C#은 부동 소수점 숫자를 소수점 이하 두 자리로 반올림합니다
-
decimal.Round()
메소드를 사용하여Decimal
값을 2 개의Decimal
위치로 반올림하는 C# 프로그램 -
Math.Round()
메소드를 사용하여Decimal
값을 2 개의Decimal
위치로 반올림하는 C# 프로그램
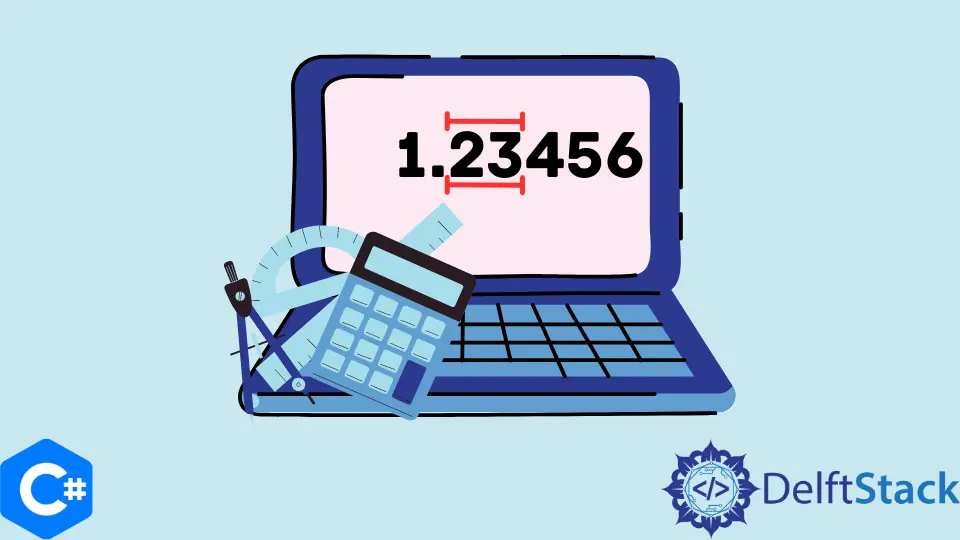
C#에서, 우리는 다른 방법을 사용하여 하나의 부점 수, 예를 들어 decimal.Round()
와 Math.Round()
를 쉽게 버릴 수 있다.
이 기사에서는 부동 값을 소수점 이하 2 자리로 반올림하는 방법에 중점을 둘 것입니다.
decimal.Round()
메소드를 사용하여Decimal
값을 2 개의Decimal
위치로 반올림하는 C# 프로그램
decimal.Round()
메소드는 ‘소수’숫자를 지정된 자릿수로 반올림하는 데 사용되는 가장 간단한 메소드입니다. 이 방법은 최대 28 개의 ‘소수점’을 허용합니다.
이 방법을 사용하는 올바른 구문은 다음과 같습니다.
decimal.Round(decimalValueName, integerValue);
예제 코드:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 123.456M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = decimal.Round(decimalValue, 2);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
출력:
The Decimal Value Before Applying Method: 123.456
The Decimal Value After Applying Method: 123.46
반올림 할 소수점 이하 자릿수를 알려주는 정수 값이 0-28 범위에 있지 않으면이 메소드는 ArgumentOutOfRangeException
을 던집니다. 이 ‘예외’는 try-catch
블록을 사용하여 처리됩니다.
decimal.Round()
메소드를 사용하는 또 다른 방법이 있습니다. 예제 코드는 다음과 같습니다.
예제 코드:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 12.345M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = decimal.Round(decimalValue, 2, MidpointRounding.AwayFromZero);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
출력:
The Decimal Value Before Applying Method: 12.345
The Decimal Value After Applying Method: 12.35
MidpointRounding.AwayFromZero
는 숫자를 0에서 멀어지게하는 데 사용됩니다. 대응하는 것은MidpointRounding.ToEven
이며, 주어진 십진수를 가장 가까운 짝수로 반올림합니다.
예제 코드:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 12.345M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = decimal.Round(decimalValue, 2, MidpointRounding.ToEven);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
출력:
The Decimal Value Before Applying Method: 12.345
The Decimal Value After Applying Method: 12.34
Math.Round()
메소드를 사용하여Decimal
값을 2 개의Decimal
위치로 반올림하는 C# 프로그램
Math.Round()
메소드는 기능면에서decimal.Round()
메소드와 동일합니다.
이 방법을 사용하는 올바른 구문은 다음과 같습니다.
Math.Round(decimalValueName, integerValue);
예제 코드:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 123.456M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = Math.Round(decimalValue, 2);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
출력:
The Decimal Value Before Applying Method: 123.456
The Decimal Value After Applying Method: 123.46
decimal.Round()
방법처럼 그것도 이상을 던지고 try-catch
블록을 사용하여 처리한다. decimal.Round()
메소드에서와 마찬가지로MidpointRounding
메소드를 지정할 수도 있습니다.