Pane
の同じ例を試してみましょう。ここでは、ペインに子ノードを追加できます。例を参照してください:
package delftstack;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.TextArea;
import javafx.scene.layout.Pane;
import javafx.scene.layout.Region;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class JavaFX_Reigon extends Application {
public void start(Stage Label_Stage) {
String Label_Text = "DelftStack is a resource for everyone interested in programming.";
// Create a Label
Label Demo_Label = new Label(Label_Text);
// wrap the label
Demo_Label.setWrapText(true);
// Set the maximum width of the label
Demo_Label.setMaxWidth(300);
// Set the position of the label
Demo_Label.setTranslateX(30);
Demo_Label.setTranslateY(30);
// Create a Pane
Pane Label_Root = new Pane();
// Add Children to Pane which will work properly
Label_Root.getChildren().add(Demo_Label);
// Set the stage
Scene Label_Scene = new Scene(Label_Root, 595, 150, Color.BEIGE);
Label_Stage.setTitle("Pane Example");
Label_Stage.setScene(Label_Scene);
Label_Stage.show();
}
public static void main(String args[]) {
launch(args);
}
}
子ノードをペインに追加できるため、このコードは正常に機能します。出力を参照してください:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook