C# で小数値を小数点以下 2 桁に丸める
-
decimal.Round()
メソッドを使用してDecimal
値を 2つのDecimal
桁に丸める C# プログラム -
Math.Round()
メソッドを使用して、Decimal
値を 2つのDecimal
桁に丸める C# プログラム
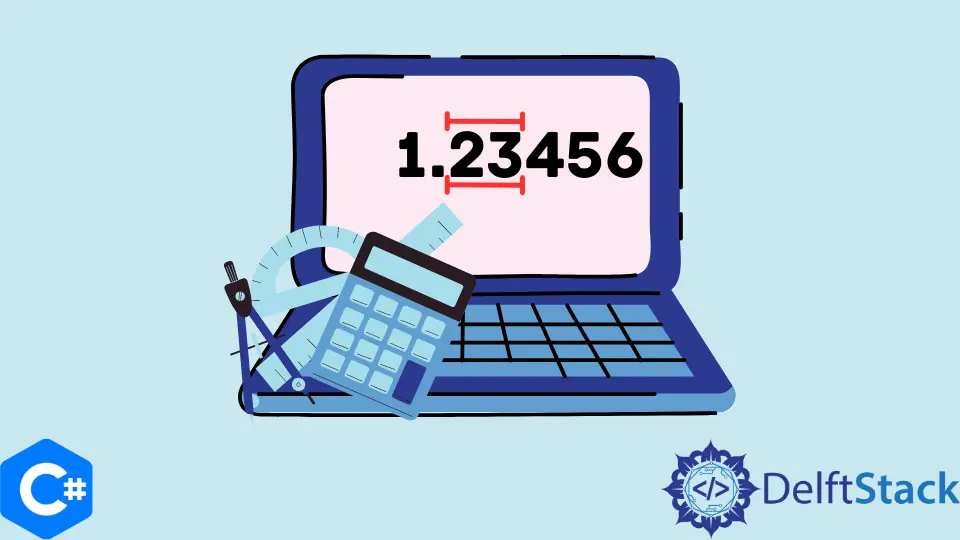
C# では、異なるメソッド、例えば、decimal.Round()
や Math.Round()
などを使用して、10 進数
を簡単に四捨五入することができます。
この記事では、浮動小数点以下 2 桁に丸める方法を中心に紹介します。
decimal.Round()
メソッドを使用して Decimal
値を 2つの Decimal
桁に丸める C# プログラム
メソッド decimal.Round()
は、decimal
数を指定された桁数に四捨五入するために使用される最も簡単なメソッドです。この方法では、最大 28 の小数桁を使用できます。
このメソッドを使用するための正しい構文は次のとおりです。
decimal.Round(decimalValueName, integerValue);
コード例:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 123.456M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = decimal.Round(decimalValue, 2);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
出力:
The Decimal Value Before Applying Method: 123.456
The Decimal Value After Applying Method: 123.46
このメソッドは、丸める小数の桁数を示す整数値が 0〜28 の範囲内にない場合に ArgumentOutOfRangeException
をスローします。この exception
は、try-catch
ブロックを使用して処理されます。
decimal.Round()
メソッドを使用する別の方法があります。サンプルコードを以下に示します:
コード例:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 12.345M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = decimal.Round(decimalValue, 2, MidpointRounding.AwayFromZero);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
出力:
The Decimal Value Before Applying Method: 12.345
The Decimal Value After Applying Method: 12.35
MidpointRounding.AwayFromZero
は、ゼロから離れるように数値を丸めるために使用されます。これに対応するものは MidpointRounding.ToEven
で、指定された 10 進数を最も近い偶数に丸めます。
コード例:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 12.345M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = decimal.Round(decimalValue, 2, MidpointRounding.ToEven);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
出力:
The Decimal Value Before Applying Method: 12.345
The Decimal Value After Applying Method: 12.34
Math.Round()
メソッドを使用して、Decimal
値を 2つの Decimal
桁に丸める C# プログラム
メソッド Math.Round()
は、機能的には decimal.Round()
メソッドと同じです。
このメソッドを使用するための正しい構文は次のとおりです。
Math.Round(decimalValueName, integerValue);
コード例:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 123.456M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = Math.Round(decimalValue, 2);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
出力:
The Decimal Value Before Applying Method: 123.456
The Decimal Value After Applying Method: 123.46
また、decimal.Round()
メソッドと同じように、exceptions
をスローします。このメソッドは、try-catch
ブロックを使用して処理されます。decimal.Round()
メソッドのように MidpointRounding
メソッドを指定することもできます。